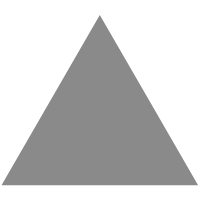
5
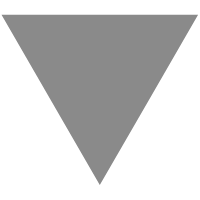
设计模式--责任链(Responsibility_Chain)模式
source link: https://segmentfault.com/a/1190000040406679
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
使多个对象都有机会处理请求,从而避免请求的发送者和接受者之间耦合关系,将这些对象连成一条链,并沿着这条链传递请求,直到有一个对象处理它为止
- Chain of Responsibility模式的应用场合在于“一个请求可能有多个接受者,但是最后真正的接受者只有一个”,这时候请求发送者与接受者的耦合有可能出现“变化脆弱”的症状,职责链的目的就是将二者解耦,从而更好地应对变化
- 应用了Chain of Responsibility模式后,对象的职责分配将更具灵活性,我们可以在运行时动态添加、修改请求的处理职责
- 如果请求传递到职责链的末尾任得不到处理,应该有一个合理的缺省机制,这也是每一个接受者对象的责任,而不是发出请求的对象的责任
Go语言代码实现
responsibility_chain.go
package Responsibility_Chain
import "strconv"
type Handler interface {
Handler(handlerID int) string
}
type handler struct {
name string
next Handler
handlerID int
}
func NewHandler(name string, next Handler, handlerID int) *handler{
return &handler{
name: name,
next: next,
handlerID: handlerID,
}
}
func (h *handler) Handler(handlerID int) string{
if h.handlerID == handlerID{
return h.name + " handled " + strconv.Itoa(handlerID)
}
return h.next.Handler(handlerID)
}
responsibility_chain_test.go
package Responsibility_Chain
import (
"fmt"
"testing"
)
func TestNewHandler(t *testing.T) {
wang := NewHandler("laowang", nil, 1)
zhang := NewHandler("lanzhang", wang, 2)
r := wang.Handler(1)
fmt.Println(r)
r = zhang.Handler(2)
fmt.Println(r)
}
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK