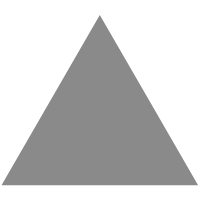
7
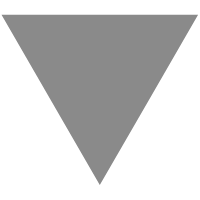
GitHub - dpouris/gottp-server: Gottp is an HTTP server that can be used to serve...
source link: https://github.com/dpouris/gottp-server
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
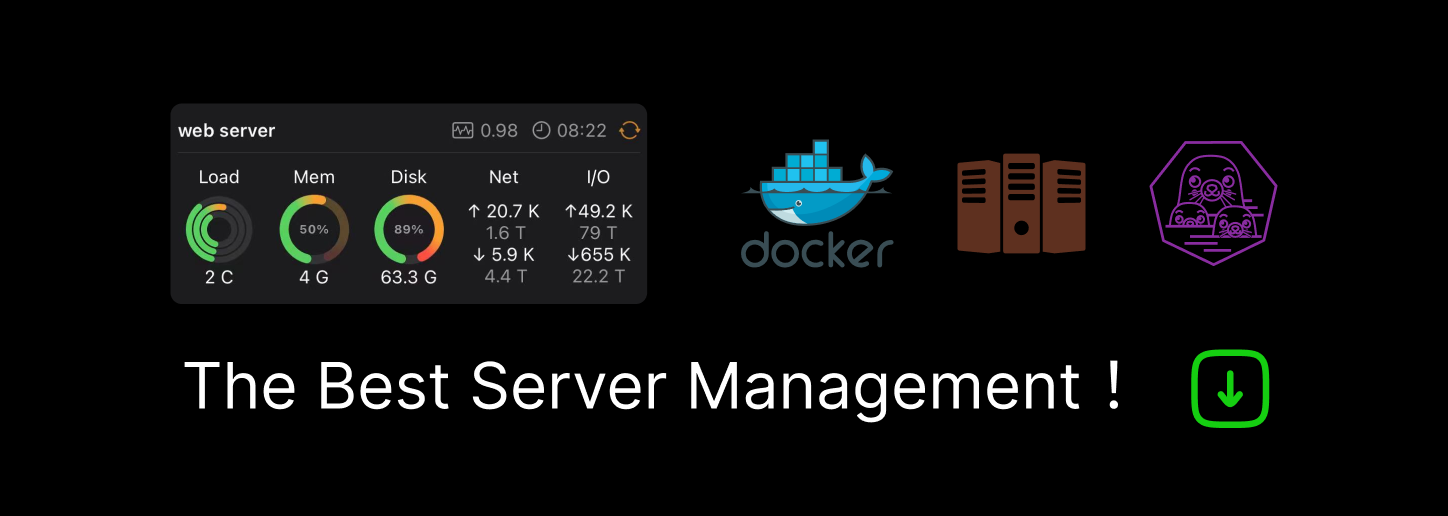
GOTTP SERVER
Gottp is an HTTP server that can be used to serve static files and make simple API routes. It provides an abstraction on top of the built in http package to get up and running in no time.
INSTALLATION
$ go get -u github.com/dpouris/gottp-server
EXAMPLE
package main
import (
"encoding/json"
"fmt"
"io/ioutil"
"net/http"
Gottp "github.com/dpouris/gottp-server"
)
func main() {
g := Gottp.Server()
g.Get("/hey", func(r Gottp.Res, req *Gottp.Req) error {
// Loads the HTML file
heyPage, err := ioutil.ReadFile("./examples/hey.html")
if err != nil {
Gottp.LogError(err.Error(), g.Logger)
}
// Write the HTML to the response body
r.Write(heyPage)
return nil
})
g.Post("/hey", func(r Gottp.Res, req *Gottp.Req) error {
// A map that is marshalled to JSON
log_map := map[string]string{
"hey": "you",
"hello": "world",
}
// Write the JSON to the resonse body
err := r.JSON(log_map)
if err != nil {
Gottp.LogError(err.Error(), g.Logger)
}
return nil
})
// Listen on port 8088
g.ListenAndServe(":8088")
}
USAGE
New Server
g := Gottp.Server()
GET
g.Get("/path", func(r Gottp.Res, req *Gottp.Req) error {
// Handler logic
})
POST
g.Post("/path", func(r Gottp.Res, req *Gottp.Req) error {
// Handler logic
})
ListenAndServe
g.ListenAndServe(":8000") //Pass in whatever port is free
Global Middleware
g.AddGlobalMiddleware(func(r http.ResponseWriter, req *http.Request) error {
// middleware logic
})
LOGGING
By default Gottp handles all incoming requests and Logs the info on the Logs field. On the example bellow I create a new instance of Gottp server and supply Gottp.Logger
to the Log functions.
import Gottp "github.com/dpouris/gottp-server"
func main() {
g := Gottp.Server()
// Logs to stdout
Gottp.LogInfo("This is an info message", g.Logger)
Gottp.LogWarning("This is an warning message", g.Logger)
Gottp.LogError("This is an error message", g.Logger)
}
// OUTPUT
2022/06/07 11:45:40 INFO - This is an info message
2022/06/07 11:45:40 WARN - This is an warning message
2022/06/07 11:45:40 ERROR - This is an error message
All logs
You can access all the logs on the Gottp.Logs
field.
g.Get("/logs", func(r Gottp.Res, req *Gottp.Req) error {
log_map := make(map[int]any, len(g.Logs))
for i, v := range g.Logs {
log_map[i] = v
}
err := r.JSON(log_map)
if err != nil {
Gottp.LogError(err.Error(), g.Logger)
}
return nil
})
-
Sample Response
{ "0": "[GET] ON ROUTE /hey", "1": "[GET] ON ROUTE /logs" } // Logs are stored in the Logs field of Gottp instance
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK