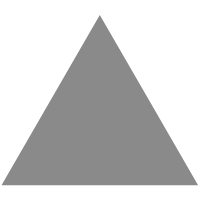
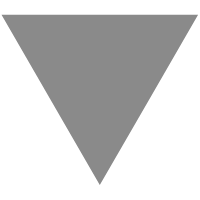
PHP的适配器模式实现
source link: https://panda843.github.io/article/247704845.html
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
PHP的适配器模式实现
适配器模式在不修改现有代码的基础上,保留了架构。使用继承的适配器和使用组件的适配器各有利弊,继承的类冗余度/空间复杂度偏高,组件的调用栈/时间复杂度偏高,应该结合实际情况选择。
简单来说,当你的实现和需要的接口,都无法修改的时候。
例如,你需要给甲方已有的系统做标准的兼容,标准不可修改,甲方的系统也不可修改,这个时候你就需要适配器的设计模式了。
对于web编程来说,将你现有的实现,和三方库结合起来,就需要使用适配器模式。
适配器模式是一种利用适配器将现有的实现,适配到已有接口的设计模式,最常见的例子就是变压器,将已有的5V输入的电器,通过变压器,适配到220V的电源插座。
相比继承,组件可用性高,低耦合,冗余度低,因此推荐采用组件的模式来进行设计。
继承方式实现
<?php |
组件方式实现
<?php |
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK