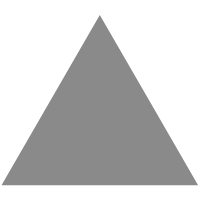
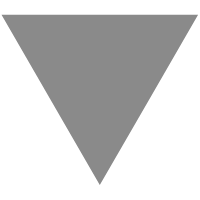
PHP的类型约束
source link: https://panda843.github.io/article/1678094766.html
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
PHP的类型约束
众所周知,在 强类型 语言中,类型约束 是语法上的要求,即:定义一个变量的时候,必须指定其类型,并且以后该变量也只能存储该类型数据。
而我们的PHP是弱类型语言,其特点就是无需为变量指定类型,而且在其后也可以存储任何类型,当然这也是使用PHP能快速开发的关键点之一。但是在php的高版本语法中(PHP5起),在某些特定场合,针对某些特定类型,也是可以进行语法约束的。
自PHP5起,我们就可以在函数(方法)形参中使用类型约束了。
函数的参数可以指定的范围如下:
- 必须为对象(在函数原型里面指定类的名字);
- 数组(PHP 5.1 起);
- callable(PHP 5.4 起)。
如果使用 NULL 作为参数的默认值,那么在调用函数的时候依然可以使用 NULL 作为实参。
如果一个类或接口指定了类型约束,则其所有的子类或实现也都如此。
类型约束不能用于标量类型如 int 或 string。Traits 也不允许。
<?php |
函数调用的参数与定义的参数类型不一致时,会抛出一个可捕获的致命错误。
<?php |
类型约束不只是用在类的成员函数里,也能使用在函数里
<?php |
类型约束允许 NULL 值
<?php |
以上就是PHP类型约束的大概简介和使用方法了,在使用PHP进行开发过程中,用到它的地方可能不是太多,我们最常看见或用到类型约束的地方是在“依赖注入”的设计模式中
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK