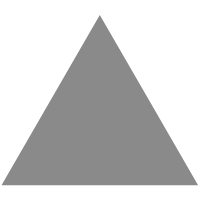
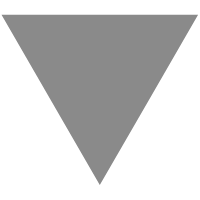
[Dart] Draggable (Movable) Element
source link: http://siongui.github.io/2015/02/17/dart-draggable-movable-element/
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
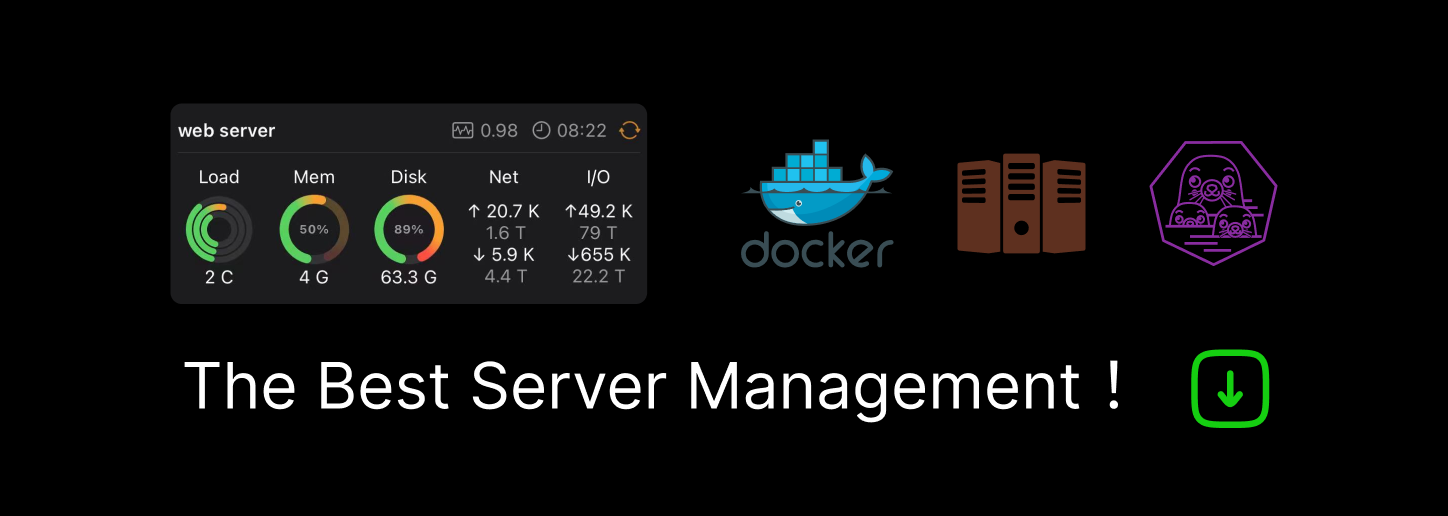
[Dart] Draggable (Movable) Element
February 17, 2015
This post is Dart version of my previous posts [1] and [2].
If you need draggable element using pure (vanilla) JavaScript, see [1].
If you need draggable element in AngularJS way, see [2].
Dartium is needed for the demo.
Development Environment: Ubuntu Linux 14.10, Dart 1.8.
Source Code for Demo (HTML):
dart-draggable-element.html | repository | view raw
<!doctype html> <html> <head> <meta charset="utf-8"> <title>Dart Draggable (div) Element</title> </head> <body> <div id="dragMe" style="width: 200px; height: 200px; background: yellow;">Drag Me</div> <script type='text/javascript'> var script = document.createElement('script'); if (navigator.userAgent.indexOf('(Dart)') === -1) { // Browser doesn't support Dart script.setAttribute("type","text/javascript"); script.setAttribute("src", "app.js"); } else { script.setAttribute("type","application/dart"); script.setAttribute("src", "app.dart"); } document.getElementsByTagName("head")[0].appendChild(script); </script> </body> </html>
Source Code for Demo (Dart):
app.dart | repository | view raw
// https://api.dartlang.org/apidocs/channels/stable/dartdoc-viewer/dart:html.Element // https://api.dartlang.org/apidocs/channels/stable/dartdoc-viewer/dart:html.MouseEvent // https://www.dartlang.org/articles/improving-the-dom/ // http://stackoverflow.com/questions/14476738/remove-event-listener-with-the-new-library // https://code.google.com/p/dart/issues/detail?id=15216 // https://github.com/threeDart/three.dart/issues/109 import 'dart:html'; void draggable(Element elm) { int startX, startY, initialMouseX, initialMouseY; var docMouseMoveSub, docMouseUpSub; elm.style.position = "absolute"; void mousemove(e) { e.preventDefault(); // e.clientX works in Dartium, but fails on compiled js int dx = e.client.x - initialMouseX; // e.clientY works in Dartium, but fails on compiled js int dy = e.client.y - initialMouseY; elm.style.top = (startY+dy).toString() + 'px'; elm.style.left = (startX+dx).toString() + 'px'; } void mouseup(e) { docMouseMoveSub.cancel(); docMouseUpSub.cancel(); } elm.onMouseDown.listen((e) { e.preventDefault(); startX = elm.offsetLeft; startY = elm.offsetTop; // e.clientX works in Dartium, but fails on compiled js initialMouseX = e.client.x; // e.clientY works in Dartium, but fails on compiled js initialMouseY = e.client.y; docMouseMoveSub = document.onMouseMove.listen(mousemove); docMouseUpSub = document.onMouseUp.listen(mouseup); }); } void main() { draggable(querySelector("#dragMe")); }
Makefile for automating the development:
Makefile | repository | view raw
# path of Dart and utilities DART_DIR=../../../../../dart DART_SDK=$(DART_DIR)/dart-sdk DART_SDK_BIN=$(DART_SDK)/bin DARTVM=$(DART_SDK_BIN)/dart DART2JS=$(DART_SDK_BIN)/dart2js DARTPUB=$(DART_SDK_BIN)/pub DARTIUM=$(DART_DIR)/chromium/chrome HTML=dart-draggable-element.html all: test # Fix Dartium startup error: # http://askubuntu.com/questions/369310/how-to-fix-missing-libudev-so-0-for-chrome-to-start-again test: DART_FLAGS='--checked' $(DARTIUM) --user-data-dir=/tmp/data $(HTML) & demo: js chromium-browser $(HTML) js: app.dart $(DART2JS) --minify --out=app.js app.dart # http://stackoverflow.com/questions/2989465/rm-rf-versus-rm-rf clean: -rm *.js -rm *.deps -rm *.map help: $(DARTVM) --print-flags
Dart MouseEvent clientX and clientY are deprecated. If you use clientX or clientY, the compiled JavaScript will not work. see [3] for detail.
References:
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK