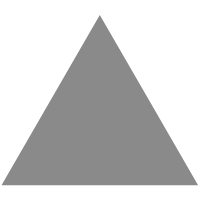
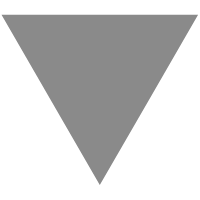
Getting to Know… React
source link: https://aarontgrogg.com/blog/2022/01/06/getting-to-know-react/
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
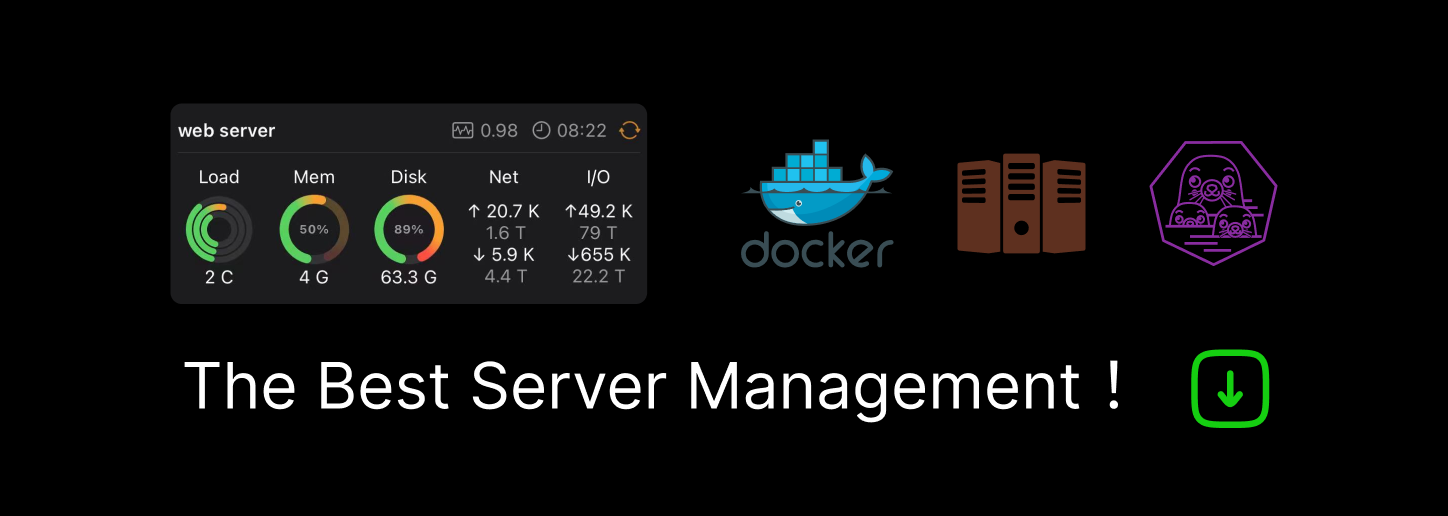
Getting to Know… React
- Share:
In this series, as I “get to know” some technology, I collect and share resources that I find helpful, as well as any tips, tricks and notes that I collected along the way.
My goal here is not to teach every little thing there is to learn, but to share useful stuff that I come across and hopefully offer some insight to anyone that is getting ready to do what I just finished doing.
So with that said, let’s get to know… React!
TL;DC, jump to the Notes, Tips & Tricks Section
I admit, I totally missed the boat on the whole MVC library/framework movement (I’m just going to call them all frameworks from here on). I come from a mindset that says “your site should work just fine without JS” and, at that time, all of those frameworks certainly flew in the face of that mindset; without JS, you usually get nothing but a blank page…
Well, my opinion was clearly not shared by the masses, and now some of the frameworks do offer SSR… So now I find myself a bit behind the times, but nevertheless ready to dig in.
Okay, so I didn’t totally miss the boat… I actually did write a 6-part series comparing what, at the time, were what I considered to be the “big four”: AngularJS, Ember.js, React and Backbone.js.
Read one, or collect them all!
But before I could dig in, I had to make a monstrous decision: Which one??? Angular was certainly the first powerhouse and still is, it seems, with enterprises, but it certainly does not control the job market anymore. React is easily the biggest player right now, with jobs and jobs galore. Backbone and Ember are both still very viable options, though neither is very strong in the job market. And recently there have been some very interesting new kids on the block that have a slightly different take on things, like Vue, Svelte, Qwik, and, well, the list goes on and on and on…
But, figuring most people are probably learning a new tech to help find a new job or improve their standings at their current one, I went with the current king of the job boards, and that is definitively React…
So, let’s get to know React!
Getting Started
As with all new things, the first stab at learning is a massive Google search… And boy are there a lot of tutorials! Articles, videos, articles with videos, documentation…
Of course, the first place we should all start is the documentation. But man can that stuff be dry… And when I was starting my search, React’s documentation was all words and code samples, no videos, no interaction… And I definitely learn best by tinkering!
React’s new documentation, in beta at the time of writing, though incomplete, looks fantastic! All code samples are interactive demos, letting you tinker right there, no installs required, and even handy “reset” buttons so you can break things to your heart’s content and easily get back to the start again! Bravo, React Docs Team!So I went looking for articles & videos. There are naturally a lot of “Learn React in 10 minutes!” types of articles/videos out there, and while they do at least expose you to some bits and pieces, you are never going to actually learn something in 10 minutes… Or 15, or 20…
One article that I will recommend is Tania Rascia‘s Getting Started with React. For me, her writing style is just very easy to read and ingest. It was the first React article that I read, because I was familiar with her and saw she had one. I would recommend this as a first “toe in the water” tutorial.So then I went looking for courses. Again, there are plenty! And I tried plenty!! But with each one, I found myself walking away… Either the code was really old (tech changes fast!), I had trouble understanding or following the presenter, or they just bounced around too much…
Note to all Presenters: Please, when you are live coding something, STOP clicking-dragging-highlighting-scrolling all over the damned place! You are making your audience nauseous! Thank you.But finally, I found my way to Scrimba and found a course description that sounded perfect. But it was not free, which is fine with me, I definitely do not mind paying for someone’s time, but I was now quite gun-shy, and was afraid of paying for a 12-hour, 126-lesson course, only to find the presenter drove me nuts!
So I reached out to Scrimba support and asked if they had a sample video of the presenter. To my surprise, they did not… But they did refer me to a free intro course (you do have to create an account and login), on the same subject, by the same instructor! :-)
I immediately took that intro course and loved it and the presenter so much that I subscribed and took the full course! They are both awesome, and Bob Ziroll, for me, is an excellent instructor: He speaks slowly and calmly; he presents subjects methodically, some times deviating to a seemingly unrelated subject or example, only to bring it back around and incorporate that subject or example in what he was previously teaching; he breaks up the lessons with lots of “hands on the keyboard” time; and the Scrimba interface itself is fantastic, allowing you to sort of interact with what appears to be the video, editing and testing code right in the browser, then returning seamlessly to the lesson…
Note: I am not in any way affiliated with, nor sponsored by Scrimba. I just like their stuff.Between Tania’s initial article, a host of other articles and videos, and these two courses, I have collected the following, which will likely be updated as I continue to learn more… Have fun!
Notes, Tips & Tricks
-
Glossary
Component
Controlled Components
Hooks
JSX
Lifecycle
Lifecycle Methods
Lifting State
Mount
Props
State
Unmount
-
Primer
-
Add React to an existing page/site, using ES6
{ (props) { (props) .state = { liked: } } render() { (.state.liked) { } React.createElement( , { onClick: () => .setState({ liked: }) }, ) } } container = .querySelector() ReactDOM.render(React.createElement(LikeButton), container);
-
Add React to an existing page/site, using JSX
{ render() {
-
Create Complete React App
cd react-app-demo && npm start
/react-app-demo /node_modules /public /src .package.json
-
Add Dependencies to an Existing React Project
-
JSX
-
“Declarative” vs. “Imperative”
elem = .createElement() elem.className = elem.innerHTML = .querySelector().append(elem)
$().append()
ReactDOM.render( React.createElement( , {
ReactDOM.render(
elem =
-
render()
1 Line vs. Multiple Lines{ render() {
{ render() { ( } }
{ render() { ( } }
-
Various Ways to
import
React and ComponentsReact ReactDOM
MyComponent
{Apple, Banana, Orange}
React { ... }
React, {Component} { ... }
-
Components
FunctionalComponent = () => {
{ render() {
{
{
{
Basic Component construct: Using Props:
Using State:
Lifecycle Methods:
-
Props
{ { id, firstName, lastName, jobTitle } = props ... }
employees = [ { id: , firstName: , lastName: , jobTitle: , }, { id: , firstName: , lastName: , jobTitle: , }, { id: , firstName: , lastName: , jobTitle: , }, { id: , firstName: , lastName: , jobTitle: , }, ];
{ ( }
{ rows = props.data.map( employee => { ( }) ( {rows} ) }
{ ( }
{ ( }
<Component prop1= isCool={} team={[ {name: , title: }, {name: , title: }, {name: , title: }, {name: , title: } ]} onClick={myOnClickFunction} />
{ rows = props.allEmployees.map( (row, index) => { ( }) ( }
{ (
-
State
React { [count, setCount] = React.useState(); ... }
React, {useState} { [count, setCount] = useState(); ... }
[count, setCount] = useState();
setCount()
setCount(prevCount => prevCount + )
setCount(prevCount => prevCount++)
setTasks(tasks.push()) setTasks(prevTasks => prevTasks.push())
setTasks(prevTasks => [...prevTasks, ])
setContact(prevContact => { {...prevContact, phoneNumber: newPhoneNumber} })
setContact(prevContact => ({...prevContact, phoneNumber: newPhoneNumber}))
{ (props) { (props); .state = { count: }; } ... }
.setState({ state: (.state + ) })
{ (props) { (props) .state = props.data } removeItem = ( index ) => { { items } = .state .setState({ items: items.filter((item, i) => { i !== index }) }) } render() { ( } } Table = props => { { items, removeItem } = props ( } TableBody = props => { rows = props.items.map( (row, index) => { ( }) ( }
Table = props => { ( }
-
Lifting/Raising/Derived State
-
Event Listeners
Click me
{ setState(prev => { prev.map(user => { user.id === id ? {...user, active: !user.active} : user }) }) }
/** DO NOT DO THIS **/ Click me
{toggle(id)}}>Click me
-
Inline CSS
Hello World!
Hello World!
styles = {width: px}
Hello World!
styles = { backgroundColor: , fontColor: }
Hello World!
-
Adding Assets (images, SVGs, videos, etc.)
/my-react-app /node_modules /public /src .package.json
{ ( }
logo ; { ( }
-
Conditional Rendering
Hello { firstName !== '' ? firstName : 'World' }!
{ props.shouldShow &&
-
Forms
[firstName, setFirstName] = React.useState() { setFirstName(event.target.value) }
[formData, setFormData] = React.useState({ firstName: , lastName: }) { setFormData(prevFormData => { { ...prevFormData, [event.target.name]: event.target.value } }) }
-- Choose -- Red Blue ...
The comment is here
{ {name, value, type, checkbox} = event.target setFormData(prevFormData => { { ...prevFormData, [name]: (type === ? checked : value) } }) }
Submit
{ event.preventDefault() saveDataSomewhere(formData) }
Employment
-
Side Effects
{ [count, setCount] = useState() useEffect(() => { .title = }) ( }
useEffect(() => { { setIsOnline(status.isOnline) } ChatAPI.subscribe(id, handleStatusChange) { ChatAPI.unsubscribe (id, handleStatusChange) } })
useEffect(() => { .title = ; }, [count]);
useEffect(() => { fetch() .then(res => res.json()) .then(data => setData(data)) })
useEffect(() => { fetch() .then(res => res.json()) .then(data => setData(data)) }, []);
-
Deploy to Live Web Server
Summary
I am super happy that I have finally dug into this MVC stuff! I really enjoyed learning what I have already learned, and definitely plan to keep learning more! I don't know if I will ever need it, as most things that I build are web "sites", not really web "apps", meaning, mostly just content, not a lot of interaction.
And while I know I could use React for a content-driven site, it also seems like the wrong tool for the job...
But I know that if I ever do need to do something "appy", I could crank out a React component or two and have a super fly add-on to any existing site!
Resources
Below is a very short list of resources that I found useful while getting to know React. I highly recommend you visit them all as well!
Thanks a lot for following along, and please, if you think I missed anything or if you differ with my interpretation or understanding of something, let me know! My goal here is for everyone to read this and get to know this topic better! And you can help with that!
Happy reacting,
Atg
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK