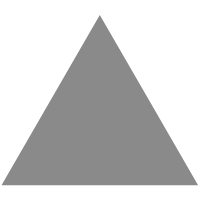
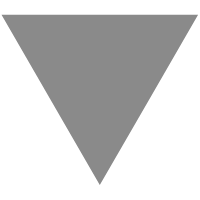
jQuery get Value of Selected Radio Button
source link: https://www.laravelcode.com/post/jquery-get-value-of-selected-radio-button
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
jQuery get Value of Selected Radio Button
jQuery radio button is used when you want to give user choice to select one from few options compared to select dropdown. In this article, I will share you how to get selected radio value. Radio is different input textbox or textarea. The value for radio is already defined, but we only need to get value for checked radio.
Here is how you can get value when user clicks any of radio button. jQuery's :checked selector only gets value from checked radio.
<!doctype html>
<html>
<head>
<link href="https://cdn.jsdelivr.net/npm/[email protected]/dist/css/bootstrap.min.css" rel="stylesheet">
</head>
<body>
<div class="container">
<h1 class="m-3">jQuery radio button value</h1>
<div class="form-check">
<input class="form-check-input" type="radio" name="gender" id="male" value="1">
<label class="form-check-label" for="male">Male</label>
</div>
<div class="form-check">
<input class="form-check-input" type="radio" name="gender" id="female" checked value="2">
<label class="form-check-label" for="female">Female</label>
</div>
</div>
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
<script type="text/javascript">
console.log($('input[name=gender]:checked').val());
</script>
</body>
</html>
If the radio button is not checked by default, it will return undefined value. So get value when change radio button. Here is the example.
<!doctype html>
<html>
<head>
<link href="https://cdn.jsdelivr.net/npm/[email protected]/dist/css/bootstrap.min.css" rel="stylesheet">
</head>
<body>
<div class="container">
<h1 class="m-3">jQuery radio button value</h1>
<div class="form-check">
<input class="form-check-input" type="radio" name="gender" id="male" value="1">
<label class="form-check-label" for="male">Male</label>
</div>
<div class="form-check">
<input class="form-check-input" type="radio" name="gender" id="female" value="2">
<label class="form-check-label" for="female">Female</label>
</div>
</div>
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
<script type="text/javascript">
$('input[name=gender]').change(function() {
console.log($(this).val());
});
</script>
</body>
</html>
Now if you want to check new radio button, then you need to use jQuery prop() method which uses to change element property value. You can see below exmaple:
<div class="form-check">
<input class="form-check-input" type="radio" name="gender" id="male" value="1">
<label class="form-check-label" for="male">Male</label>
</div>
<div class="form-check">
<input class="form-check-input" type="radio" name="gender" id="female" checked value="2">
<label class="form-check-label" for="female">Female</label>
</div>
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
<script type="text/javascript">
$('#male').prop('checked', true);
</script>
This way you can get value from selected radio button.
I hope you liked this article.

Hi, My name is Harsukh Makwana. i have been work with many programming language like php, python, javascript, node, react, anguler, etc.. since last 5 year. if you have any issue or want me hire then contact me on [email protected]
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK