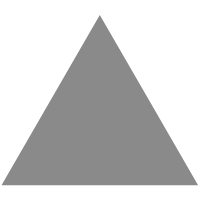
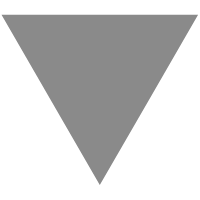
How to prevent null checks from your code!!
source link: https://blog.knoldus.com/how-to-prevent-null-checks-from-your-code/
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
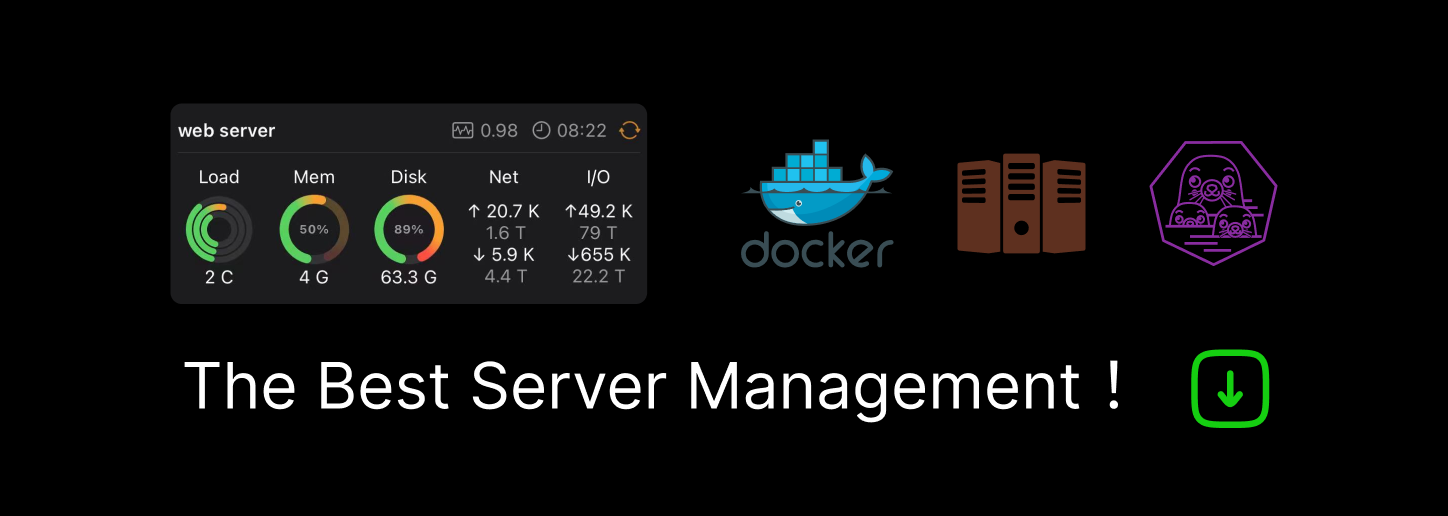
How to prevent null checks from your code!!
Reading Time: 2 minutes
Today we are going to discuss how to remove or prevent null checks from your code which actually looks ugly in code. To remove these kinds of check we are going to use one library which is provided by apache commons to help you, especially when you are dealing with null objects.
Firstly, we will go through one problem then we will see why we need this library to resolve these types of issues or problems.
package com.knoldus;
import java.util.ArrayList; import java.util.stream.Collectors;
public class LambdaEx {
public static void main(String[] args) { ArrayList<String> list1 = null; list1.stream().map(elem –> elem + "Knoldus").collect(Collectors.toList()); list1.forEach(System.out::println); } }
As we can see in the above example, the list is assigned with null so when we tried to perform any operation on that list we got NullPointerException which is correct behavior as well in case of null but we should always handle these kinds of scenarios very gracefully and beautifully. So, we will see how we can handle these scenarios gracefully by using apache commons.
package com.knoldus;
import org.apache.commons.collections4.CollectionUtils;
import java.util.ArrayList; import java.util.List; import java.util.stream.Collectors;
public class LambdaEx {
public static void main(String[] args) { ArrayList<String> list = null; final List<String> modifiedCollection = CollectionUtils.emptyIfNull(list) .stream() .map(elem –> elem + " ") .collect(Collectors.toList());
CollectionUtils.emptyIfNull(modifiedCollection).forEach(System.out::println); } }
So, as we can see in the above example we handled the NullPointerException very gracefully with the CollectionUtils which is provided by apache commons.
Now, we will take one more scenario where you are calling one function which is returning a List<//something> then after taking that value from that function you are doing any computation by using that list. So, suppose the list is coming null to you and you are performing some computation logic, then it is obvious you will get the NullPointerException which we need to handle to cover all scenarios because we are not sure that function will always give the list with values.
Now, we will go one step deeper where what we are going to check is if the given list or any collection is not null but one of its value is null. So, how to handle that case as well which occurs in real life scenarios very usually.
Note: We will handle this case with the ObjectUtils which is also provided by apache commons.
package com.knoldus;
import org.apache.commons.collections4.CollectionUtils; import java.util.ArrayList; import java.util.List; import java.util.stream.Collectors;
public class LambdaEx {
public static void main(String[] args) { final List<String> names = new ArrayList<>(); names.add("Kunal"); names.add("Akshansh"); names.add(null);
final List<String> modifiedCollection = CollectionUtils.emptyIfNull(names) .stream() .filter( name –> name.equalsIgnoreCase("Kunal")) .map(name –> name + "Sethi") .collect(Collectors.toList());
CollectionUtils.emptyIfNull(modifiedCollection).forEach(System.out::println);
} }
We can see the above example where we are getting NullPointerException while performing some operations on given collection values. So, this is the time to see how we can remove or fix this problem in a mannered way.
import org.apache.commons.collections4.CollectionUtils; import org.apache.commons.lang3.ObjectUtils;
import java.util.ArrayList; import java.util.List; import java.util.stream.Collectors;
public class ObjectUtilsEx {
public static void main(String[] args) { final List<String> names = new ArrayList<>(); names.add("Kunal"); names.add("Akshansh"); names.add(null);
final List<String> modifiedCollection = CollectionUtils.emptyIfNull(names) .stream() .filter(ObjectUtils::anyNotNull) .filter( name –> name.equalsIgnoreCase("Kunal")) .map(name –> name + " Sethi") .collect(Collectors.toList());
CollectionUtils.emptyIfNull(modifiedCollection).forEach(System.out::println);
} }
As we can see in the above example we handled that NullPointerException case with the help of ObjectUtils class and in a very beautiful way.
I hope this blog was valuable, and you have gained an understanding of how to prevent and handle null checks in code.
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK