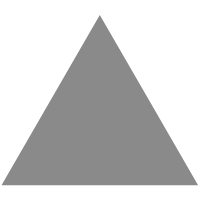
5
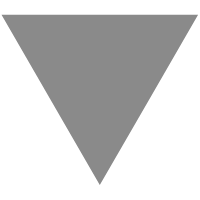
设计模式--组合(Component)模式
source link: https://segmentfault.com/a/1190000040406665
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
将对象组合成树形结构以表示“部分--整体”的层次结构,Composite使得用户对单个对象和组合对象的使用具有一致性(稳定)
- Composite模式采用树形结构来实现普遍存在的对象容器,从而将“一对多”的关系转化为“一对一”的关系,使得客户代码可以一致地(复用)处理对象和对象容器,无需关系处理的是单个的对象,还是组合的对象容器
- 将“客户代码与复杂的对象容器结构”解耦是Composite的核心思想解耦之后,客户代码将于纯粹的抽象接口--而给对象容器的内部实现结构--发生依赖,从而更能“应对变化”
- Composite模式在具体实现中,可以让父对象中的子对象反向追溯,如果父对象有频繁的遍历需求,可使用缓存技巧来改善效率
Go语言代码实现
composite.go
package Composite
import "fmt"
type Component interface {
Traverse()
}
type Leaf struct {
value int
}
func NewLeaf (value int) *Leaf{
return &Leaf{value: value}
}
func (l *Leaf) Traverse() {
fmt.Println(l.value)
}
type Composite struct {
children []Component
}
func NewComposite() * Composite{
return &Composite{children: make([]Component, 0)}
}
func (c *Composite) Add (component Component) {
c.children = append(c.children, component)
}
func (c *Composite) Traverse() {
for idx, _ := range c.children{
c.children[idx].Traverse()
}
}
composite_test.go
package Composite
import (
"fmt"
"testing"
)
func TestComposite_Traverse(t *testing.T) {
containers := make([]Composite, 4)
for i := 0; i< 4; i++ {
for j := 0; j < 3 ; j++ {
containers[i].Add(NewLeaf(i * 3 + j))
}
}
for i := 0; i < 4; i++ {
containers[0].Add(&containers[i])
}
for i := 0; i < 4; i++{
containers[i].Traverse()
fmt.Printf("Finished\n")
}
}
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK