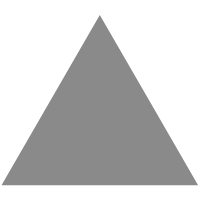
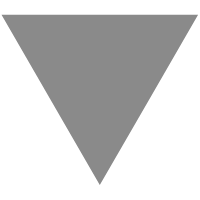
Firebase Authentication In Android - Ravi Rupareliya
source link: https://www.ravirupareliya.com/blog/firebase-authentication-in-android/?amp%3Butm_medium=rss&%3Butm_campaign=firebase-authentication-in-android
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
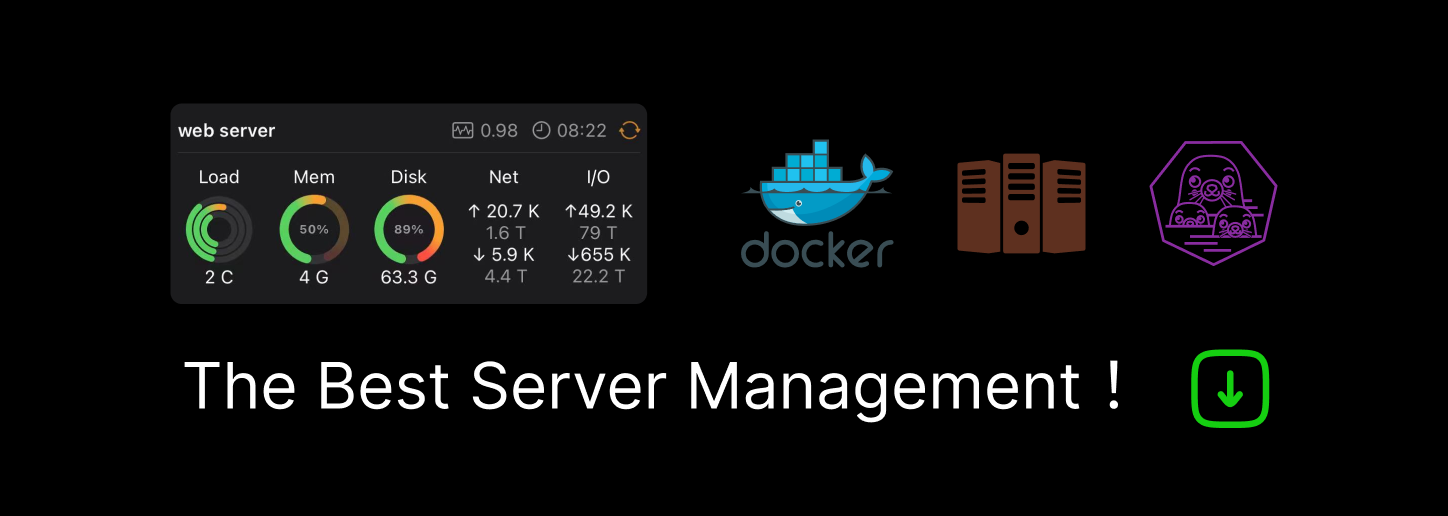
Firebase Authentication In Android
Authentication is the process to provide uniqueness to each user of your application. It will be the identity of particular user.
If your application requires user’s data to be securely stored, you need to authenticate each and every user.
In earlier days we need to have some back-end service developed either in PHP, Java or some other platform. We need some web developer to do that kind of things.
Firebase Authentication provides a way to directly authenticate a user via email and password and store that user to cloud.
Firebase Authentication also support social media authentication like G+, Facebook, Twitter, Github. It means now its a easy task to perform login with facebook or login with g+ task.
Types of Authentication Firebase supports :
- Email and password based authentication – Authentication with email and password
- Federated identity provider integration – Authentication with social media(G+, Facebook etc)
- Custom auth system integration
- Anonymous auth – Creates an anonymous account.
In today’s post we will see how basic authentication works.
Setup Firebase Authentication
Step 1 : Create a new project on Firebase Console. Enter you project name and select country, than click CREATE PROJECT
Step 2 : After creation of project click on Add Firebase to your Android app. Enter package name, App nickname(optional) and SHA-1 key(optional) and click on ADD APP.
Step 3 : You will receive google-services.json file, put it inside app/ folder of your project.
Step 4 : Add dependency to your root level build.gradle file.
Step 5 : Add dependency to your app/module level build.gradle file.
Step 6 : Add plugin at the bottom of your app-level build.gradle file.
Step 7 : That’s it, sync your project to apply all these effect of dependencies.
Initialize FirebaseAuth in your Activity or Application class.
Register a User
Login a User
You can get current user object either from Task or by using FirebaseAuth object
Change Password
Reset Password
Update Current User Profile
Logout User
Isn’t it looks so easy? Yes it is, have your own application with authentication and that also without having help of web developer or any heavy servers.
Ravi Rupareliya
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK