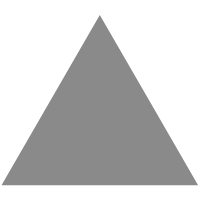
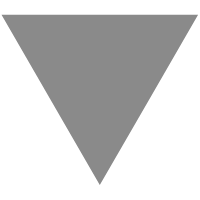
A Beginner's Guide to Using Interfaces in Java
source link: https://www.makeuseof.com/using-interfaces-java/
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
A Beginner's Guide to Using Interfaces in Java
Published 19 hours ago
With interfaces in Java, you can add multiple inheritances. Here's a beginner's guide to using them.
An interface is a reference type that is used to enforce a contract with a class. A contract refers to an obligation to implement the methods that an interface defines.
Interfaces provide an abstraction between the methods they define & how the user implements them in a class. A practical use case of this is in APIs (Application Programming Interfaces).
APIs allow your program to communicate with other programs without knowing how they are implemented. This is important for both proprietary reasons (for the company that owns the rights) and for easy development on your part.
Let's take a look at how to use Java interfaces.
Defining Interfaces
To declare an interface, place the keyword interface before the interface name.
interface Car{
// constant declarations, if any
int tyres = 4;
// method signatures
int lights (int brightness);
int turn (int tyres, String direction){
// some code
}
}
In your interface header, you can also include its level of visibility before the keyword interface.
The values in an interface are constant. These values are by default public, static and final. Therefore, there's no need to use these keywords while declaring values in the body of an interface.
Related: How to Manage Variable Scope in Java
An interface's body can also have default, abstract, static methods. These methods are by default public, so there's no need to indicate these access modifiers when declaring them.
Abstract methods are declared by leaving out the curly brackets of a method's body. See line 7 in the code above. Static methods are declared by proceeding the method name with the keyword static & default methods are declared with the default modifier.
Now would be a good time to mention that you must use methods declared in an interface in any classes that implement it. Not doing so will make the compiler "enforce the contract" by giving a compilation error.
This particular property of interfaces may have some drawbacks. Consider a case where an application programming interface (API) provider decides to add more methods to their interfaces, but several apps are based on the old interfaces. Developers using the old interfaces in their programs would have to rewrite their code, which is not practical.
So, that's where default methods come in. They enable API providers to add more methods to their interfaces while ensuring binary compatibility with older interface versions.
default int getDirection ( String coordinates){
// write some code to give a default implementation
}
The above method shows how a default method called getDirection is declared. Notice that you have to include an implementation of a default method when you write it.
Using Interfaces
Now we've defined interfaces in Java, we can move on to how you can implement them. You'll find this out in the section below.
Implementing Interfaces
To implement an interface, use the keyword implements using this syntax:
class A implements interface P{
}
Remember that you must use all the interface methods in the class. You can ignore this rule only if one of the methods is defined as default in the interface.
Related: An Introduction to Using Linked Lists in Java
If you want your class to implement multiple interfaces, you can separate them using commas in your header declaration.
Example:
class A implements interface P, Q, R{
}
If the class that's implementing the interface is a subclass, use the syntax below:
class A extends B implements C, D{
}
Interfaces enable multiple inheritances in Java. Ordinarily, a class can only extend one class (single inheritance). Interfaces are the only way that Java can carry out multiple inheritances.
Interfaces can also extend other interfaces, just like a class can extend another class. The child interface inherits the methods of the interface it extends.
See the example below:
interface A extends B{
}
Other than using default methods to modify an interface without requiring the developers to modify their current programs, you can also extend the existing interfaces.
Now You’ve Got Some Basic Knowledge About Java Interfaces
Interfaces in Java demonstrate abstraction, one of the four pillars of object-oriented programming. Polymorphism is one of those four pillars. It refers to the ability of a method to take on many forms.
You can implement polymorphism in Java through method overloading or method overriding. Next on your Java reading list should be how to implement these functions.
About The Author
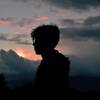
Jerome Davidson (25 Articles Published)
Jerome is a Staff Writer at MakeUseOf. He covers articles on Programming and Linux. He's also a crypto enthusiast and is always keeps tabs on the crypto industry.
Subscribe to our newsletter
Join our newsletter for tech tips, reviews, free ebooks, and exclusive deals!
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK