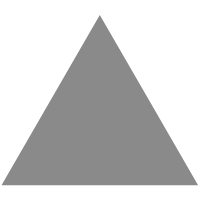
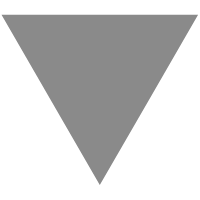
Use Socket.IO With Nuxt.js/Vue.js
source link: https://dzone.com/articles/use-socketio-with-nuxtjsvuejs
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
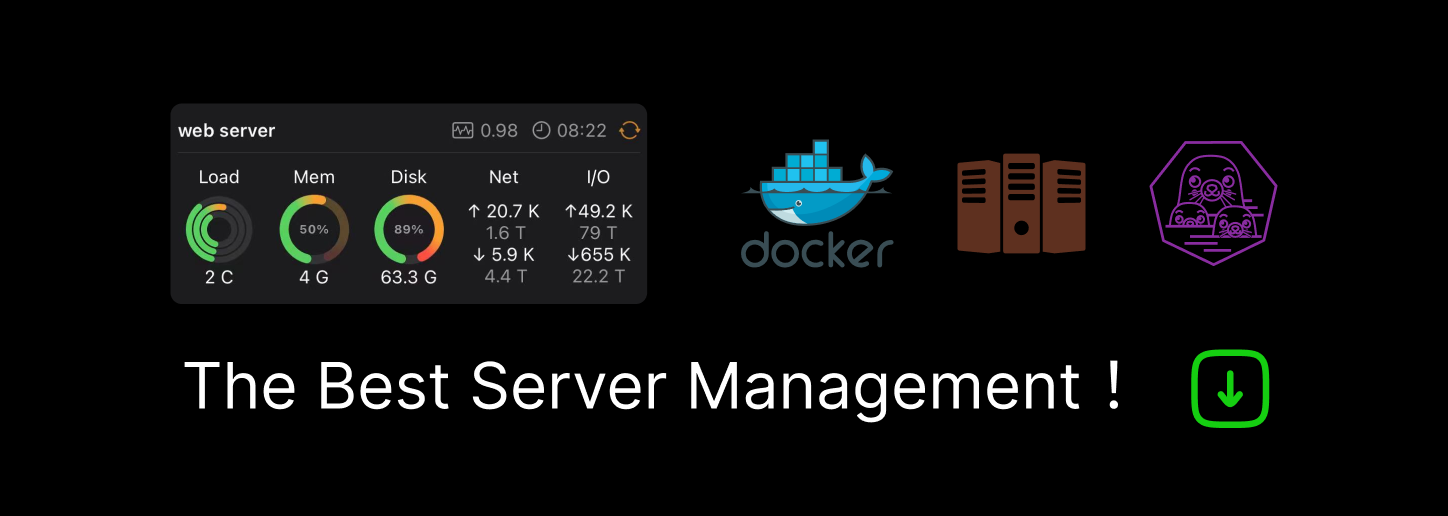
Use Socket.IO With Nuxt.js/Vue.js
Learn how to implement the Socket.IO Javascript library with Nuxt.js and Vue.js. Plus learn more information about the socket.emit and socket.on methods.
Join the DZone community and get the full member experience.
Join For FreeFor one of my recent Nuxt.js projects, I had to use Socket.IO to send the data from the server to the users in real-time without reloading the whole page. Initially, I was using Vue.js, but then the client insisted on implementing Nuxt.js for the server-side rendering purpose.
First, I used Axios as a middleware to send and receive HTTP requests. Axios is a great library to send and receive data without loading the whole page. It works great with Vue.js and Node.js. But the problem with Axios is that it only sends the response once with res.send
or res.end
method. If you try to send two or more times with these methods, then the app crashes.
To solve this issue, I used the Socket.IO library. I already used Socket.IO with Vanilla Javascript and Node.js, but I had no idea how things are done with Nuxt.js and Vue.js. I had to research to figure out how this whole thing works. For that reason, I have shared how to set up Socket.IO with Nuxt.js. You can just copy the piece and paste it anywhere you want to.
The NPM packages you need to install before getting started:
- Socket.IO: To use Socket.IO easily, you need to install Socket.IO package for Nuxt.js
- Socket.IO client: Install this package to use
socket.emit
andsocket.on
methods on the client-side
You also need Express.js installed. If you are using Nuxt.js, then it installs by default. So, apart from Socket packages, you do not need to install anything else.
Let’s dive into the code now.
Install packages
Install NPM and save socket.io
and socket.io-client
Now, open the nuxt.config.js file and update the module block:
modules: [
['~/io'],
],
For my project, I created an app that gets the IP address of a user, and based on the location, it returns the price of the cryptocurrency in their currency. For example, if a user is from the US then the tool shows data in the US dollar. Along with that, with the help of Socket.IO, the price changes in real-time. To store the data, I converted all the decimals of an IP address into hexadecimal for safety purposes and when I had to show the IP address to the user, I converted hexadecimal to decimal number. Since this article is about implementing Socket.IO, I’m going to stick to that. But just to give an idea of how a hexadecimal to decimal converter can help store data, I shared the example.
Now, open the plugins folder placed at the root. Inside of the plugins folder, create a .js file and name it "socket.io.js." Copy and paste the following code inside of that socket.io.js file:
import io from 'socket.io-client'
const socket = io(process.env.WS_URL)
export default socket
Now, create a new folder and name it "io" at the root level. Inside of that root folder, create a new file and name it "index.js." Paste the following code inside that index.js file:
import socketIO from 'socket.io'
var network = require('network');
export default function () {
this.nuxt.hook('render:before', (renderer) => {
const server = http.createServer(this.nuxt.renderer.app)
const io = socketIO(server)
// overwrite nuxt.server.listen()
this.nuxt.server.listen = (port, host) => new Promise(resolve => server.listen(port || 3000, host || 'localhost', resolve))
// close this server on 'close' event
this.nuxt.hook('close', () => new Promise(server.close))
// Add socket.io events
const messages = []
io.on('connection', (socket) => {
socket.on('last-messages', function (fn) {
network.get_public_ip(function(err, ip) {
socket.emit(‘ip’, ip); // should return your public IP address
})
var x = new BigNumber(ip, 16)
var dectobin = x.toString(10);
})
})
})
}
All the server-side work is done. Now, you need to add the following code to the front-end Vue file. I have not added the HTML (the template part in the Vue), but just the script part.
<script>
import socket from '~/plugins/socket.io.js'
export default {
data () {
return { message: 'send', messages: [], isShowing: false, isShowing1: false, isShowing2: false }
},
beforeMount () {
socket.on(ip', (ip) => {
console.log(ip)
this.messages.push(ip)
})
},
methods: {
senlocation () {
socket.emit('last-messages ')
},
},
}
</script>
Front-End and Back-End Code Explanation
Apart from the two Socket.IO methods, there is everything Vue in the code. Here, in the code, we have used two Socket.IO methods heavily, socket.emit
& socket.on
on both front-end and back-end.
Socket.emit
method: This method is used whenever you want some data or an alert from or to the server.
Socket.on
method: This method is used to listen to the socket.emit
. This method constantly watches the socket and as soon as it gets data from the server, it shows it to the user without loading the whole page.
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK