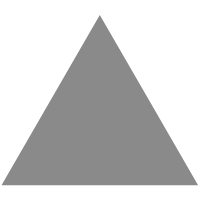
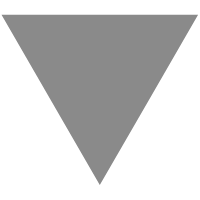
An Introduction to Arrays in C
source link: https://www.makeuseof.com/arrays-in-c-introduction/
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
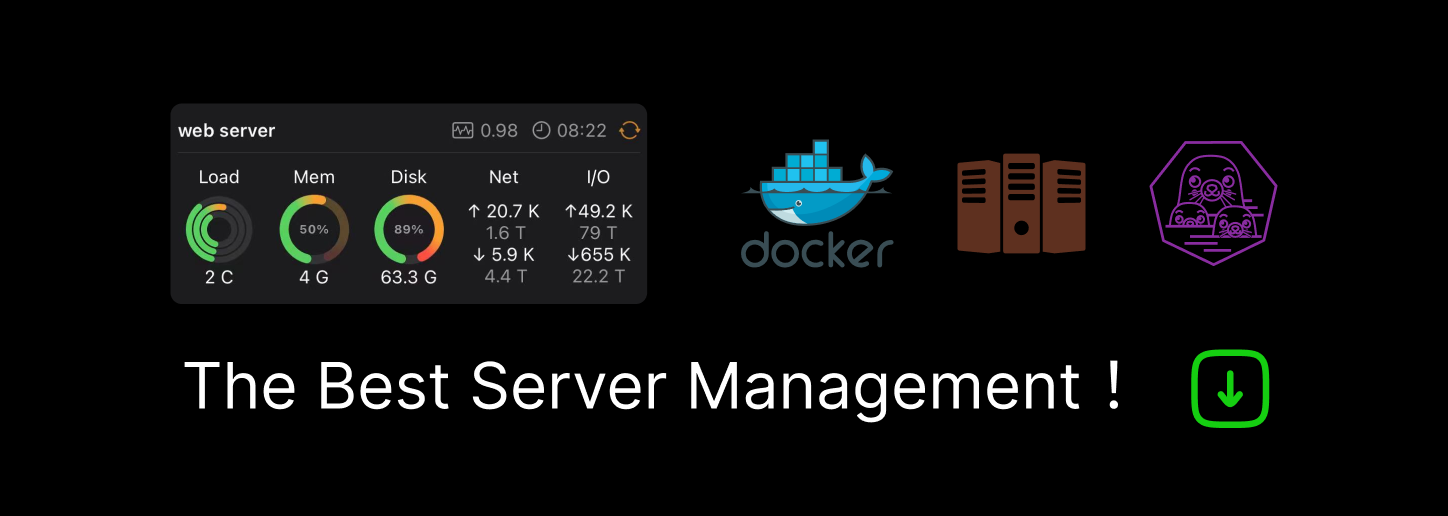
An Introduction to Arrays in C
Published 7 hours ago
C is sometimes challenging to learn, and many beginner programmers get mixed up when it comes to arrays. Here's a simple tutorial to help you.
An array is a data structure used to store sequential items of the same data type. The position of an element in the sequence is called an index. Indexes begin from 0 to (n-1).
In this article, you will learn how to use arrays in C. Most of the concepts here cut across to most other programming languages, so be sure to take note of them.
Defining Arrays
To define an array, write its data type followed by the array name and square brackets:
int age[8];
Inside the square brackets is the expected number of data items that the array will receive. If you need to declare two arrays at the same time, you can do so as below. It's worth mentioning that declaring them separately is the preferred way.
int age[8], height[8];
It's also possible to declare an array as below:
int[8] age;
The above syntax would mean that age is a pointer to an array of type int. Though you can use the "pointer syntax", the first one is preferred.
Related: How to Display the Multiplication Table of a Number Using Python, C++, JavaScript, and C
Sometimes, you may not know how many elements you expect your array to have. In such a case, you would have to declare the array without the number of elements. See the example below:
int age[];
Operations on Arrays
To reference an array element, write the array name followed by its index in square brackets (e.g. age[5]). You can also use variables inside the square brackets:
age[x+y]
You need to ensure that the computation of these variables is in the range 0 to (n-1). Otherwise, you'll get a compilation error.
Initialization
Array elements can be given at declaration or later on in the program. This action is known as initialization since the array initially has null values.
See the example below. It prints an element's value and its index in the array. Line 5 uses an initializer list to initialize the integer array.
#include <stdio.h>
int main(void) {
printf("%s%11s
", "Element", "Value");
int n[4] = {1, 4, 9, 16};
// output array elements in tabular format
for (size_t i = 0; i < 4; ++i) {
printf("%5u%10d
", i, n[i]);
}
}
Output :
Element Value
0 1
1 4
2 9
3 16
Using Arrays to Store Strings
Arrays can also be used to store strings, not just integers. When used this way, remember to include single quotes for each element in the initializer list.
char fruit[]= ['b', 'e', 'r', 'r', 'y', '\0' ];
Notice that the char data type is used. A string literal actually consists of individual characters, and that's why you see char.
Related: How to Learn C++ Programming: 6 Sites to Get Started
The array shown ends with an escape sequence (\0). This is a string-termination character called the null character. You must always include this when initializing an array list.
There's actually a simpler way to initialize a character array. We showed the former approach first so you know that a character array always has a special ending character (\0). It's a common error for beginner programmers to have the array size less by one, forgetting the last element(\0).
char string1[5] = "Code";
Suppose you need to get user input and store it in an array. You can use the standard library function scanf for this.
You need to specify the number of characters the function should expect, though. This is because scanf doesn't check the array size and may write data to addresses beyond the array, causing a buffer overflow.
Use the %s conversion specifier to define the maximum expected input. The scanf function will read all characters entered until space, tab, newline, or an end-of-file indicator is encountered.
Advancing Your C Programming
The resources you use to learn are just as crucial as your zeal to learn. Learning without good practice will make your programming journey challenging.
C is a particularly challenging language to learn since it's not object-oriented. Luckily, we've got plenty of resources to help you master C programming.
About The Author
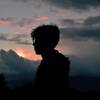
Jerome Davidson (23 Articles Published)
Jerome is a Staff Writer at MakeUseOf. He covers articles on Programming and Linux. He's also a crypto enthusiast and is always keeps tabs on the crypto industry.
Subscribe to our newsletter
Join our newsletter for tech tips, reviews, free ebooks, and exclusive deals!
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK