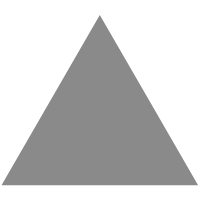
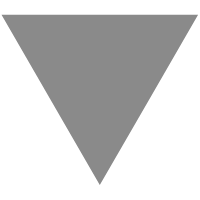
Send Verification Mail to New User in Anguler9
source link: https://www.laravelcode.com/post/send-verification-mail-to-new-user-in-anguler9
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
Send Verification Mail to New User in Anguler9
Hello folks, In this Angular 7/8/9 Firebase tutorial, I am going to answer commonly asked question How to send verification email to the new user? I am also going to show you How to prevent new users from accessing their account unless they validate their email address?
Firebase offers many powerful features for creating an authentication system. Sending email verification is not that difficult using Angular Firebase. I am going to show you how you can achieve this functionality very easily using Firebase API (AngularFire2 Library).
I will be using AngularFire2 library from the node package manager (NPM) and the latest Angular 7/8/9 release for this tutorial.
Create Auth Service
ng generate service auth
Import AuthService Class in app.module.ts
// Auth service
import { AuthService } from "./shared/services/auth.service";
providers: [
AuthService
]
Create the Auth Service for sending verification email using Firebase auth.service.ts
import { Injectable, NgZone } from '@angular/core';
import { AngularFireAuth } from "@angular/fire/auth";
import { Router } from "@angular/router";
@Injectable({
providedIn: 'root'
})
export class AuthService {
constructor(
public afAuth: AngularFireAuth, // Inject Firebase auth service
public router: Router, // Inject Route Service
public ngZone: NgZone // NgZone service to remove outside scope warning
) {
}
// Send email verfificaiton when new user sign up
SendVerificationMail() {
return this.afAuth.auth.currentUser.sendEmailVerification()
.then(() => {
this.router.navigate(['<!-- enter your route name here -->']);
})
}
// Sign up with email/password
SignUp(email, password) {
return this.afAuth.auth.createUserWithEmailAndPassword(email, password)
.then((result) => {
this.SendVerificationMail(); // Sending email verification notification, when new user registers
}).catch((error) => {
window.alert(error.message)
})
}
// Sign in with email/password
SignIn(email, password) {
return this.afAuth.auth.signInWithEmailAndPassword(email, password)
.then((result) => {
if (result.user.emailVerified !== true) {
this.SendVerificationMail();
window.alert('Please validate your email address. Kindly check your inbox.');
} else {
this.ngZone.run(() => {
this.router.navigate(['<!-- enter your route name here -->']);
});
}
this.SetUserData(result.user);
}).catch((error) => {
window.alert(error.message)
})
}
}
We have successfully created auth.service.ts
file and written pretty straightforward logic in it. There are 3 methods in our AuthService class.
- SendVerificationMail(): This method sends a verification email to newly created users using Firebase API with Angular.
- SignUp(email, password): This method allows users to create a new account and sends a verification email to a newly created user.
- SignIn(email, password): This Sign in method prevents the user to access if the email address is not verified.
You can also check out my detailed article on Full Firebase Authentication System with Angular 7.

Hi, My name is Harsukh Makwana. i have been work with many programming language like php, python, javascript, node, react, anguler, etc.. since last 5 year. if you have any issue or want me hire then contact me on [email protected]
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK