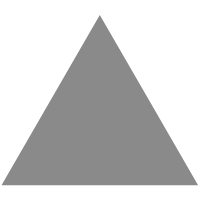
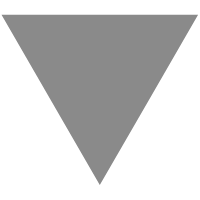
Python typeerror: ‘str’ object is not callable Solution
source link: https://dev.to/itsmycode/python-typeerror-str-object-is-not-callable-solution-5f0d
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
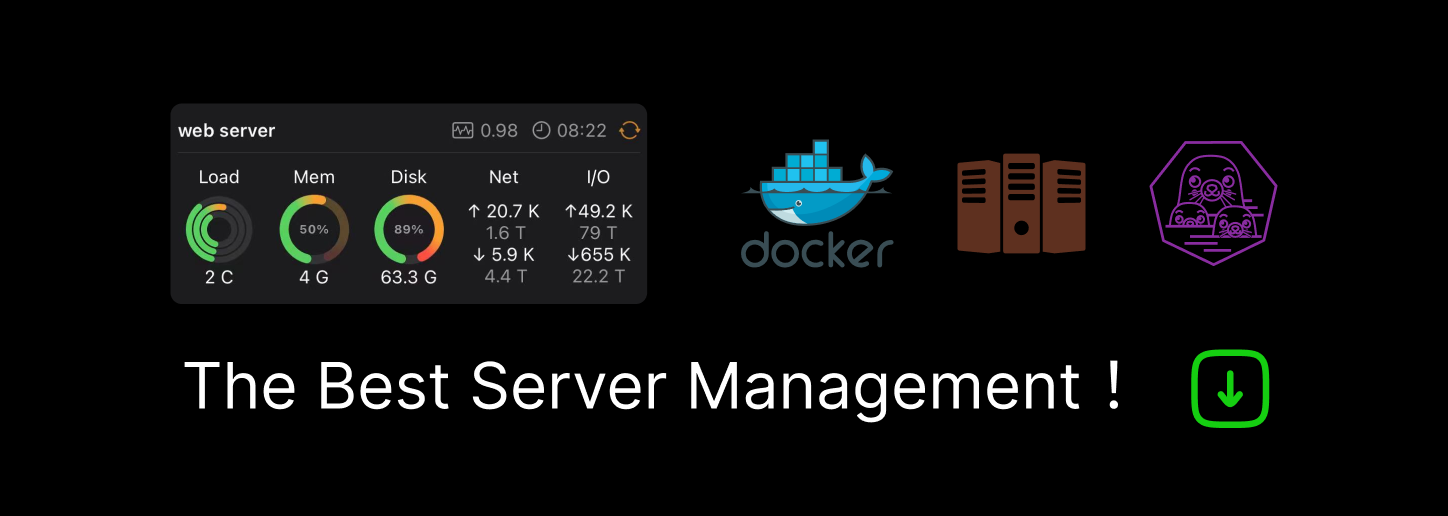
ItsMyCode |
One of the most common errors in Python programming is typeerror: ‘str’ object is not callable , and sometimes it will be painful to debug or find why this issue appeared in the first place.
What is typeerror: ‘str’ object is not callable in Python?
Python has a built-in method str()
which converts a specified value into a string. The str()
method takes an object as an argument and converts it into a string.
Since str()
is a predefined function and a built-in reserved keyword in Python, you cannot use it in declaring it as a variable name or a function name. If you do so, Python will throw a typeerror: ‘ str ‘ object is not callable.
Let us take a look at few scenarios where you could reproduce this error.
Scenario 1 – Declaring a variable name called “str”
The most common scenario and a mistake made by developers are declaring a variable named ‘ str
‘ and accessing it. Let’s look at a few examples of how to reproduce the ‘str’ object is not callable error.
str = "Hello, "
text = " Welcome to ItsMyCode"
print(str(str + text))
# Output
Traceback (most recent call last):
File "c:\Projects\Tryouts\listindexerror.py", line 4, in <module>
print(str(str + text))
TypeError: 'str' object is not callable
In this example, we have declared ‘ str
‘ as a variable, and we are also using the predefined str()
method to concatenate the string.
str = "The cost of apple is "
x = 200
price= str(x)
print((str + price))
# output
Traceback (most recent call last):
File "c:\Projects\Tryouts\listindexerror.py", line 3, in <module>
price= str(x)
TypeError: 'str' object is not callable
The above code is similar to example 1, where we try to convert integer x into a string. Since str
is declared as a variable and if you str()
method to convert into a string, you will get object not callable error.
Solving typeerror: ‘str’ object is not callable in Python.
Now the solution for both the above examples is straightforward; instead of declaring a variable name like “ str ” and using it as a function, declare a more meaningful name as shown below and make sure that you don’t have “ str ” as a variable name in your code.
text1 = "Hello, "
text2 = " Welcome to ItsMyCode"
print(str(text1 + text2))
# Output
Hello, Welcome to ItsMyCode
text = "The cost of apple is "
x = 200
price= str(x)
print((text + price))
# Output
The cost of apple is 200
Scenario 2 – String Formatting Using %
Another hard-to-spot error that you can come across is missing the *%
* character in an attempt to append values during string formatting.
If you look at the below code, we have forgotten the string formatting %
to separate our string and the values we want to concatenate into our final string.
print("Hello %s its %s day"("World","a beautiful"))
# Output
Traceback (most recent call last):
File "c:\Projects\Tryouts\listindexerror.py", line 1, in <module>
print("Hello %s its %s day"("World","a beautiful"))
TypeError: 'str' object is not callable
print("Hello %s its %s day"%("World","a beautiful"))
# Output
Hello World its a beautiful day
In order to resolve the issue, add the %
operator before replacing the values ("World","a beautiful")
as shown above.
The post Python typeerror: ‘str’ object is not callable Solution appeared first on ItsMyCode.
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK