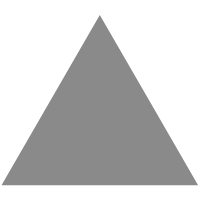
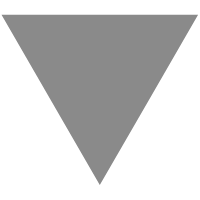
IT Enthusiast — How do you write FizzBuzz?
source link: https://rodiongork.tumblr.com/post/112134190153/how-do-you-write-fizzbuzz
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
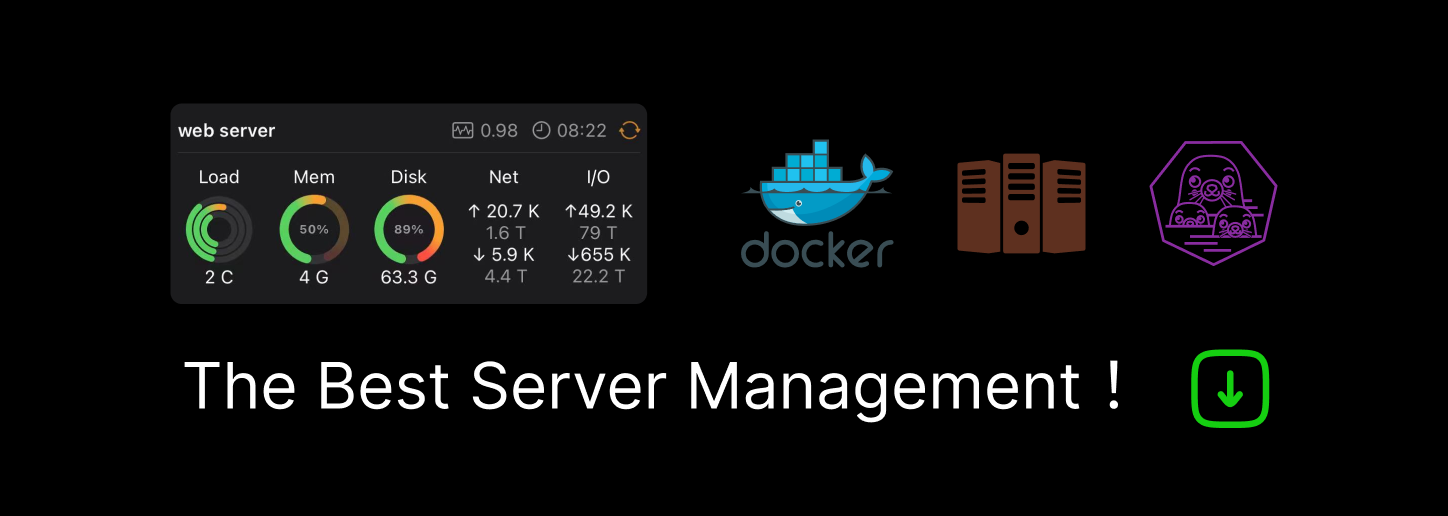
How do you write FizzBuzz?
You probably know these articles about FizzBuzz: authors point out that many developers (even experienced) are very poor in implementing simplest task when they are interviewed. Example article.
I agree with them heartily - I also meet many people who suck in basic logic and writing basic code. However, now I’m going to have a bit of fun discussing possible implementations. How could this be written? How do you write it? What are common and uncommon ways?
I dare to remind the problem statement: Print integers from 1 to 100, but for each of them divisible by 3
put Fizz
instead - and for each divisible by 5
put Buzz
instead. If the value is divisible by both - output FizzBuzz
.
Let us see. Here is the basic approach (in Python) - it was often written by school pupils when I ask them:
for i in range(1, 101):
if i % 15 == 0:
print("FizzBuzz")
elif i % 3 == 0:
print("Fizz")
elif i % 5 == 0:
print("Buzz")
else:
print(i)
What we do not like about this code? it looks like here is more branches than necessary because we check i % 15
case separately. We can make some trick here - printing FizzBuzz
as consisting of two parts :
for i in range(1, 101):
s = ''
if i % 3 == 0:
s += 'Fizz'
if i % 5 == 0:
s += 'Buzz'
print(s if s != '' else str(i))
Could this be improved? Or made more funny? Surely! Let us use some arithmetic and small array of answers:
a = ['0', 'Fizz', 'Buzz', 'FizzBuzz']
while a[0] != '100':
v = int(a[0]) + 1
a[0] = str(v)
print(a[(1 if v % 3 == 0 else 0) + (2 if v % 5 == 0 else 0)])
At least this can puzzle your interviewer for a minute or two…
But it is not the end of heresy. A friend of mine, when I told her about FizzBuzz said that she do not remember how modulo operator is written or used. :)
But we can do without modulo, of course! Moreover, here is an example of problem where you can not use modulo (it is hard to implement in the proposed language): FizzBuzz in Asm.
Our second version is the most suitable to be modified for such a case:
cnt3 = 0
cnt5 = 0
for i in range(1, 101):
s = ''
cnt3 += 1
cnt5 += 1
if cnt3 == 3:
cnt3 = 0
s += 'Fizz'
if cnt5 == 5:
cnt5 = 0
s += 'Buzz'
print(s if s != '' else str(i))
You see, we simply use two counter running up to 3
and 5
instead of using modulo operation - though this makes solution bit longer. But still it could be a useful trick for cases for platforms where division / modulo operations are costly.
So here are a few of my solutions. Would you like to propose some of your own - probably, more funny? Please feel free to paste them in comments!
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK