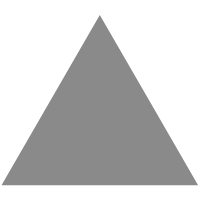
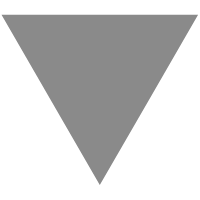
Pretty Output Format in C++
source link: https://jdhao.github.io/2021/07/04/pretty_fmt_in_cpp/
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
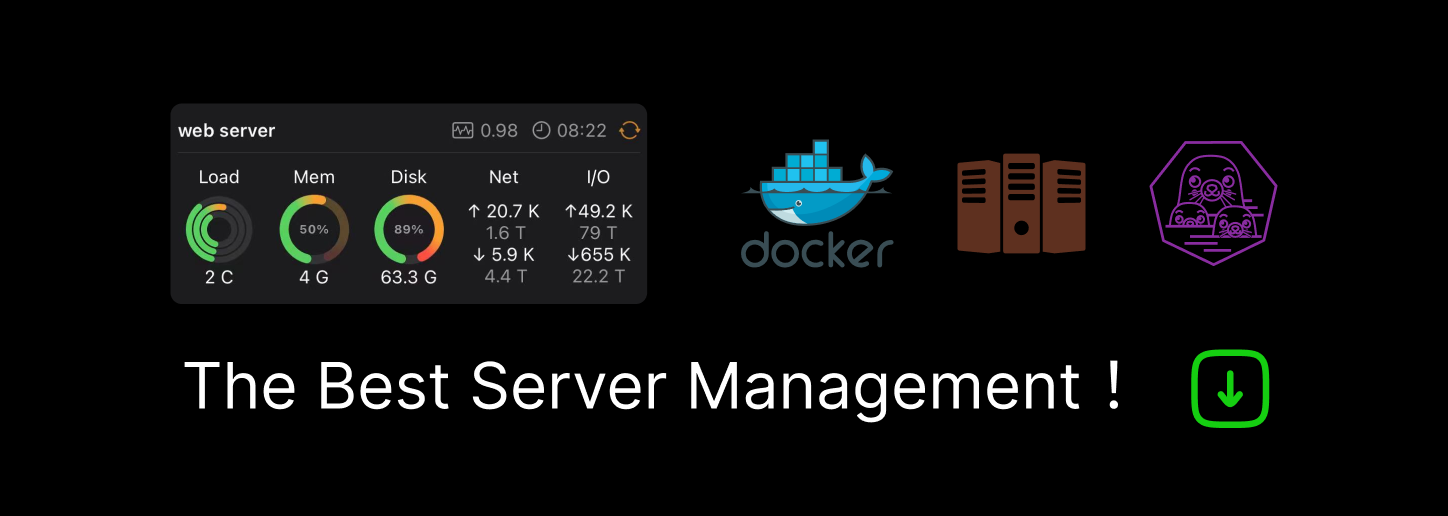
Pretty Output Format in C++
Although std::cout
in C++ is powerful, it lacks the ease of use of format
string in Python. Fortunately, the 3rd party package fmt
provides a similar feature for C++.
Build fmt
To use the fmt package, we need to build it:
git clone --depth 1 https://github.com/fmtlib/fmt.git
cd fmt
mkdir build && cd build
cmake -G "Unix Makefiles" -DCMAKE_INSTALL_PREFIX=~/tools/fmt -DCMAKE_BUILD_TYPE=Release ..
make -j 8
make install
This will build the static version of fmt and install it under
$HOME/tools/fmt
.
To build a dynamic library, use the following cmake command instead:
cmake -G "Unix Makefiles" -DCMAKE_INSTALL_PREFIX=~/tools/fmt -DCMAKE_BUILD_TYPE=Release -DBUILD_SHARED_LIBS=TRUE ..
How to use
Here is a short snippet to test it:
#include <iostream>
#include <vector>
#include <fmt/ranges.h>
#include <fmt/core.h>
using std::vector;
int main() {
vector<int> arr = {1, 2, 3};
fmt::print("arr is {}", arr);
fmt::print("The price of {} is {} Yuan/kg", "apple", 4.2);
return 0;
}
Use the following comamnd to build against the static version of fmt:
g++ -L$HOME/tools/fmt/lib64/ -I$HOME/tools/fmt/include/ test.cc -l:libfmt.a -o test_static
And the following command to build against the dynamic version of fmt:
g++ -L$HOME/tools/fmt/lib64/ -I$HOME/tools/fmt/include/ test.cc -lfmt -o test_shared
To run the dynamic executable, you need to set up the env variable
LD_LIBRARY_PATH
properly:
LD_LIBRARY_PATH=$HOME/tools/fmt/lib64:$LD_LIBRARY_PATH ./test_shared
The size differs significantly between the static and dynamic executable files:
32K test_shared
220K test_static
References
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK