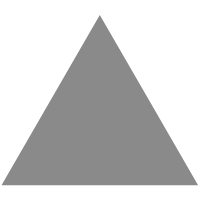
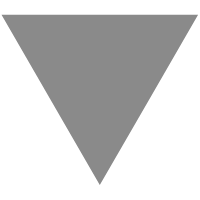
Produce By Path - New JavaScript Design Pattern
source link: https://dev.to/rubenarushanyan/produce-by-path-new-javascript-design-pattern-8in
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
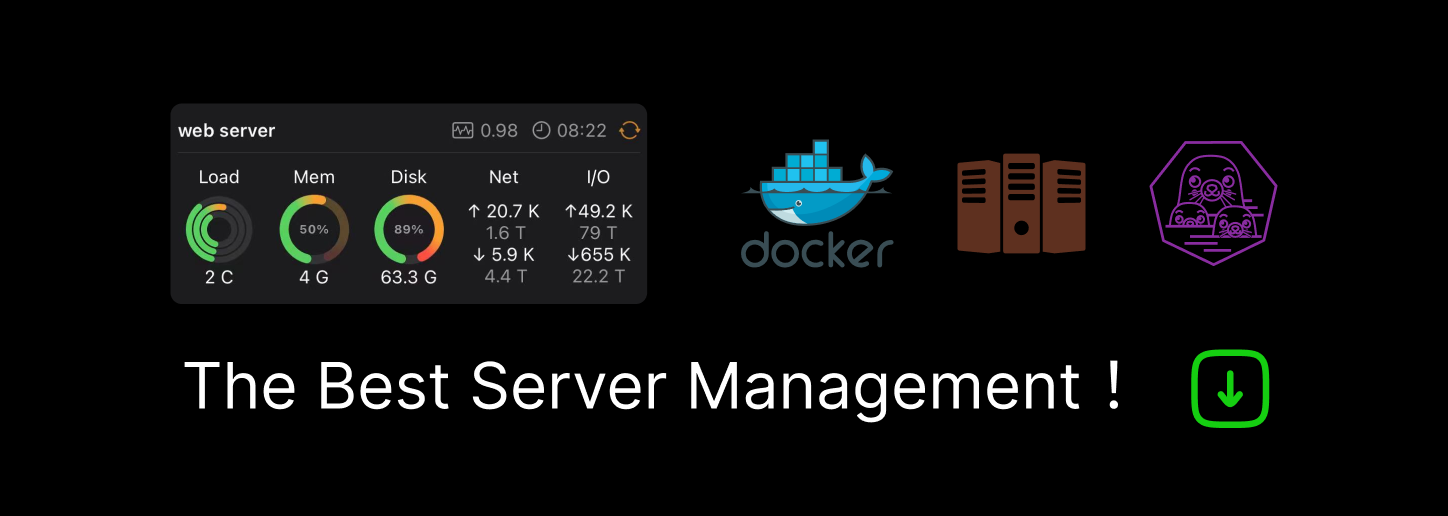
Produce By Path - New JavaScript Design Pattern
Jul 11
・2 min read
Description
Produce By Path is a design pattern, which is used to dynamically produce values by using the path to which it is applied.
Installation
npm install produce-by-path
Usage
import ProduceByPath from "produce-by-path"
// CommonJS usage
// const ProduceByPath = require("produce-by-path")
// define producer instance to our liking :)
const instance = new ProduceByPath({
call: (path, args) => {
return ({
path,
args,
})
},
toPrimitive: (path, hint) => {
return path.join("--")
}
})
// Now we can apply the [[instance]] object with any properties
// combination and call as a function and receive the desired
// result as we defined in the [[call]] handler.
console.log( instance.I.love.you("arg1", "arg2") )
// {
// path: ["I", "love", "you"],
// args: ["arg1", "arg2"]
// }
// We can also apply the [[instance]] object with any properties
// combination and convert as a primitive value and receive
// the desired result as we defined in [[toPrimitive]] handler.
console.log( String(instance.I.love.you) )
// I--love--you
console.log( instance.I.love.you + '')
// I--love--you
Why should I use an ProduceByPath pattern?
Using that pattern in some cases we can build a simple and intuitive interface for my software.
For example, the redux-cool library use that pattern for making action objects.
import {actionsCreator} from "redux-cool"
const first_action = actionsCreator.MY.FIRST.ACTION("arg1", "arg2")
console.log(first_action)
// {
// type: "MY/FIRST/ACTION",
// args: ["arg1", "arg2"],
// cb: f() identity,
// _index: 1
// }
const second_action = actionsCreator.This.is.my.second.action(2021)
console.log(second_action)
// {
// type: "This/is/my/second/action",
// args: [2021],
// cb: f() identity,
// _index: 2
// }
// If we just need to generate an action type as a string,
// we can do it easily
const type1 = String(actionsCreator.MY.FIRST.ACTION)
console.log(type1)
// "MY/FIRST/ACTION"
// or any string conversion
const type2 = actionsCreator.MY.FIRST.ACTION + ""
console.log(type2)
// "MY/FIRST/ACTION"
Links
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK