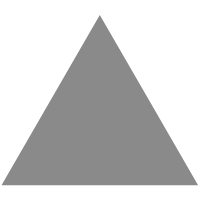
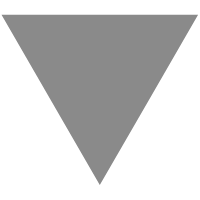
Python 内置函数
source link: https://www.biaodianfu.com/python-built-in-functions.html
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
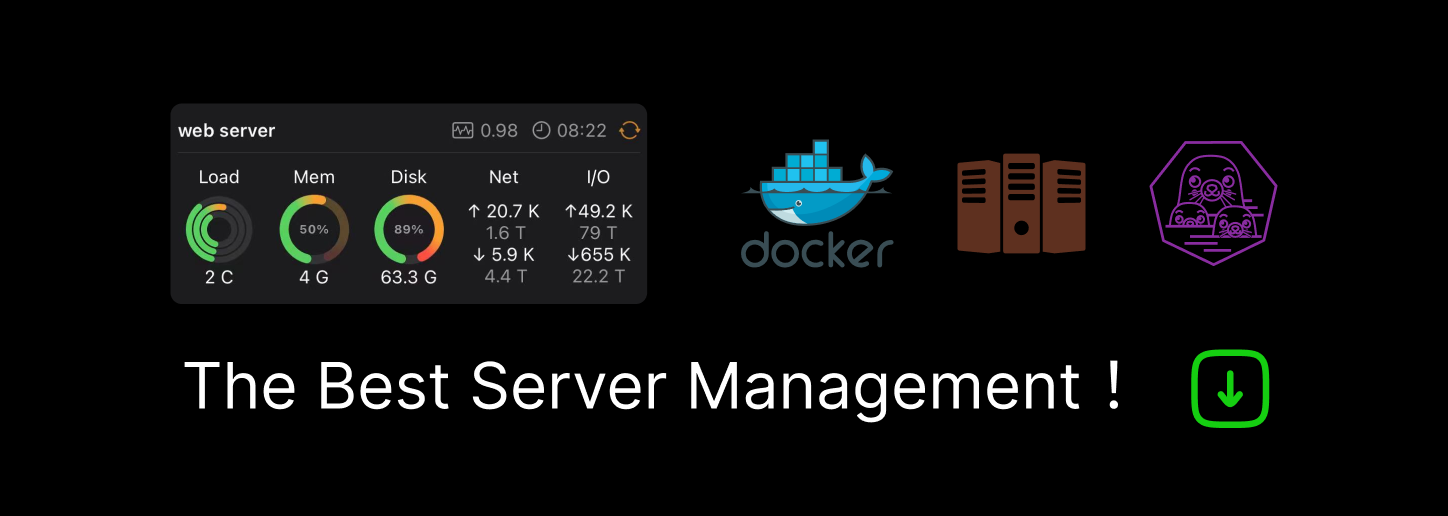
Python设计理念是“小的核心语言”+“大的标准库”,当Python想要添加新功能时,更多思考的是改将此特性加入核心语言支持还是作为扩展放入库中。Python 标准库非常庞大,包含了很多模块,要想使用某个函数,必须提前导入对应的模块,否则函数是无效的。而内置函数是解释器的一部分,它随着解释器的启动而生效。所以内置函数的数量必须被严格控制,否则 Python 解释器会变得庞大和臃肿。一般来说,只有那些使用频繁或者和语言本身绑定比较紧密的函数,才会被提升为内置函数。
目前Python提供的内置函数共79个:
abs() delattr() hash() memoryview() set() all() dict() help() min() setattr() any() dir() hex() next() slice() ascii() divmod() id() object() sorted() bin() enumerate() input() oct() staticmethod() bool() eval() int() open() str() breakpoint() exec() isinstance() ord() sum() bytearray() filter() issubclass() pow() super() bytes() float() iter() print() tuple() callable() format() len() property() type() chr() frozenset() list() range() vars() classmethod() getattr() locals() repr() zip() compile() globals() map() reversed() __import__() complex() hasattr() max() round()
以上的内容按照首字母排序,为了更好的了解和掌握这些知识,进行简单的分类,对一些比较难理解的进行重点分析。
- abs():返回绝对值
- divmod():返回商和余数
- max():返回最大值
- min():返回最小值
- pow():幂计算
- round():四舍五入数字
- sum():求和
- bool():返回Bool值
- int():返回整数
- float():返回浮点数
- dict():返回字典
- list():返回列表
- tuple():返回元组
- set():返回集合
- str():返回字符串
- complex():返回复数
- bytearray():返回字节数组
- bytes():返回不可变字节数组
- memoryview():根据传入参数创建一个新的内存查看对象
- ord():返回Unicode字符对应的整数
- chr():返回整数对应的Unicode字符
- bin():将整数转换成二进制字符串
- oct():将整数转化为8进制字符串
- hex():将整数转换成16进制字符串
- frozenset():根据传入参数创建一个不可变集合
- enumerate():根据可迭代对象创建枚举对象
- range():根据传入的参数创建一个新的range对象
- iter():根据传入的参数创建一个新的可迭代对象
- slice():根据传入的参数创建一个新的切片对象
- super():根据传入的参数创建一个新的子类和父类关系的代理对象
- object():创建一个新的object对象
enumerate()
seasons = ['Spring', 'Summer', 'Fall', 'Winter'] print(list(enumerate(seasons))) print(list(enumerate(seasons, start=1))) # 指定起始值
super()
super()函数用于提供对父类或兄弟类的方法和属性的访问。super()函数返回一个代表父类的对象。
class Parent: def __init__(self, txt): self.message = txt def print_message(self): print(self.message) class Child(Parent): def __init__(self, txt): super().__init__(txt) x = Child("Hello, and welcome!") x.print_message()
- all():判断每个元素是否为True
- any():判断是否存在True的元素
- filter():使用指定方法进行元素过滤
- map():使用指定方法去作用传入的每个可迭代对象的元素,生成新的可迭代对象
- next():返回可迭代对象中的下一个元素值
- reversed():反转序列生成新的可迭代对象
- sorted():对可迭代对象进行排序,返回一个新的列表
- zip():聚合传入的每个迭代器中相同位置的元素,返回一个新的元组类型迭代器
filter()
def if_odd(x): # 定义奇数判断函数 return x % 2 == 1 a = list(range(1, 10)) # 定义序列 print(list(filter(if_odd, a))) # 筛选序列中的奇数 # [1, 3, 5, 7, 9]
map()
a = map(ord, 'abcd') print(list(a)) # [97, 98, 99, 100]
zip()
x = [1, 2, 3] # 长度3 y = [4, 5, 6, 7, 8] # 长度5 print(list(zip(x, y))) # 取最小长度3 # [(1, 4), (2, 5), (3, 6)]
- help():返回对象的帮助信息
- dir():返回对象或者当前作用域内的属性列表
- id():返回对象的唯一标识符
- hash():获取对象的哈希值
- type():type:返回对象的类型
- len():返回对象的长度
- ascii():返回对象的可打印表字符串表现方式
- format():格式化显示值
dir()
import math print(dir(math)) # ['__doc__', '__loader__', '__name__', '__package__', '__spec__', 'acos', 'acosh', 'asin', 'asinh', 'atan', 'atan2', 'atanh', 'ceil', 'copysign', 'cos', 'cosh', 'degrees', 'e', 'erf', 'erfc', 'exp', 'expm1', 'fabs', 'factorial', 'floor', 'fmod', 'frexp', 'fsum', 'gamma', 'gcd', 'hypot', 'inf', 'isclose', 'isfinite', 'isinf', 'isnan', 'ldexp', 'lgamma', 'log', 'log10', 'log1p', 'log2', 'modf', 'nan', 'pi', 'pow', 'radians', 'remainder', 'sin', 'sinh', 'sqrt', 'tan', 'tanh', 'tau', 'trunc']
format()
- __import__():
- isinstance():判断对象是否是类或者类型元组中任意类元素的实例
- issubclass():判断类是否是另外一个类或者类型元组中任意类元素的子类
- hasattr():检查对象是否含有属性
- getattr():获取对象的属性值
- setattr():设置对象的属性值
- delattr():删除对象的属性
- callable():检测对象是否可被调用
- globals():返回当前作用域内的全局变量和其值组成的字典
- locals():返回当前作用域内的局部变量和其值组成的字典
- vars():返回当前作用域内的局部变量和其值组成的字典,或者返回对象的属性列表
- print():向标准输出对象打印输出
- input():读取用户输入值
- open():使用指定的模式和编码打开文件,返回文件读写对象
- compile():将字符串编译为代码或者AST对象,使之能够通过exec语句来执行或者eval进行求值
- eval():执行动态表达式求值
- exec():执行动态语句块
- repr():返回一个对象的字符串表现形式(给解释器)
- @property:标示属性的装饰器
- @classmethod:标示方法为类方法的装饰器
- @staticmethod:标示方法为静态方法的装饰器
- breakpoint()
参考链接:
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK