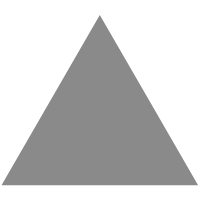
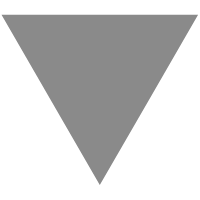
React JS : Components
source link: https://blog.knoldus.com/react-js-components/
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
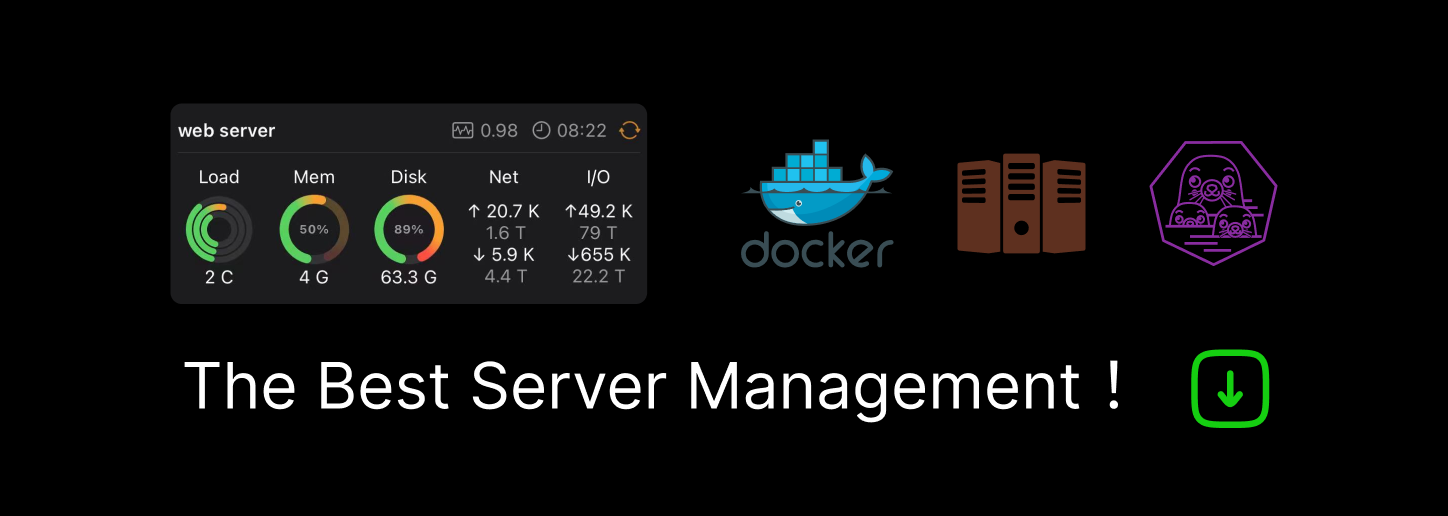
Reading Time: 6 minutes
React js is a JavaScript library for building user interfaces. Now we will study about components of react js.
Components
Components let you split the UI into independent, reusable pieces, and think about each piece in isolation. This page provides an introduction to the idea of components
Conceptually, components are like JavaScript functions. They accept arbitrary inputs (called “props”) and return React js elements describing what should appear on the screen
Types of React js Components
There are two types of main components in React js they are
- Stateful components
- Stateless components
Stateful Components
Any components are called stateful components, these state can be changed anywhere throughout the life cycle of components. With the name itself, we can understand it’s behavior.
In these components. Stateful components are class-based components. In class-based components, we will be creating one class where we will extend the core react library.
In this, Once we create a component with a particular class name we can use it by calling <component class name/> It looks very much like any other HTML tag.
Example:
import React from ‘react’;
import ReactDOM from ‘react-dom’;
class ClassComponents extends React.Component {
constructor() {
super()
this.state = {
msg: “Use me anywhere”
render() {
return (
<div>
<button>{this.state.msg}</button>
</div>
ReactDOM.render(
<ClassComponents />, document.getElementById(‘root’)
<div id=”root”></div>
Stateless components
All the attributes of these components will be fixed and not going to change throughout the life cycle of the component. With the name itself, we can understand it’s behavior. This is a Stateless component with a class where everything is static.
Example:
In the below example we are developing a react component having no state, it contains rules to apply a coupon.
Code:
import React from ‘react’;
import ReactDOM from ‘react-dom’;
class ClassComponents extends React.Component {
constructor() {
render() {
return (
<div>
<p>Condition to apply coupon:</p>
<ol>
<li>If you are ordering for the first time.</li>
<li>Minimum order amount>1000</li>
</ol>
</div>
ReactDOM.render(
<ClassComponents />, document.getElementById(‘root’)
<div id=”root”></div>
Lifecycle of Components
The three phases are: Mounting, Updating, and Unmounting.
Mounting
Mounting means putting elements into the DOM.
React has four built-in methods
- constructor()
- getDerivedStateFromProps()
- render()
- componentDidMount()
The render() method is required and will always be called, the others are optional and will be called if you define them.
constructor
The constructor() method is called first ,
The constructor() method is called with the props, and you should always start by calling the super(props) .
This will initiate the parent’s constructor method and allows the component to inherit methods from its parent (React.Component).
Example:
The constructor method is called, by React, every time you make a component:
class Header extends React.Component {
constructor(props) {
super(props);
this.state = {favoritecolor: “red”};
render() {
return (
<h1>My Favorite Color is {this.state.favoritecolor}</h1>
ReactDOM.render(<Header />, document.getElementById(‘root’));
getDerivedStateFromProps
The getDerivedStateFromProps() is called one step ahead of rendering the element in the DOM.
This is the natural place to set the state object based on the initial props.
It takes state as an argument, and returns an object with changes to the state.
The example starts with the favorite color being “red”, the getDerivedStateFromProps() method updates the favorite color based on the favcol attribute:
Example:
class Header extends React.Component {
constructor(props) {
super(props);
this.state = {favoritecolor: “red”};
static getDerivedStateFromProps(props, state) {
return {favoritecolor: props.favcol };
render() {
return (
<h1>My Favorite Color is {this.state.favoritecolor}</h1>
ReactDOM.render(<Header favcol=”yellow”/>, document.getElementById(‘root’));
render
The render() method is required, and is the method that actually outputs the HTML to the DOM.
Example:
A simple component with a simple render() method:
class Header extends React.Component {
render() {
return (
<h1>This is the content of the Header component</h1>
ReactDOM.render(<Header />, document.getElementById(‘root’));
Updating
React has five built-in methods
- getDerivedStateFromProps()
- shouldComponentUpdate()
- render()
- getSnapshotBeforeUpdate()
- componentDidUpdate()
The render() method is required and will always be called, the others are optional and will be called if you define them.
getDerivedStateFromProps
At updates the getDerivedStateFromProps() method is called.
This is the first method which is called after a component gets updated.
This is the natural place to set the state object based on the initial props.
The example has a button that changes the favorite color to blue, the getDerivedStateFromProps() method is called,
that updates the state with the color from the favcol attribute,
Example:
If the component gets updated, the getDerivedStateFromProps() method is called:
class Header extends React.Component {
constructor(props) {
super(props);
this.state = {favoritecolor: “red”};
static getDerivedStateFromProps(props, state) {
return {favoritecolor: props.favcol };
changeColor = () => {
this.setState({favoritecolor: “blue”});
render() {
return (
<div>
<h1>My Favorite Color is {this.state.favoritecolor}</h1>
<button type=”button” onClick={this.changeColor}>Change color</button>
</div>
ReactDOM.render(<Header favcol=”yellow”/>, document.getElementById(‘root’));
shouldComponentUpdate
shouldComponentUpdate() method you can return a Boolean value that specifies “React” should continue with the rendering or not.
The default value is true.
Example:
Stop the component from rendering at any update:
class Header extends React.Component {
constructor(props) {
super(props);
this.state = {favoritecolor: “red”};
shouldComponentUpdate() {
return false;
changeColor = () => {
this.setState({favoritecolor: “blue”});
render() {
return (
<div>
<h1>My Favorite Color is {this.state.favoritecolor}</h1>
<button type=”button” onClick={this.changeColor}>Change color</button>
</div>
ReactDOM.render(<Header />, document.getElementById(‘root’));
Example:
class Header extends React.Component {
constructor(props) {
super(props);
this.state = {favoritecolor: “red”};
shouldComponentUpdate() {
return true;
changeColor = () => {
this.setState({favoritecolor: “blue”});
render() {
return (
<div>
<h1>My Favorite Color is {this.state.favoritecolor}</h1>
<button type=”button” onClick={this.changeColor}>Change color</button>
</div>
ReactDOM.render(<Header />, document.getElementById(‘root’));
render
The render() method is called after a component gets updated, it has to re-render the HTML to the DOM.
The example below has a button that changes the favorite color to blue:
Example:
Click the button to make a change in the component’s state:
class Header extends React.Component {
constructor(props) {
super(props);
this.state = {favoritecolor: “red”};
changeColor = () => {
this.setState({favoritecolor: “blue”});
render() {
return (
<div>
<h1>My Favorite Color is {this.state.favoritecolor}</h1>
<button type=”button” onClick={this.changeColor}>Change color</button>
</div>
ReactDOM.render(<Header />, document.getElementById(‘root’));
getSnapshotBeforeUpdate
In the getSnapshotBeforeUpdate() method you have access to the props and state , meaning that even after the update, you can check what the values were the update.
The example below might seem complicated.
After the component is mounting it is rendered with the favorite color “red”. After the component has been mounted, a timer changes the state. And after one second, the favorite color becomes “yellow”.
That action triggers the update phase, and this component has a getSnapshotBeforeUpdate() method.
This method is executed, and writes a message to the empty DIV1 element.
After the componentDidUpdate() method is executed and writes a message in the empty DIV2 element.
Example:
class Header extends React.Component {
constructor(props) {
super(props);
this.state = {favoritecolor: “red”};
componentDidMount() {
setTimeout(() => {
this.setState({favoritecolor: “yellow”})
}, 1000)
getSnapshotBeforeUpdate(prevProps, prevState) {
document.getElementById(“div1”).innerHTML =
“Before the update, the favorite was ” + prevState.favoritecolor;
componentDidUpdate() {
document.getElementById(“div2”).innerHTML =
“The updated favorite is ” + this.state.favoritecolor;
render() {
return (
<div>
<h1>My Favorite Color is {this.state.favoritecolor}</h1>
<div id=”div1″></div>
<div id=”div2″></div>
</div>
ReactDOM.render(<Header />, document.getElementById(‘root’));
componentDidUpdate
The componentDidUpdate method is called after the component is updated in the DOM.
The component is mounting it is rendered with the favorite color “red”.
The component has been mounted, a timer changes the state, and the color becomes “yellow”.
This action triggers the update phase,and this component has a componentDidUpdate method,
this method is executed and writes a message in the empty DIV element:
Example:
The componentDidUpdate method is called after the update has been rendered in the DOM:
class Header extends React.Component {
constructor(props) {
super(props);
this.state = {favoritecolor: “red”};
componentDidMount() {
setTimeout(() => {
this.setState({favoritecolor: “yellow”})
}, 1000)
componentDidUpdate() {
document.getElementById(“mydiv”).innerHTML =
“The updated favorite is ” + this.state.favoritecolor;
render() {
return (
<div>
<h1>My Favorite Color is {this.state.favoritecolor}</h1>
<div id=”mydiv”></div>
</div>
ReactDOM.render(<Header />, document.getElementById(‘root’));
Unmounting
The next phase in the lifecycle is after a component is removed from the DOM, or unmounting as React likes to call it.
React has only one built-in method that gets called after a component is unmounted:
- componentWillUnmount()
componentWillUnmount
The componentWillUnmount method is called after component is removed from the DOM.
Example:
Click the button to delete the header:
class Container extends React.Component {
constructor(props) {
super(props);
this.state = {show: true};
delHeader = () => {
this.setState({show: false});
render() {
let myheader;
if (this.state.show) {
myheader = <Child />;
return (
<div>
{myheader}
<button type=”button” onClick={this.delHeader}>Delete Header</button>
</div>
class Child extends React.Component {
componentWillUnmount() {
alert(“The component named Header is about to be unmounted.”);
render() {
return (
<h1>Hello World!</h1>
ReactDOM.render(<Container />, document.getElementById(‘root’));
Conclusion
In this blog we have been gone through the concept of components of react js , its types and the life cycle of the components
To read more about components you can visit here
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK