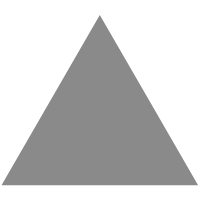
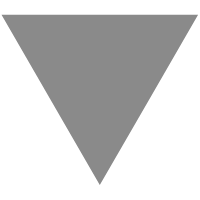
Relaible and Secure API: How to Test with Hoppscotch
source link: https://hackernoon.com/relaible-and-secure-api-how-to-test-with-hoppscotch
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
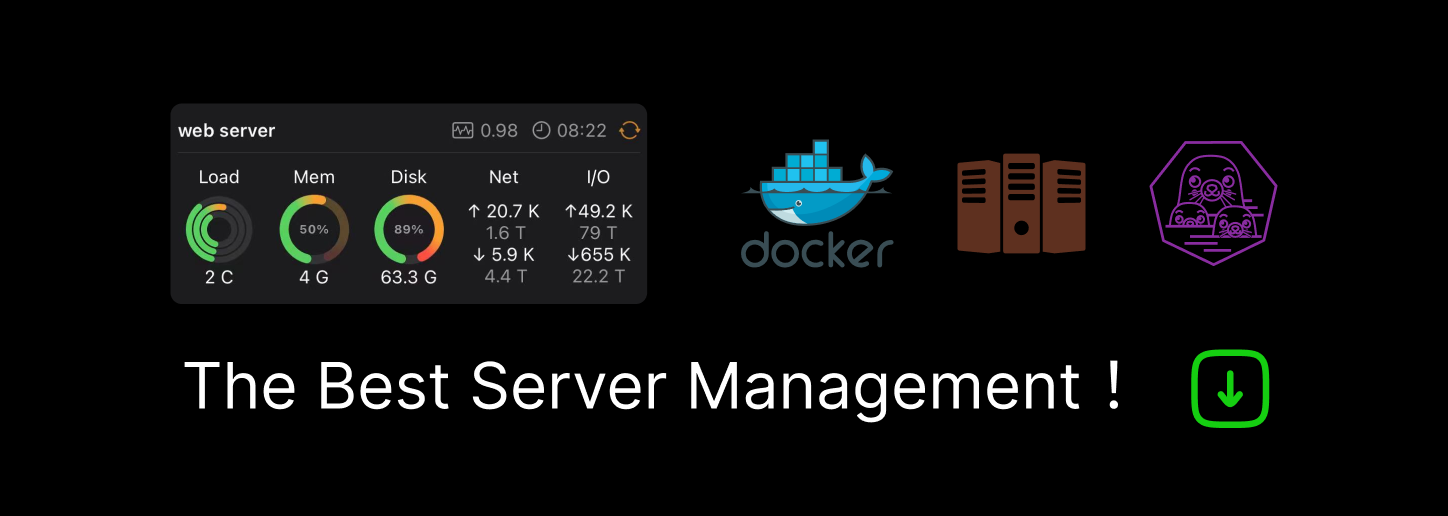
I m a self learnt Node JS Developer from India.
In this blog, we will study how API Testing is done in Hoppscotch by writing pre-request scripts and test scripts to make sure the API is highly secured and reliable because if our API is not secure & reliable, it will crash just like Tom.
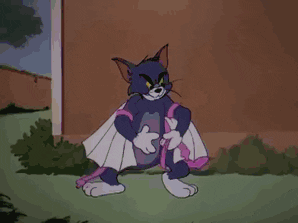
Flying Tom
What’s API
API stands for Application-Programming-Interface. It is the middle layer between User Interface and the Backend Server. You can take API
as a waiter in a restaurant, where User requests
are customers and Server
is the Chef in the Kitchen. When a customer has a specific request, it is taken to the chef by the waiter. In the same way, When a user makes a specific request, it is requested to the server through API
.
Hoppscotch
Hoppscotch is a tool that helps us to build HTTP requests that we send to Servers. It is a tool that allows us to work easily with APIs. It is an HTTP Client using which we can make HTTP requests using a GUI for validating the responses we retrieve using those API requests.
How it works
The Best way of learning is by working on it practically. We will test some APIs and will write tests for them. Go to Hoppscotch.io. Let’s start creating HTTP requests. Let’s make our first HTTP GET Request on Hoppscotch using the URL:
https://api-client-1122.herokuapp.com/
Copy this URL and paste it into the URL box in Hoppscotch. And start with a simple GET Request and press Send button.
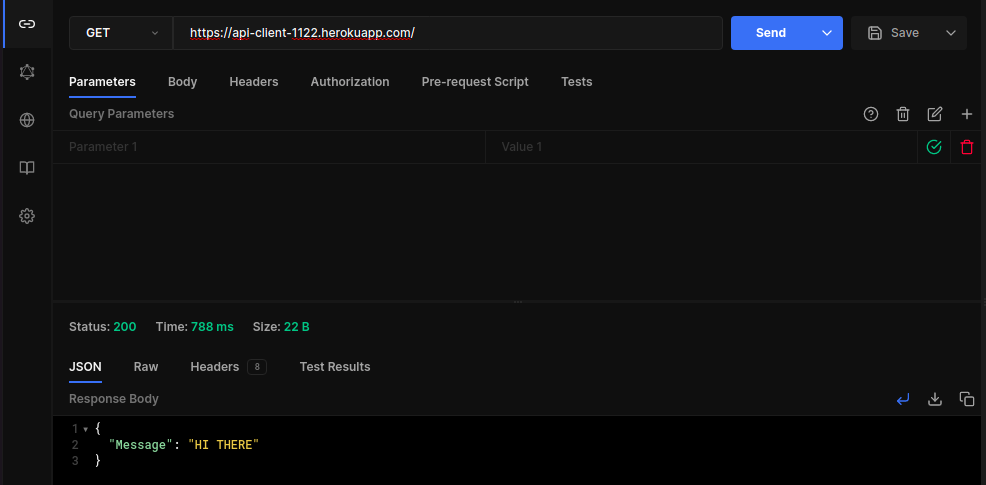
GET Request
{ "Message": "HI THERE" }
That’s super cool! Now save the response by clicking on Save
button. A new dialog box appears.
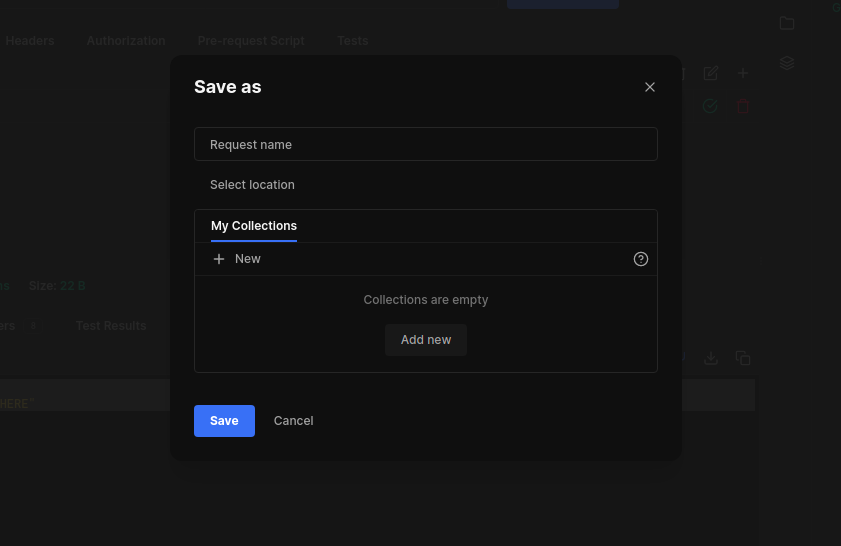
Save Button
1st Request
.Collections
So what are Collections
in Hoppscotch
?
With the help of Collections
, we can group together all the HTTP Requests of the single API Endpoint to organize it in a well-mannered way. Click on Add new
button to create a new Collection
and name it Users API
and click on save
button.
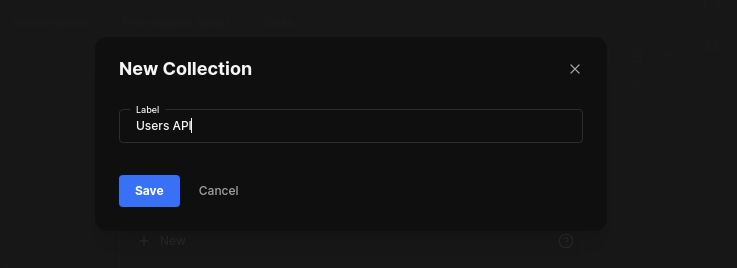
User API
User API
from My Collections
and save the request to the User API
Collection.
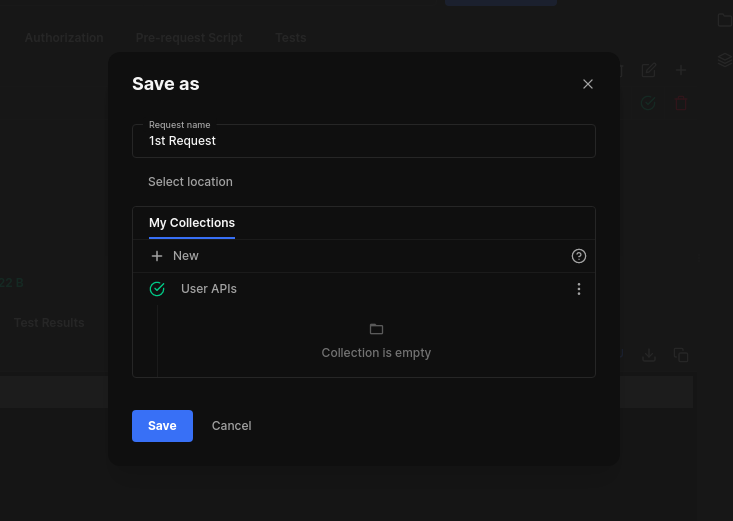
User API
Collection
successfully and saved our 1st request to the User API
Collection.Now we will try to fetch the Users list from the API. Paste the below URL for fetching the Users list from the server.
https://api-client-1122.herokuapp.com/users
Using GET Method send the request.
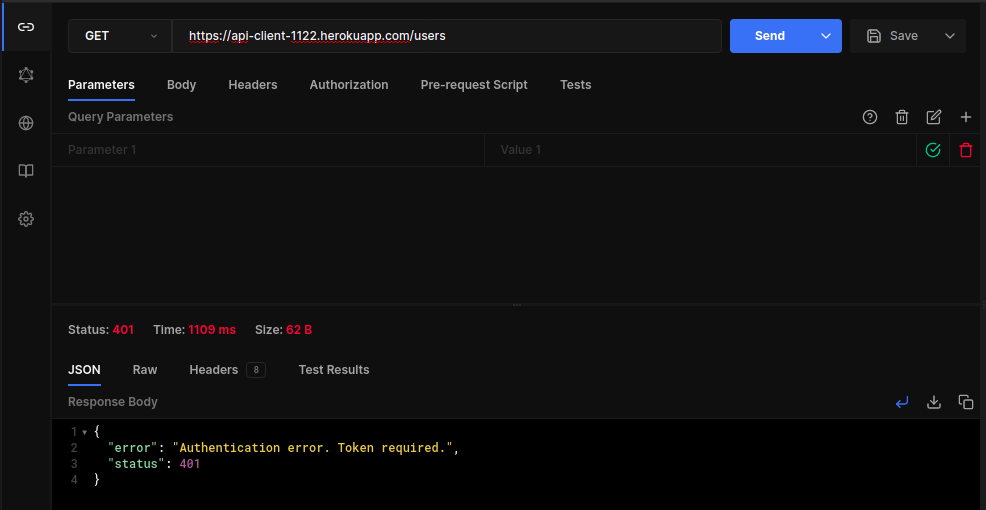
Unauthorized
POST
.And change Content Type
to application/json
because we are providing our data in json
format.
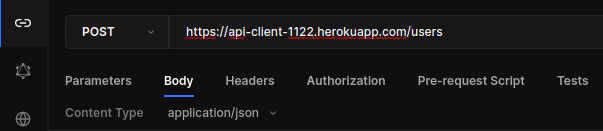
{
"username": "sankee",
"password": "IplayGTA5"
}
Add your data to the request body which you want to send with the request.
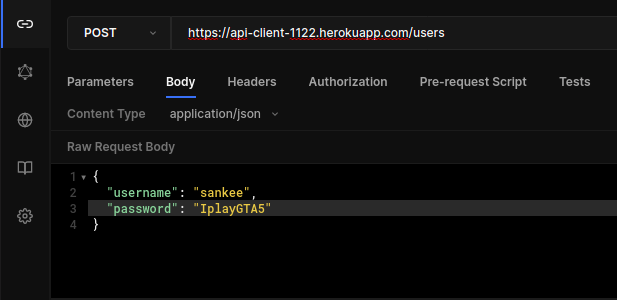
Send
button to make the request.
Wooo! We just made our first POST
request here.
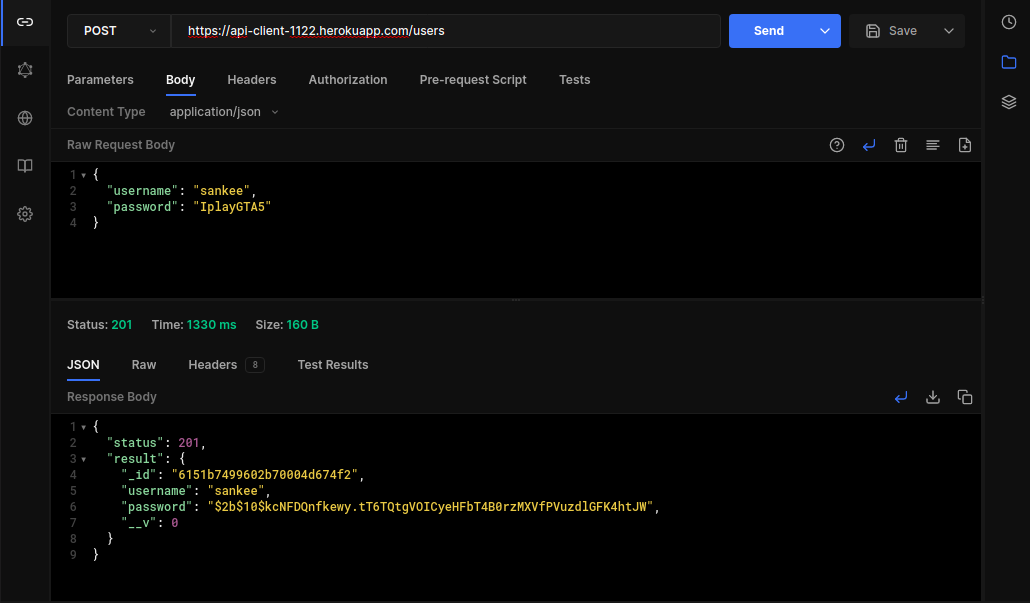
Response
{
"status": 201,
"result": {
"_id": "6151b7499602b70004d674f2",
"username": "sankee",
"password": "$2b$10$kcNFDQnfkewy.tT6TQtgVOICyeHFbT4B0rzMXVfPVuzdlGFK4htJW",
"__v": 0
}
}
Let’s move forward and generate an auth token by sending our credentials to the login
route. Add the same JSON data to the request body to generate auth token.
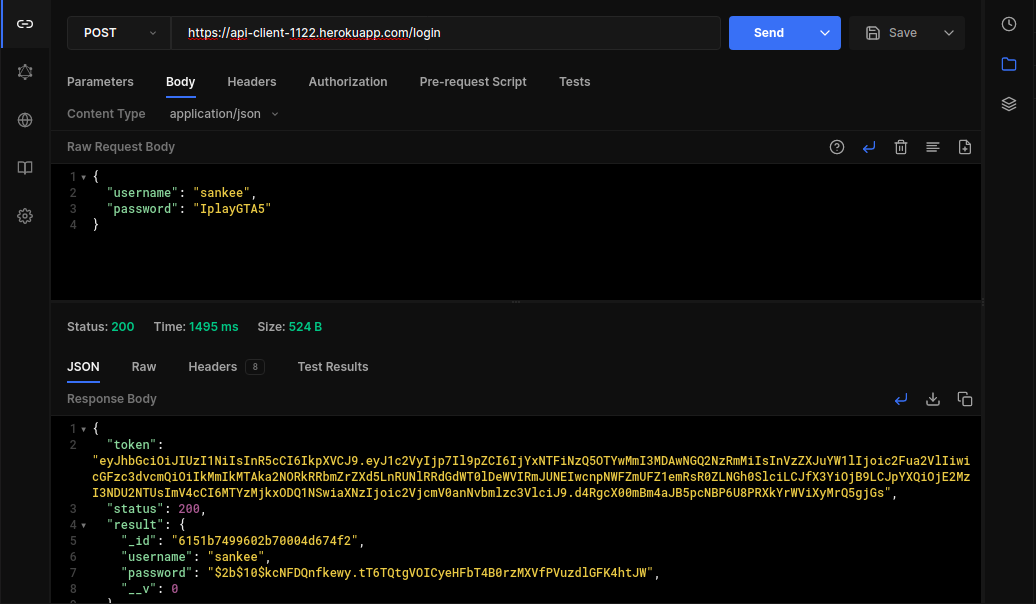
Auth token
auth token
for using it in user
route.
Save the login
route to the User API
collection by clicking on the dropdown arrow next to Save
Button and click on Save as
button. Name it and select User API
from My Collections
, Save it!.
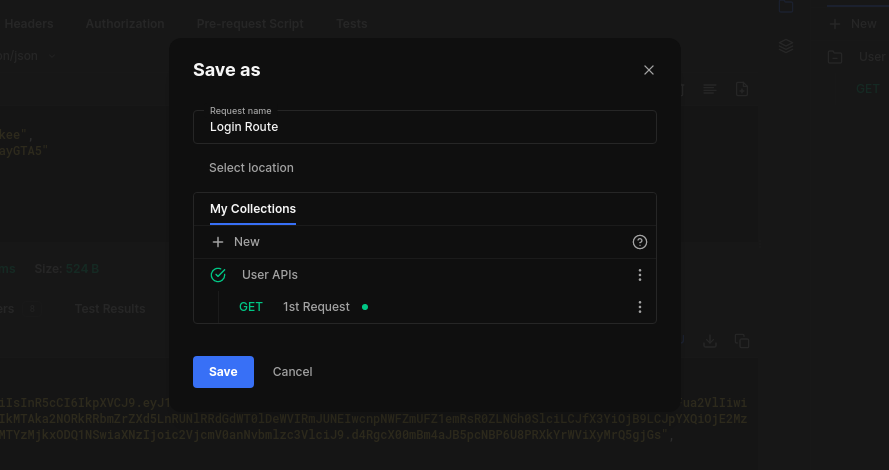
Login Route
users
route by adding the auth token
along with the request.
Click on Authorization
button below the URL box and set Authorization Type
to Bearer Token
. Paste the copied auth token
in the Token
box.
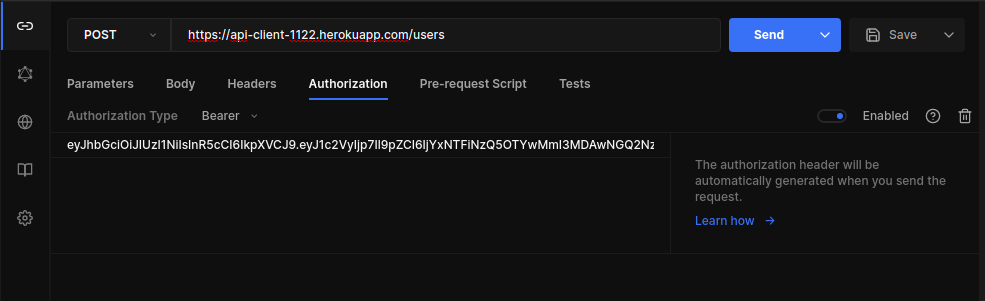
Authz
GET
Method Request to fetch the users list.
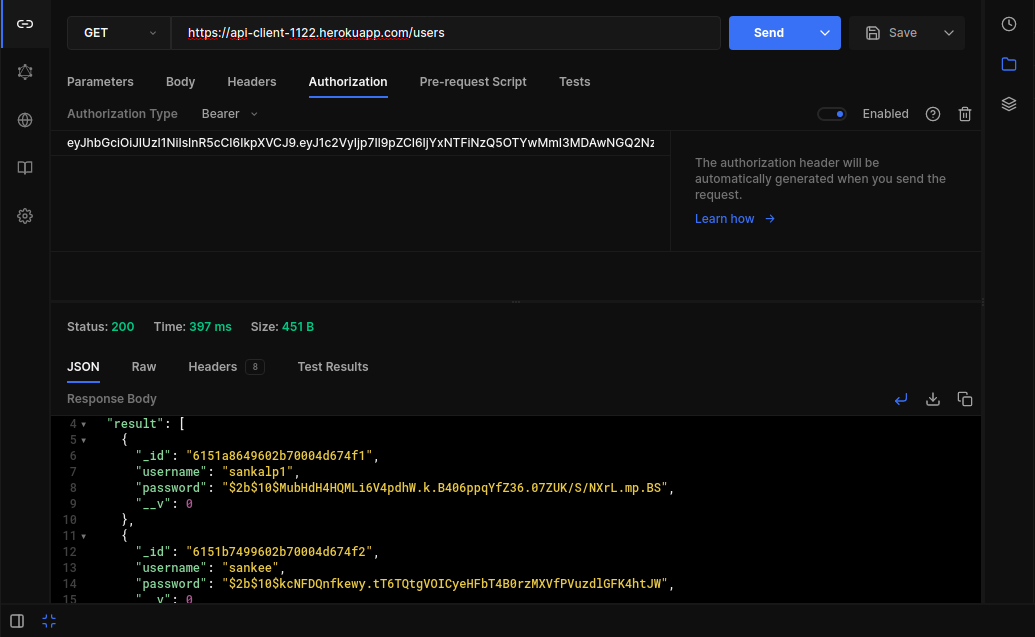
Users List
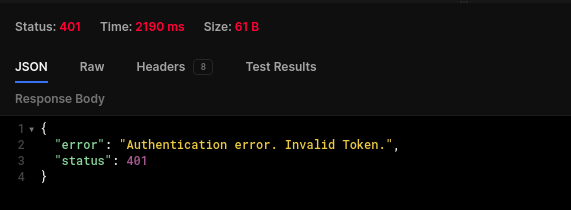
Error
Auth Token
.
Make it correct and save the /users
route to User API
collection in the same way as we did for /login
route.
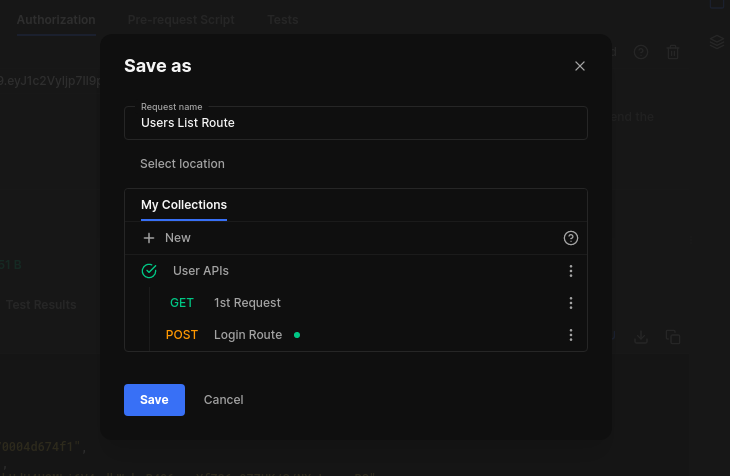
Users route
id
of one of the users for further use. 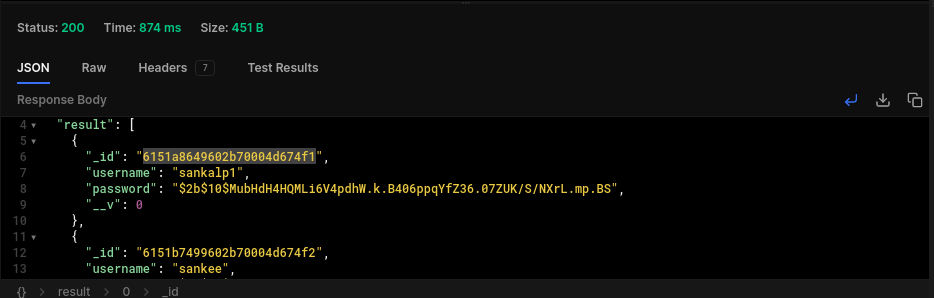
Copy ID
PUT
request. Now your URL Box will look like something of this kind.https://api-client-1122.herokuapp.com/users/6151a8649602b70004d674f1
Change the method to PUT
and add this data to the request body.
{
"username": "sankalp123"
}
Send the request.
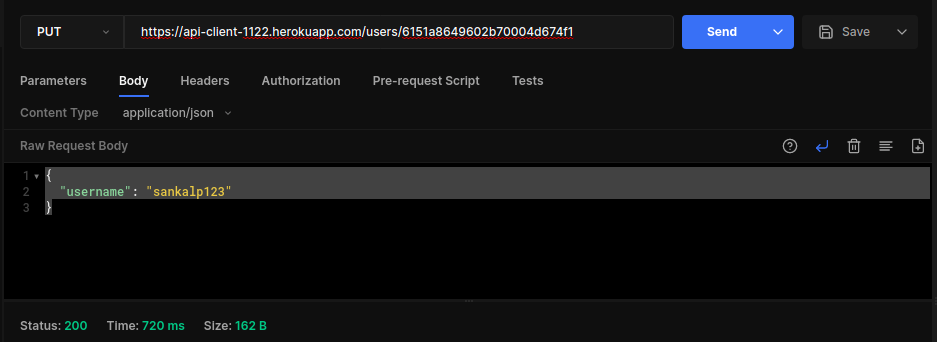
GET
request on the same route. 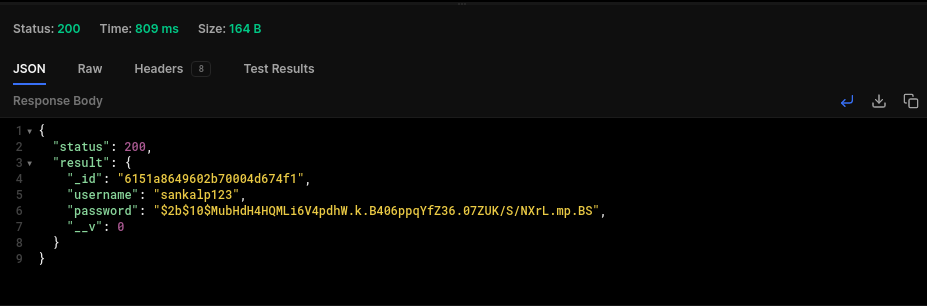
Update an User
to the User API
Collection.Environments
Our URL is getting a bit lengthy, No worries, Environments
are here for rescue. We can create variables to store data and we can use those variables in our requests. Let’s make a variable baseURL
and store our API Endpoint in it. Click on Environment
icon button next to our workspace and click on Add new
button and name it User API
.
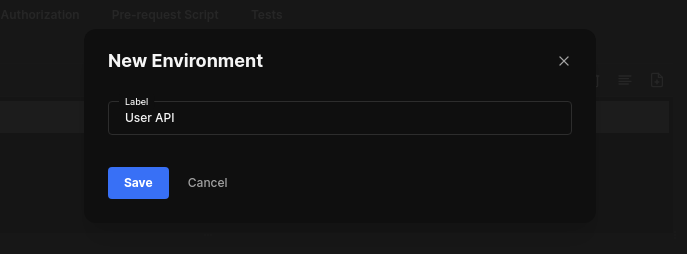
User API
and add variables to the env
. Name it baseURL
and add https://api-client-1122.herokuapp.com/
url to value
box and save it.
Switch to User API
env by clicking on No Environment
button followed by User API
button. 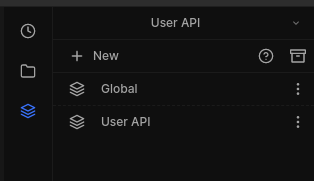
<<value>>
brackets are here. Go to URL box and replace the API with the syntax. 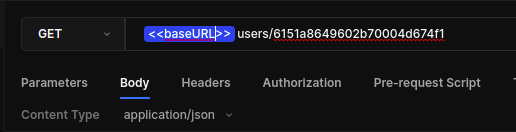
ENV VARIABLE
environment
.
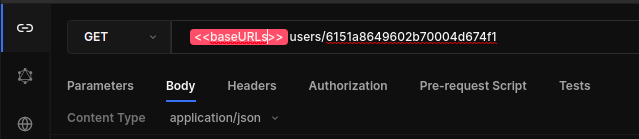
DELETE
method. Let’s delete a User by changing the Method to DELETE
and with the same id we used for updating a User Document. 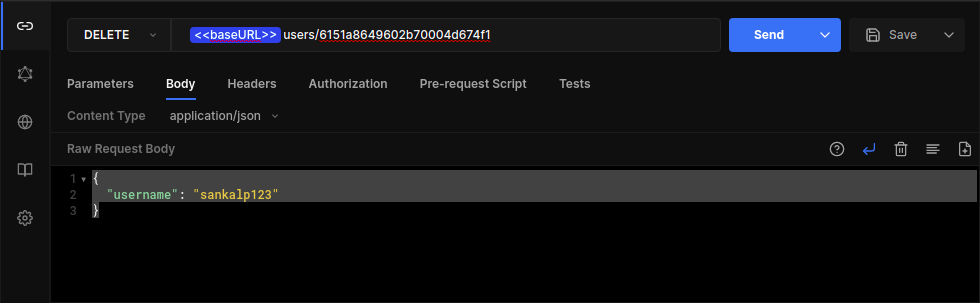
Delete
GET
request to the same URL.
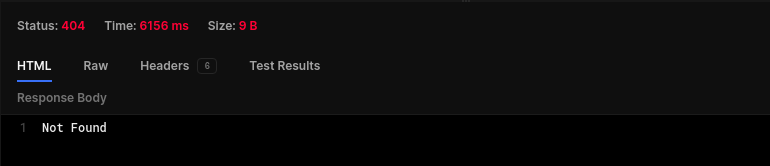
Verify
We have gone through all the common Request Methods and also organized our all requests using Collections
and created variables by using Environments
.
TESTS
Tests
are scripts written in javascript which execute after the response is received? Let’s start writing tests for our API Requests. Go to our 1st Request
and then go to the Tests
section. We have some pre-written tests on the right side of the workspace.
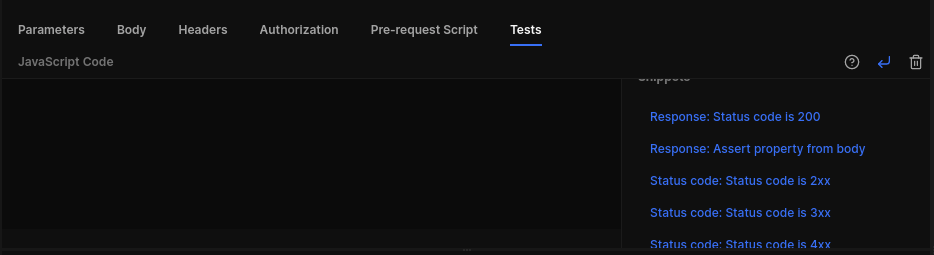
snippets
Response: status code is 200
.
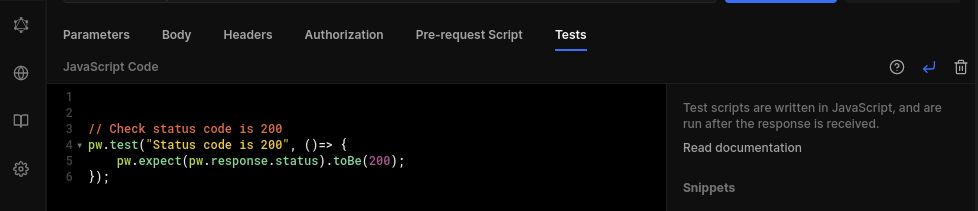
snippet200
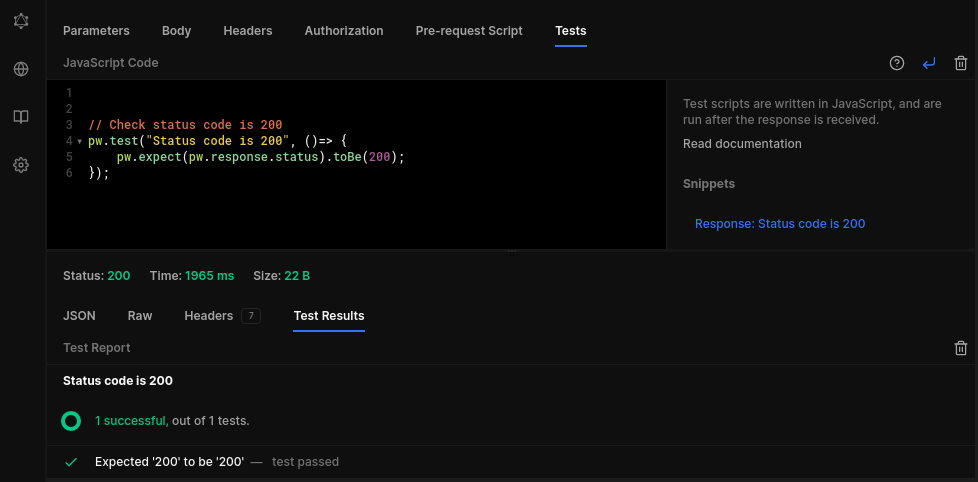
Test Result
pw.test(name, func()=>{});
Syntax is pretty much simple, we start with pw.test
followed by name
and function
for the test
.
// Check for HI THERE
pw.test("Response is HI THERE", () => {
pw.expect(pw.response.body).toBe('{"Message":"HI THERE"}')
})
Add this code to Tests
and let’s check if this fails or Passes.
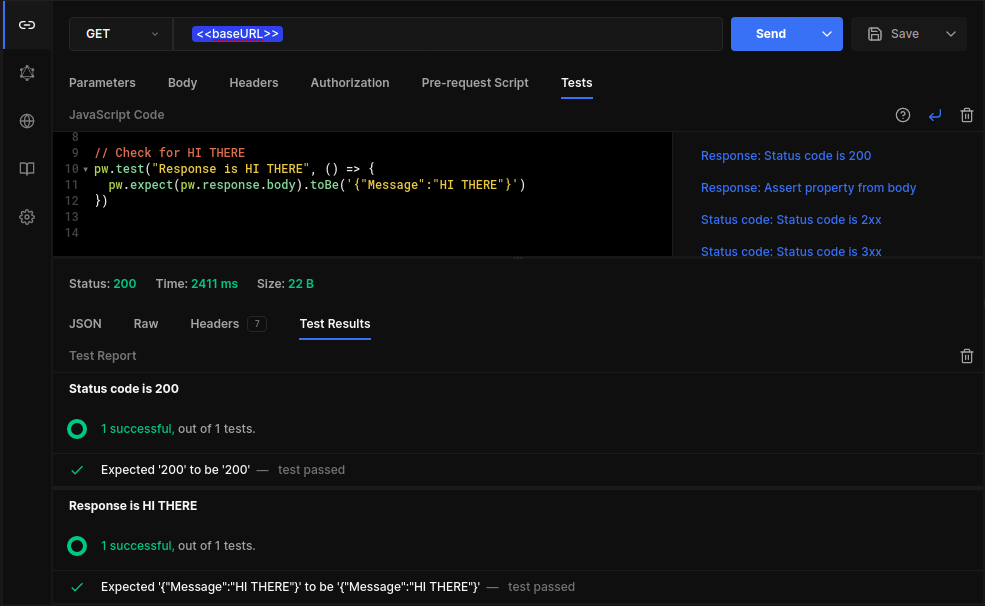
status code 200
and expected message
. Now it’s your assignment to add status code tests to all the HTTP requests we made today because Practice makes you perfect.Conclusion
Yep! We worked on the complete practical use of the Hopscotch HTTP Client. We learned how to make different HTTP Requests along with the 4 most common Request Methods - GET
, POST
, PUT
& DELETE
. We worked on how to organize all of our API requests using Collections
and creating & using variables by using Environments
.
Let’s connect
Thanks for reading, Peace and Bubbyeye, and yeah keep learning no matter how sleepy you are..!
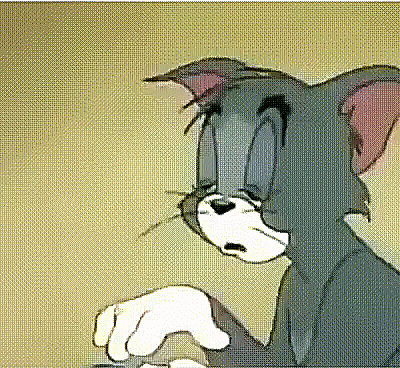
Sleepy Tom
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK