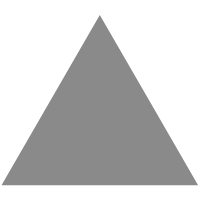
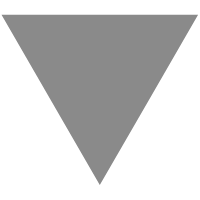
[Golang] GopherJS DOM Example - Dropdown Menu
source link: http://siongui.github.io/2016/01/16/gopherjs-dom-example-dropdown-menu/
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
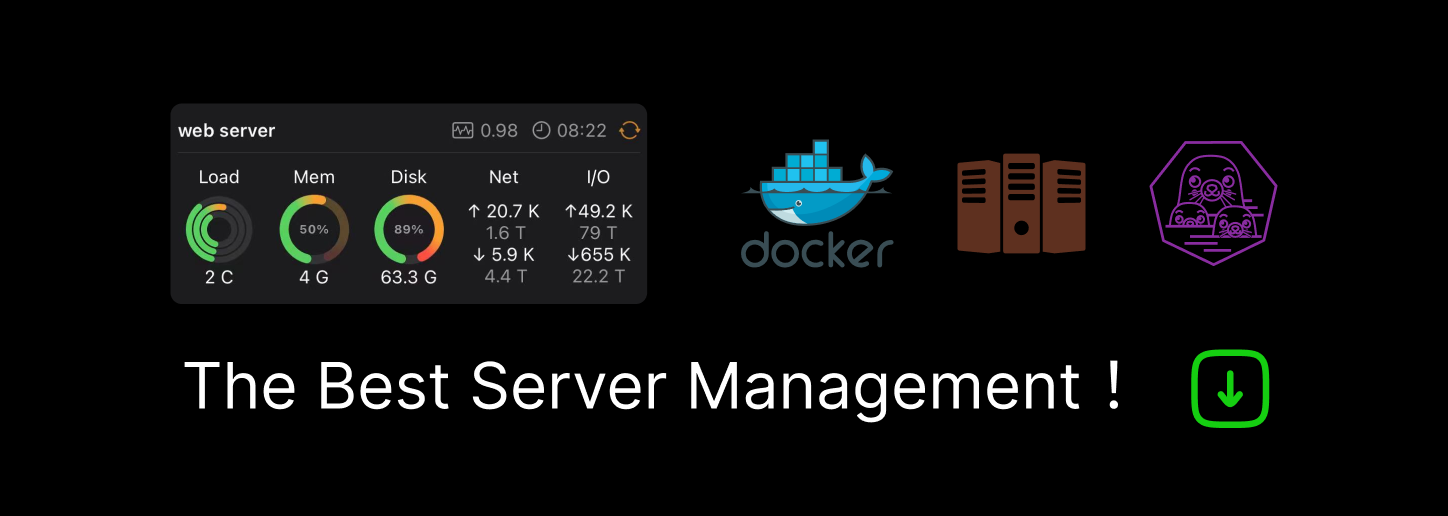
Source Code
First we write a simple HTML and CSS for our demo:
index.html | repository | view raw
<!doctype html> <html> <head> <title>Golang Dropdown Menu by GopherJS</title> <link rel="stylesheet" type="text/css" href="style.css"> </head> <body> <div id="menu-dropdown">Menuā¼</div> <div id="menuDiv-dropdown" class="invisible"> line1<br>line2<br>line3 </div> <script src="demo.js"></script> </body> </html>
style.css | repository | view raw
#menu-dropdown { font-size: 3em; color: blue; } #menu-dropdown:hover { cursor: pointer; } #menuDiv-dropdown { font-size: 3em; position: absolute; border: 1px solid blue; } .invisible { display: none; }
We will bind a onclick event handler to the HTML DOM document object. When users click inside the browser, we will check which DOM element is clicked. According to the clicked element and visibility of the dropdown menu, the dropdown menu will show up or disappear.
dropdown.go | repository | view raw
package main import "honnef.co/go/js/dom" func checkParent(target, elm dom.Element) bool { for target.ParentElement() != nil { if target.IsEqualNode(elm) { return true } target = target.ParentElement() } return false } func main() { d := dom.GetWindow().Document() toggle := d.GetElementByID("menu-dropdown").(*dom.HTMLDivElement) menu := d.GetElementByID("menuDiv-dropdown").(*dom.HTMLDivElement) d.AddEventListener("click", false, func(event dom.Event) { if !checkParent(event.Target(), menu) { // click NOT on the menu if checkParent(event.Target(), toggle) { // click on the link menu.Class().Toggle("invisible") } else { // click both outside link and outside menu, hide menu menu.Class().Add("invisible") } } }) }
Compile the Go code to JavaScript by:
$ gopherjs build dropdown.go -o demo.js
Put demo.js together with the index.html and style.css in the same directory. Open the index.html with your browser. Click on the Menu text to toggle the visibility of the menu. Also try to click outside the dropdown menu, the dropdown menu will disappear.
Tested on: Ubuntu Linux 15.10, Go 1.5.3.
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK