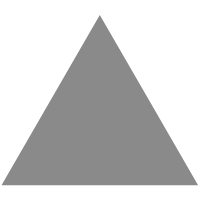
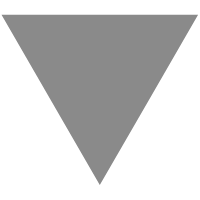
Get acquainted with powerful streams API and lambda expressions
source link: https://blog.knoldus.com/get-acquainted-with-powerful-streams-api-and-lambda-expressions/
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
Overview
The addition of the Stream was one of the major features added to Java 8. This in-depth tutorial is an introduction to the many functionalities supported by streams, with a focus on simple, practical examples.
To understand this material, you need to have a basic, working knowledge of Java 8 (lambda expressions, Optional, method references).
Introduction
First of all, Java 8 Streams should not be confused with Java I/O streams (ex: FileInputStream etc); these have very little to do with each other.
Simply put, streams are wrappers around a data source, allowing us to operate with that data source and making bulk processing convenient and fast.
A stream does not store data and, in that sense, is not a data structure. It also never modifies the underlying data source.
This functionality – java.util.stream – supports functional-style operations on streams of elements, such as map-reduce transformations on collections.
Let’s now dive into few simple examples of stream creation and usage – before getting into terminology and core concepts.
Java Stream Creation
Let’s first obtain a stream from an existing array:
private static Employee[] arrayOfEmps = { new Employee(1, "Jeff Bezos", 100000.0), new Employee(2, "Bill Gates", 200000.0), new Employee(3, "Mark Zuckerberg", 300000.0) }; Stream.of(arrayOfEmps);
We can also obtain a stream from an existing list:
private static List<Employee> empList = Arrays.asList(arrayOfEmps); empList.stream();
Note that Java 8 added a new stream() method to the Collection interface.
And we can create a stream from individual objects using Stream.of():
Stream.of(arrayOfEmps[0], arrayOfEmps[1], arrayOfEmps[2]);
Or simply using Stream.builder():
Stream.Builder<Employee> empStreamBuilder = Stream.builder(); empStreamBuilder.accept(arrayOfEmps[0]); empStreamBuilder.accept(arrayOfEmps[1]); empStreamBuilder.accept(arrayOfEmps[2]); Stream<Employee> empStream = empStreamBuilder.build();
There are also other ways to obtain a stream, some of which we will see in sections below.
Java Streams: What Are The Next Steps?
In this article, we focused on the details of the new Stream functionality in Java 8. We saw various operations supported and how lambdas and pipelines can be used to write concise code. We also saw some characteristics of streams like lazy evaluation, parallel and infinite streams. You’ll find the sources of the examples over on GitHub.
Now, what should you do next? Well, there’s a lot to explore in your journey to be a better Java developer, so here are a few suggestions.
For starters, you can continue your exploration of the concepts you’ve seen today with a look at the reactive paradigm, made possible by very similar concepts to the one we discussed here.
Additionally, keep in touch with the Stackify blog. We’re always publishing articles that might be of interest to you. You might need to learn more about the main Java frameworks, or how to properly handle exceptions in the language. In today’s article, we’ve covered an important feature that was introduced with Java 8. The language has come a long way since then and you might want to check out more recent developments.
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK