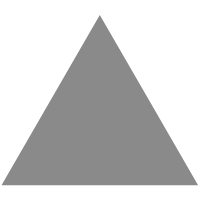
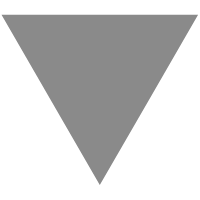
Comparing Previous useEffect Values in React
source link: https://dev.to/mcavaliere/comparing-previous-useeffect-values-in-react-2o4g
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
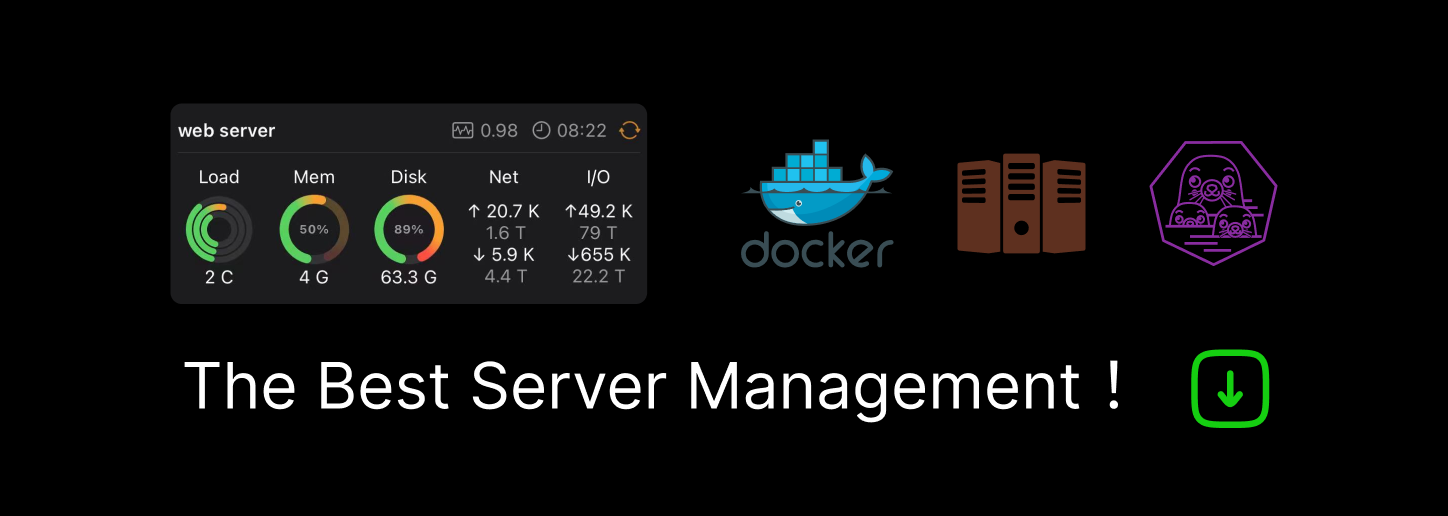
Comparing Previous useEffect Values in React
With functional components now being the standard in React, we lost one notable perk of using the lifecycle hooks (such as componentDidUpdate()
) of class-based components: the intrinsic ability to compare previous values to new ones.
If I wanted to respond to a component's "count" change for example, I could do something like:
componentDidUpdate(prevProps, prevState) {
if (this.props.count > prevProps.count) {
// Count incremented! Do something.
}
}
Enter fullscreen mode
Exit fullscreen mode
I came across the need to do this while working on Emoji Battle yesterday—I wanted to show an animation anytime an emoji's vote count incremented.
Luckily Stack Overflow had a great solution as usual, which I turned into a hook I'll probably reuse in the future.
Basically you create a very simple custom hook that uses a React ref to track the previous value, and refer to it in the useEffect.
function usePreviousValue(value) {
const ref = useRef();
useEffect(() => {
ref.current = value;
});
return ref.current;
}
Enter fullscreen mode
Exit fullscreen mode
Based on this, I used it to increment my Emoji counter as follows:
export const Count = ({ value }) => {
const controls = useAnimation();
const previousValue = usePreviousValue(value);
useEffect(() => {
if (!previousValue || value > previousValue) {
// Trigger the framer-motion animation
controls.start({
scale: [1, 1.5, 1],
transition: {
duration: 0.5,
},
});
}
}, [value]);
Enter fullscreen mode
Exit fullscreen mode
Try this usePreviousValue
hook out next time you need to track value changes in React functional components.
Recommend
-
34
Yeah, this article is based on Advance hooks, so I assume that you are aware of hooks and familiar with the usage of basic hooks like useState and useEffect. So if you don’t know about hooks you can look it up in the
-
36
-
18
React Hooks: 深入剖析 useMemo 和 useEffectLxylona一棵需要定期修剪的树...
-
8
Comparing enums in Swift is very straightforward – as long as they don’t have associated values. In this post we will discuss, what you can do in that case. Hint: This post is using Swift 4 Content
-
4
React 源码版本: v16.11.0 源码注释笔记:airingursb/react 1. useEffect 简介 1.1 为什么要有 useEffect 我们在前文中说到 Reac...
-
9
I am impressed by the expressiveness of React hooks. You can do so much by writing so little. But the brevity of hooks has a price — they’re relatively difficult to get started. Especially useEffect() — the hook that ma...
-
7
How to Solve the Infinite Loop of React.useEffect()useEffect() React hook manages the side-effects like fetching over the network, manipulating DOM directly, starting and ending timers. While the useEffect...
-
4
The React useEffect Hook helps you manage side-effects in functional React components. It also makes this task much easier than it used to be. In this tutorial you will learn about what useEffect hook is and how it works. You will also learn...
-
4
React 17 runs useEffect cleanup functions asynchronously Jun 11, 2021 , by Chetan Gawai 3 minute read Effect cleanup functi...
-
6
Comparing CSS Specificity values Kilian ValkhofBuilding tools that make developers awesome. Search term Comparing CSS Specificity values
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK