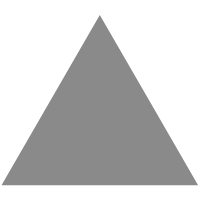
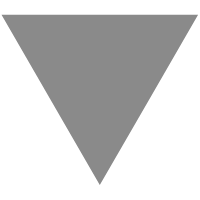
How To Change Column Order Using Pandas
source link: https://www.journaldev.com/54321/change-column-order-using-pandas
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
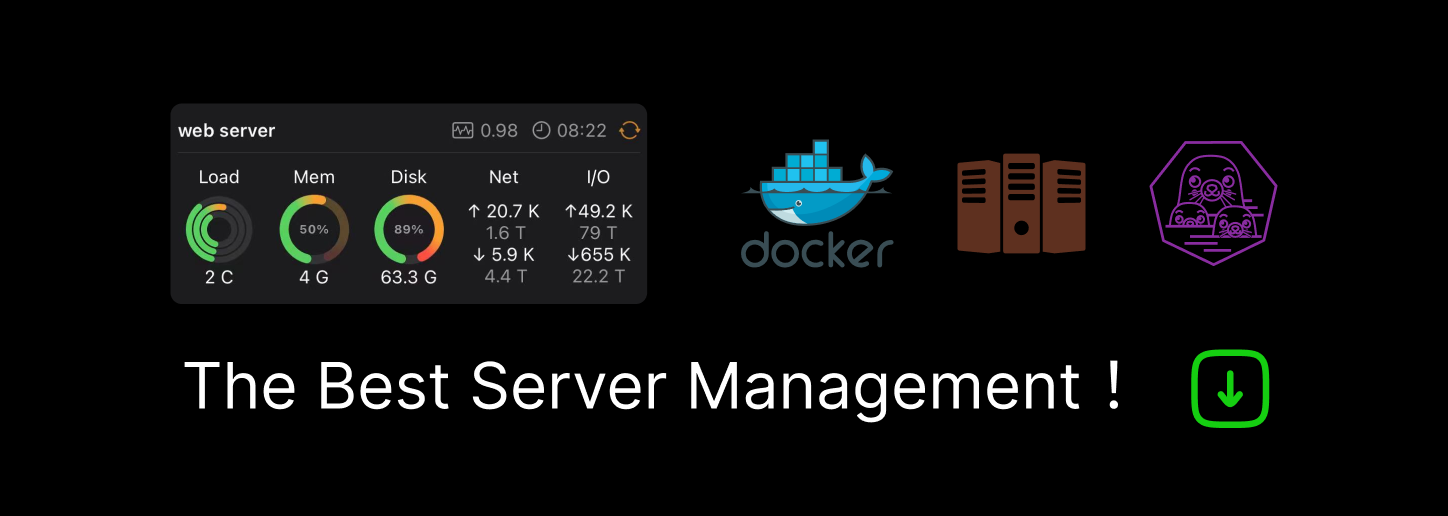
How To Change Column Order Using Pandas
In this article, we’ll learn how to change column order using Pandas. Pandas is an invaluable part of the data science world. For all your data manipulation and analysis, Pandas offers many amazing functions which can help you in the process. Pandas support dataframe objects to store data that has labeled rows and columns.
As you already know, data.columns
function can list out all the columns / variable names in your data. But, how can you change the order of the columns?. Well, it’s an interesting question and I got multiple methods for it.
So, without spending much time on Pandas, let’s see 4 different methods, using which you can change column order in python.
Change Column Order Using Pandas
As a first step, we have to import the required libraries for this purpose. We need Numpy and Pandas to work with data and our data will be a “titanic” dataset.
#import libraries
import
numpy as np
import
pandas as pd
Let’s load the data using the Pandas read_csv() function.
#data
import
pandas as pd
data
=
pd.read_csv(
'titanic.csv'
)

Here is our Titanic dataset. Now, we are going to print out the column / variable names in this data as a list.
#columns
data.columns
Index(['PassengerId', 'Survived', 'Pclass', 'Name', 'Sex', 'Age', 'SibSp',
'Parch', 'Ticket', 'Fare', 'Cabin', 'Embarked'],
dtype='object')
Also check for duplicate columns / variables.
#check for duplicates
data.columns.value_counts()
PassengerId 1
Fare 1
Embarked 1
Sex 1
Ticket 1
Pclass 1
Age 1
Survived 1
Parch 1
Name 1
Cabin 1
SibSp 1
dtype: int64
So, we don’t have any duplicate columns in our data. We are good to go 🙂
1. Pandas iloc Method
Using the Pandas iloc method, you can index or change column order in a specified order as shown below.
#iloc method
data.iloc[:, [
3
,
5
,
4
,
9
,
2
]]

- It may look like a VLOOKUP table but it’s not. As shown above, you can specify the order of the column to arrange them as shown.You can play with a different order as per your use case.
Also read: Pandas Indexing: loc, iloc, and ix in Python
2. Pandas loc Method
Yes, using pandas loc method also, you can change column order in the data. Let’s see how it works!
#loc method
data.loc[:, [
'Name'
,
'Age'
,
'Sex'
,
'Fare'
,
'Pclass'
]]

This pandas loc method also produces the same output. But, take some time and observe the difference between the working nature of these 2 methods.
In the iloc method, we specify integer input. But, in the loc method, you can pass both label and integer input. I have added an informative picture of the difference between the iloc and loc methods.
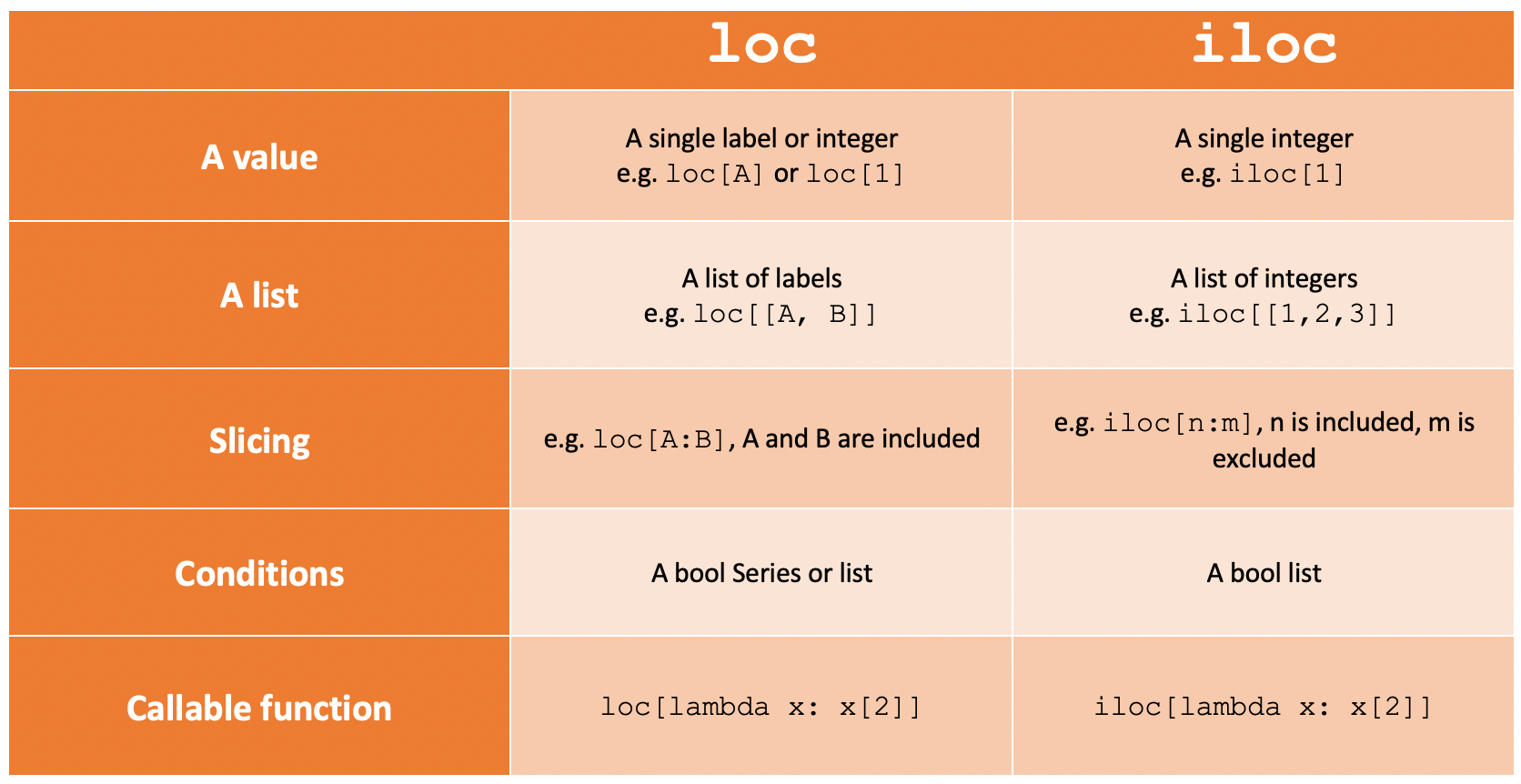
- Above, I have mentioned the same order as the iloc method to show the working difference of them as well. Feel free to change the order and get your hands dirty with your data.
3. Pandas Subset Method
The pandas’ sub-setting method is one of the simplest methods among the above methods. You have to subset the data with a required order.
#subset
data[[
'Name'
,
'Age'
,
'Sex'
,
'Fare'
,
'Pclass'
]]

Well, we got the desired output. Just like this, you can subset the data with a use-case-specific order to get a newly ordered dataframe as shown above.
Note that you can not only order the columns but also slice them and extract the required data.
4. Pandas Reverse
The final method is using the pandas reverse. But, I don’t think it can be a very useful method. Because this method will just reverse the order of the data 😛
Example;
A -> Z,
Z -> A
#revese
rev_columns
=
list
(data.columns)
rev_columns.reverse()
data[cols]

Well, we have reversed the order of all the columns. This is how it works! Let me know your thoughts on this method in the comments!
Change Column Order In Pandas – Wrapping Up
Sometimes you may need to change the column order of your data for a use case and you can use any of the above-shown methods based on your requirement. Pandas offer many functions, which help amazingly in our data analysis and wrangling. I have covered 4 methods in this story and probably they can be handy at some time.
That’s all for now! Happy Python!!! 🙂
More read: Pandas documentation (iloc)
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK