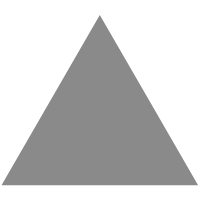
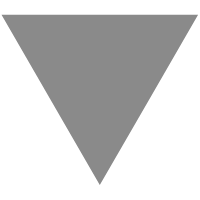
GitHub - leongrdic/php-smplang: A simple language written in PHP that evaluates...
source link: https://github.com/leongrdic/php-smplang
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
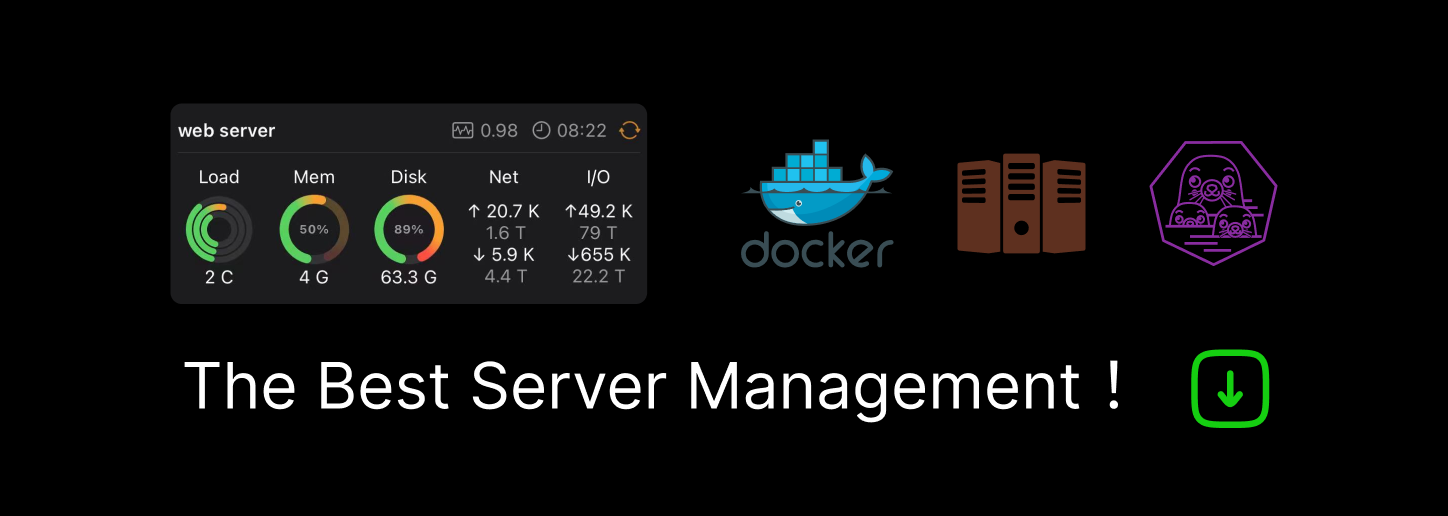
SMPLang
SMPLang is a simple expression language for PHP. It's currently in a pre-release state and any contributions and tests are welcome.
The language is partly inspired by Symfony Expression Language but there are some major differences. For more details and a performance benchmark, see test.php.
Install:
composer require leongrdic/smplang:dev-master
To use SMPLang, create a new instance of the SMPLang class and pass in an array of variables. These variables will be available to use in your expressions.
$smpl = new Le\SMPLang\SMPLang([
'variableName' => 'value',
];
You can then call the evaluate()
method on your SMPLang instance, passing in an expression string. This will return the result of the expression.
$smpl->evaluate($expression);
In case of an exception, Le\SMPLang\Exception
will be thrown with short description of the error.
Syntax
Variables are accessed only by their name. If a variable is not defined, an exception will be thrown.
Array elements are accessed with the following syntax: array.key.0
(even numeric keys).
Object properties and methods are accessed with the following syntax: object.property
or object.method(parameters)
.
Closures/functions are called with the following syntax: closure(parameter1, parameter2, ...)
Arrays are defined with the following syntax: [element1, element2, ...]
Array unpacking (...array
) is supported in array definitions and closure calls.
Supported literals
null
- booleans (
true
andfalse
) - strings (
"string"
or'string'
) - numbers (
1
,1.2
,-1
,-1.2
) - arrays (
[23, 'string']
) - hashes aren't supported currently
Arithmetic operators
+
: addition-
: subtraction*
: multiplication/
: division%
: modulo**
: exponentiation
Comparison operators
===
: strict equality!==
: strict inequality==
: equality!=
: inequality>
: greater than<
: less than>=
: greater than or equal to<=
: less than or equal to
Logical operators
&&
: logical and||
: logical or!
: logical not
String concatenation
~
: string concatenation
Ternary expressions
a ? b : c
a ?: b
(is equivalent toa ? a : b
)a ? b
(is equivalent toa ? b : null
)
Examples
$smpl = new Le\SMPLang\SMPLang();
$result = $smpl->evaluate('(1 + 2 * 3) / 7');
// $result will be 1
$smpl = new Le\SMPLang\SMPLang([
'foo' => 'bar',
'arr' => [1, 2, 3],
'hash' => ['a' => 'b'],
]);
$result = $smpl->evaluate('foo ~ " " ~ arr.1 ~ " " ~ hash.a');
// $result will be "bar 2 b"
$smpl = new Le\SMPLang\SMPLang([
'prepend' => fn(string $a): string => "hello $a",
'reverse' => strrev(...),
]);
$result = $smpl->evaluate('prepend("simple " ~ reverse("world"))');
// $result will be "hello simple dlrow"
$smpl = new Le\SMPLang\SMPLang([
'foo' => 'bar',
]);
$result = $smpl->evaluate('foo !== "bar" ? "yes" : "no"');
// $result will be "no"
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK