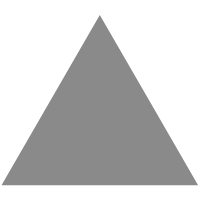
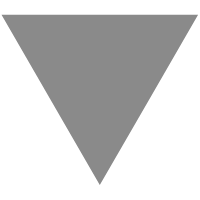
Learn How To Start OOPS IN SCALA
source link: https://blog.knoldus.com/learn-how-to-start-oops-in-scala/
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
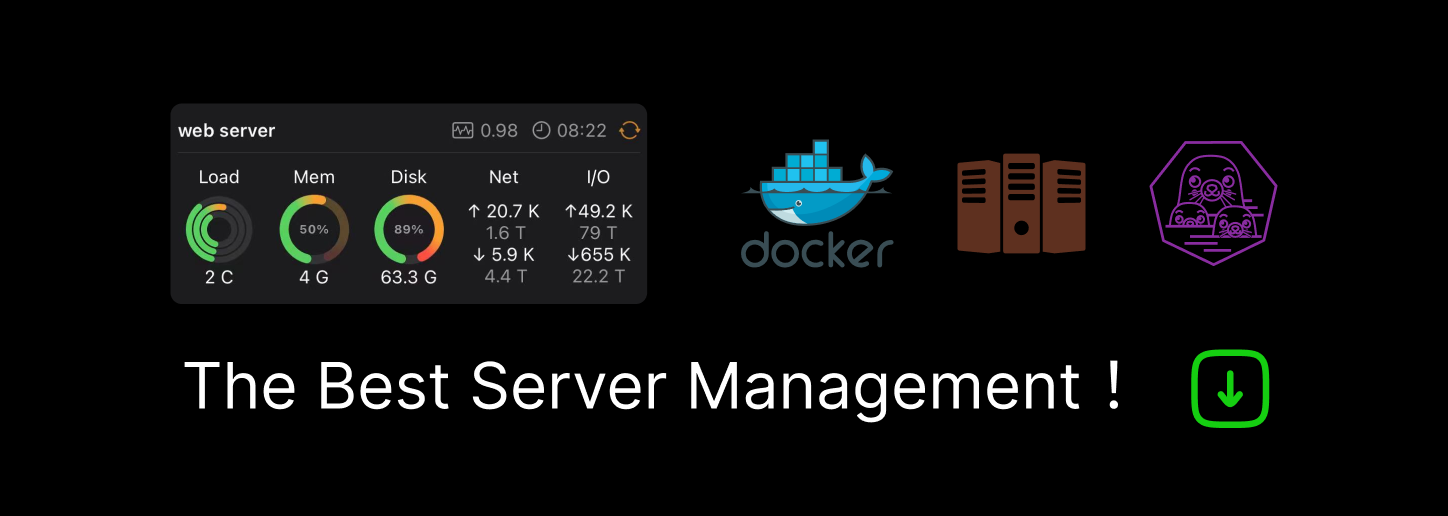
INTRODUCTION
Scala is a functional-object-oriented programming hybrid that seamlessly integrates the features of object-oriented and functional languages. It has the capability to interoperate with existing Java code and libraries.
The main aim of OOP is to bind together the data and the functions that operate on them so that no other part of the code can access this data except that function.
Why object-oriented?
Scala is a high-level programming language that mixes object-oriented and functional programming. Scala’s static types help prevent problems in complicated applications, and its JVM and JavaScript runtimes enable you to create high-performance systems with simple access to a vast library ecosystem.”
What are the 5 Pillars of OOP?
Classes and Object, Abstraction, Inheritance, and Polymorphism
OOPs concept used
Inheritance, hiding, polymorphism, and other real-world concepts are all part of object-oriented programming. It is the main objective of OOP to connect the data with the functions that operate on it so that no other part of the code is able to access the data except the functions that operate on it.
CLASSES AND OBJECTS
CLASS
A class is a user-defined blueprint or prototype from which objects are created. Essentially, a class is a collection of fields and methods (member functions that define actions).Basically, in a class constructor is used for initializing new objects, fields are variables that provide the state of the class and its objects, and methods are used to implement the behavior of the class and its objects.
SYNTAX:
class Class_name{}
class declarations can include these components, in order:
- Keyword class:A class keyword used to declare the type class.
- Class name:The name should begin with a initial letter (capitalized by convention).
- Superclass(if any):The name of the class’s parent (superclass), if any, preceded by the keyword extends. A class can only extend (subclass) one parent.
- Traits(if any): A comma-separated list of traits implemented by the class, if any, preceded by the keyword extends.
- Body: The class body surrounded by { } (curly braces).
EXAMPLE:
class Human{
var Name: String = "Rahul"
var Age: Int = 24
def Details()
{
println("Name of the Human : " + Name);
println("Age of the Human : " + Age);
}
}
object Main {
def main(args: Array[String]) {
var obj = new Human();
obj.Details();
}
}
OBJECT
It is a basic unit of Object-Oriented Programming and represents real-life entities. A typical Scala program creates many objects, which as you know, interact by invoking methods. In Scala, an object is a named instance with members such as fields and methods. An object consists of :
- State: It represented by attributes of an object. A property reflects the characteristics of an object as well.
- Behavior: It represented by methods of an object. It also reflects the response of an object with other objects.
- Identity: It gives a unique name to an object and enables one object to interact with other objects.
Initializing an object
The new operator instantiates a class by allocating memory for a new object and returning a reference to that memory.
EXAMPLE:
class School(name:String, section:String, Students:Int, Floor:String )
{
println("MY SCHOOL NAME IS:" + name + " My SCHOOL HAS :" + section+"SECTION");
println("MY SCHOOL HAS : " + Students +" STUDENTS" + " and MY SCHOOL HAS :" + Floor+"FLOOR");
}
object Main
{
def main(args: Array[String])
{
var obj = new School("SKR PUBLIC SCHOOL", "THREE ", 500, "FORTY");
}
}
CONSTRUCTORS
It is a constructor that initializes an object’s state. Like a method, a constructor consists of a set of statements (i.e. instructions) executed at the time of creation. Scala supports two types of constructors:
PRIMARY CONSTRUCTOR
AUXILARY CONSTRUCTOR
PRIMARY CONSTRUCTOR
The primary constructor and the class share a similar body, implying we need not make a constructor expressly.
SYNTAX:
class
class class_name(Parameter_list){
Example:
class Person(name: String, SirName: String, Age:Int)
{
def Data()
{
println("Name: " + name);
println("Sir Name: " + SirName)
println("Person AGE:" + Age)
}
}
object Main
{
def main(args: Array[String])
{
var obj = new Person("Rahul", "khowal",56)
obj.Data()
}
}
Auxiliary Constructor
In a Scala program, the constructors other than the primary constructor are known as auxiliary constructors. we are permitted to make quite a few auxiliary constructors in our program, yet a program contains just a single primary constructor.
Example:
class BioData( Name: String, SurName: String)
{
var Age: Int = 25
def Data()
{
println("Name: " + Name)
println("SurName: " + SurName)
println("Age: " + Age)
}
// Auxiliary Constructor
def this(Name: String, SurName: String, Age:Int)
{
// Invoking primary constructor
this(Name, SurName)
this.Age=Age
}
}
object Main
{
def main(args: Array[String])
{
var obj = new BioData("Gayatri", "Singh", 24)
obj.Data()
}
}
ABSTRACTION
Abstraction is the process to hide the internal details and showing only the functionality. It contains both abstract and non-abstract methods and cannot support multiple inheritances. A class can extend only one abstract class.
Syntax:
abstract class class_name { // code.. }
Example:
abstract class DATA
{
def details()
}
class DATA1 extends DATA
{
def details()
{
println("Name: Rahul")
println("Surname: Khowal")
}
}
object Main
{
def main(args: Array[String])
{
var obj = new DATA1()
obj.details()
}
}
POLYMORPHISM
Polymorphism means that a function type comes “in many forms”.
In programming, it means that
- the function can be applied to arguments of many types
- the type can have instances of many types.
We have seen two principal forms of polymorphism:
- subtyping: instances of a subclass can be passed to a base class
- generics: instances of a function or class are created by type parameterization
EXAMPLE:
class Calculation{
def function1(a:Int)
{
println("First Execution:" + a);
}
def function2(a:Int, b:Int)
{
var sum = a + b;
println("Second Execution:" + sum);
}
def function3(a:Int, b:Int, c:Int)
{
var product = a * b * c;
println("Third Execution:" + product);
}
}
object MAIN
{
def main(args: Array[String])
{
var ob = new Calculation();
ob.function1(120);
ob.function2(50, 70);
ob.function3(10, 5, 6);
}
}
INHERITANCE
Inheritance is an object-oriented concept that is used to reusability of code. You can achieve inheritance by using extends keyword. To achieve inheritance a class must extend to other classes. A class that is extended is called super or parent class. a class that extends class is called derived or base class.
SYNTAX:
class parent_class_name extends child_class_name{
Important Terminology
Reusable: in Reusability, once we wish to make a new class and there’s already a class that features a number of the code that we would like, we will derive our new class from the existing class we are reusing the fields and methods of the existing class.
Example:
class Parent{
var Name: String = "Rahul"
}
class Child extends Parent {
var Age: Int = 5
def details() {
println("Parent Name: " + Name)
println("Age: " + Age)
}
}
object Main {
def main(args: Array[String]) {
var obj = new Child();
obj.details();
}}
Conclusion
In this blog, we have gone through the oops concept in Scala. I hope that this was informative and easy to understand, also, if you have any queries please feel free to list them down in the comments section. If you enjoyed this article, share it with your friends and colleagues. For more blogs, Click Here
References
https://docs.scala-lang.org/
https://www.tutorialspoint.com/scala/index.htm
https://www.geeksforgeeks.org/scala-programming-language/?ref=ghme/?ref=ghm
YOU CAN ALSO CHECK MY OTHER BLOGS – CLICKHERE
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK