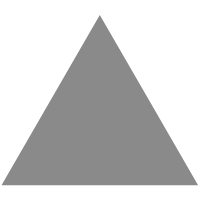
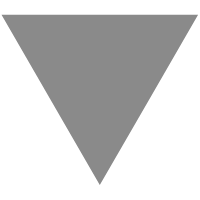
Laravel 8 CRUD operation tutorial for beginners
source link: https://www.laravelcode.com/post/laravel-8-crud-operation-tutorial-for-beginners
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
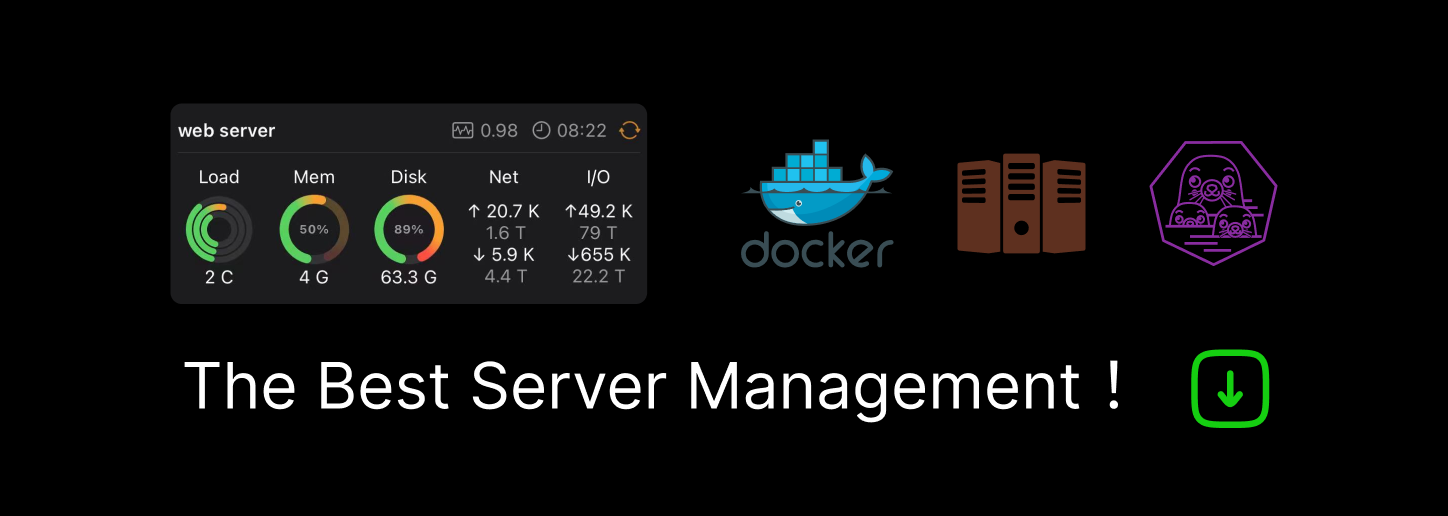
Laravel 8 CRUD operation tutorial for beginners
When you start learning any programming, you start building basic application functionalities. CRUD is the basic things you build in any application.
In this tutorial, I have created simple CRUD application for beginners from the scratch. So if you are new to Laravel, this tutorial will help you to understand the basic MVC structure of Laravel.
We are going to build blog application which includes creating, editing showing and deleting post. We will go through step by step from the starting. You can also build the Laravel CRUD application using this tutorial.
The tutorial will follows below steps:
- Step 1: Create new Laravel application
- Step 2: Configure database environment
- Step 3: Create and run database migration
- Step 4: Create Post model
- Step 5: Create application routes
- Step 6: Create PostController controller
- Step 7: Create blade view for payment button
- Step 8: Test CRUD application
Prerequisite: We assume that you have already installed LAMP server into your Ubuntu system. We also assument that you have installed Composer which is used to create Laravel application and managing PHP packages.
So, let's start from creating fresh Laravel application.
Step 1: Create new Laravel application
We are going to start tutorial from creating new Laravel 8 application. We will use Composer application command to create latest Laravel application. To start with tutorial, open the Terminal or CMD and run the below Composer command to create Laravel application.
composer create-project laravel/laravel blog --prefer-dist
After the project is created, change Terminal directory to project root.
cd blog
Step 2: Configure database environment
In the second step, we will setup database and configure into our Laravel application. Laravel database configuration are stored at .env file of root directory. Open the .env
file and change the database with your MySQL database and credentials.
DB_CONNECTION=mysql
DB_HOST=127.0.0.1
DB_PORT=3306
DB_DATABASE=blog
DB_USERNAME=root
DB_PASSWORD=root
Step 3: Create and run database migration
After we have setup database, we need to create tables into database. For that first create migration class for database table. Run the following command into Terminal to create migration file.
php artisan make:migration create_posts_table
This will create migration file at database/migrations
directory. Open that file and add new fields into up() method.
<?php
use Illuminate\Database\Migrations\Migration;
use Illuminate\Database\Schema\Blueprint;
use Illuminate\Support\Facades\Schema;
class CreatePostsTable extends Migration
{
/**
* Run the migrations.
*
* @return void
*/
public function up()
{
Schema::create('posts', function (Blueprint $table) {
$table->id();
$table->string('title');
$table->text('body');
$table->timestamps();
});
}
/**
* Reverse the migrations.
*
* @return void
*/
public function down()
{
Schema::dropIfExists('posts');
}
}
Now run the migrate command into database.
php artisan migrate
This will create posts table into database with other few default tables.
Step 4: Create Post model
Model classes are used to communicate with database and stored at app/Models
directory. Generate new Post model using following Artisan command:
php artisan make:model Post
Step 5: Create application routes
Now we need to create application routes. We are building CRUD application, so you can define resource route. Open application route file routes/web.php
and add following new resource route.
<?php
use Illuminate\Support\Facades\Route;
use App\Http\Controllers\PostController;
Route::resource('post', PostController::class);
Step 6: Create PostController controller
We have defined routes which redirects to PostController
controller class. So, create resource controller class using following Artisan command.
php artisan make:controller PostController --resource
This will create new resource controller class at app/Http/Controllers/PostController.php
file. The resource class includes following methods with routes.
Verb URI Action Route Name
GET /post index post.index
GET /post/create create post.create
POST /post store post.store
GET /post/{id} show post.
GET /post/{id}/edit edit post.edit
PUT/PATCH /post/{id} update post.update
DELETE /post/{id} destroy post.destroy
Now add the below codes into each method.
<?php
namespace App\Http\Controllers;
use App\Models\Post;
use Illuminate\Http\Request;
class PostController extends Controller
{
/**
* Display a listing of the resource.
*
* @return \Illuminate\Http\Response
*/
public function index()
{
$posts = Post::orderBy('id', 'desc')
->get();
return view('post.index', compact('posts'));
}
/**
* Show the form for creating a new resource.
*
* @return \Illuminate\Http\Response
*/
public function create()
{
return view('post.create');
}
/**
* Store a newly created resource in storage.
*
* @param \Illuminate\Http\Request $request
* @return \Illuminate\Http\Response
*/
public function store(Request $request)
{
$input = $request->validate([
'title' => 'required',
'body' => 'required',
]);
Post::create($input);
return redirect()->route('post.index');
}
/**
* Display the specified resource.
*
* @param int $id
* @return \Illuminate\Http\Response
*/
public function show($id)
{
$post = Post::find($id);
return view('post.show', compact('post'));
}
/**
* Show the form for editing the specified resource.
*
* @param int $id
* @return \Illuminate\Http\Response
*/
public function edit($id)
{
$post = Post::find($id);
return view('post.edit', compact('post'));
}
/**
* Update the specified resource in storage.
*
* @param \Illuminate\Http\Request $request
* @param int $id
* @return \Illuminate\Http\Response
*/
public function update(Request $request, $id)
{
$input = $request->validate([
'title' => 'required',
'body' => 'required',
]);
Post::where('id', $id)
->update($input);
return redirect()->route('post.index');
}
/**
* Remove the specified resource from storage.
*
* @param int $id
* @return \Illuminate\Http\Response
*/
public function destroy($id)
{
Post::find($id)
->delete();
return redirect()->route('post.index');
}
}
Your Post model file is at app/Models/Post.php
. Add $fillable attribute array with posts table fields name in the model.
protected $fillable = [
'title',
'body',
];
Step 7: Create blade view
Now we need to create blade view files to show and handle the data. Laravel view files are stored at /resource/views/
directory. We need following blade files.
File Used for
layouts/app.blade.php main layout file
post/index.blade.php show list for posts
post/show.blade.php show specific post
post/create.blade.php create post
post/edit.blade.php edit post
layouts/app.blade.php
<!doctype html>
<html>
<head>
<meta charset="utf-8">
<title>Post</title>
<link href="https://cdn.jsdelivr.net/npm/[email protected]/dist/css/bootstrap.min.css" rel="stylesheet">
</head>
<body>
<div class="container mt-5">
@yield('content')
</div>
</body>
</html>
post/index.blade.php
@extends('layouts.app')
@section('content')
<div class="row m-3">
<div class="mb-3">
<a href="{{ route('post.create') }}" class="btn btn-success">Create new post</a>
</div>
<table class="table table-bordered table-hover">
<thead>
<tr>
<th scope="col">#</th>
<th scope="col">Title</th>
<th scope="col">Body</th>
<th scope="col">Action</th>
</tr>
</thead>
<tbody>
@forelse ($posts as $post)
<tr>
<th scope="row">{{ $loop->index + 1 }}</th>
<td>{{ $post->title }}</td>
<td>{{ $post->body }}</td>
<td>
<a href="{{ route('post.show', $post->id) }}" class="btn btn-primary m-1">Show</a>
<a href="{{ route('post.edit', $post->id) }}" class="btn btn-primary m-1">Edit</a>
<a href="#" class="btn btn-danger m-1" onclick="document.getElementById('delete-post-{{ $post->id }}').submit();">Delete</a>
<form method="post" action="{{ route('post.destroy', $post->id) }}" id="delete-post-{{ $post->id }}" style="display: none;">
@csrf
@method('DELETE')
</form>
</td>
</tr>
@empty
<tr>
<td colspan="4" class="text-center">No posts found.</td>
</tr>
@endforelse
</tbody>
</table>
</div>
@endsection
post/create.blade.php
@extends('layouts.app')
@section('content')
<div class="row m-3">
<form method="post" action="{{ route('post.store') }}">
@csrf
<div class="mb-3">
<label class="form-label" for="title">Title</label>
<input type="text" name="title" class="form-control">
@error('title')
<div class="alert alert-danger">{{ $message }}</div>
@enderror
</div>
<div class="mb-3">
<label class="form-label" for="body">Body</label>
<textarea name="body" class="form-control"></textarea>
@error('body')
<div class="alert alert-danger">{{ $message }}</div>
@enderror
</div>
<input type="submit" class="btn btn-success">
<a href="{{ route('post.index') }}" class="btn btn-warning">Back</a>
</form>
</div>
@endsection
post/show.blade.php
@extends('layouts.app')
@section('content')
<div class="row">
<div class="my-3">
<ul class="list-group">
<li class="list-group-item">Title: {{ $post->title }}</li>
<li class="list-group-item">Body: {{ $post->body }}</li>
</ul>
</div>
<div class="mt-3">
<a href="{{ route('post.index') }}" class="btn btn-warning">Back</a>
</div>
</div>
@endsection
post/edit.blade.php
@extends('layouts.app')
@section('content')
<div class="row m-3">
<form method="post" action="{{ route('post.update', $post->id) }}">
@csrf
@method('PATCH')
<div class="mb-3">
<label class="form-label" for="title">Title</label>
<input type="text" name="title" value="{{ $post->title }}" class="form-control">
@error('title')
<div class="alert alert-danger">{{ $message }}</div>
@enderror
</div>
<div class="mb-3">
<label class="form-label" for="body">Body</label>
<textarea name="body" class="form-control">{{ $post->body }}</textarea>
@error('body')
<div class="alert alert-danger">{{ $message }}</div>
@enderror
</div>
<input type="submit" class="btn btn-success">
<a href="{{ route('post.index') }}" class="btn btn-warning">Back</a>
</form>
</div>
@endsection
Step 8: Test CRUD application
SO far we need to completed the CRUD application. Now we need to test the application. First, start the Laravel server using below command.
php artisan serve
And in your browser window, run the url http://localhost:8000/post
and try to add, edit and remove post.
Conclusion
Finally, we have completed the Laravel 8 simple CRUD operation tutorial. So this way, you can implement the CRUD operation in your learning curves. I hope this tutorial is helpful for those who are new and want to start from the scratch into Laravel.
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK