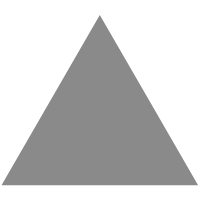
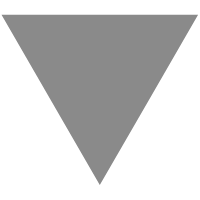
C++ Program for Matrix manipulation (menu based) by using Multi Dimensional Arra...
source link: https://sqlwithmanoj.com/2010/01/06/c-program-for-matrix-manipulation-menu-based-by-using-multi-dimensional-array-and-switch-case-q6/
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
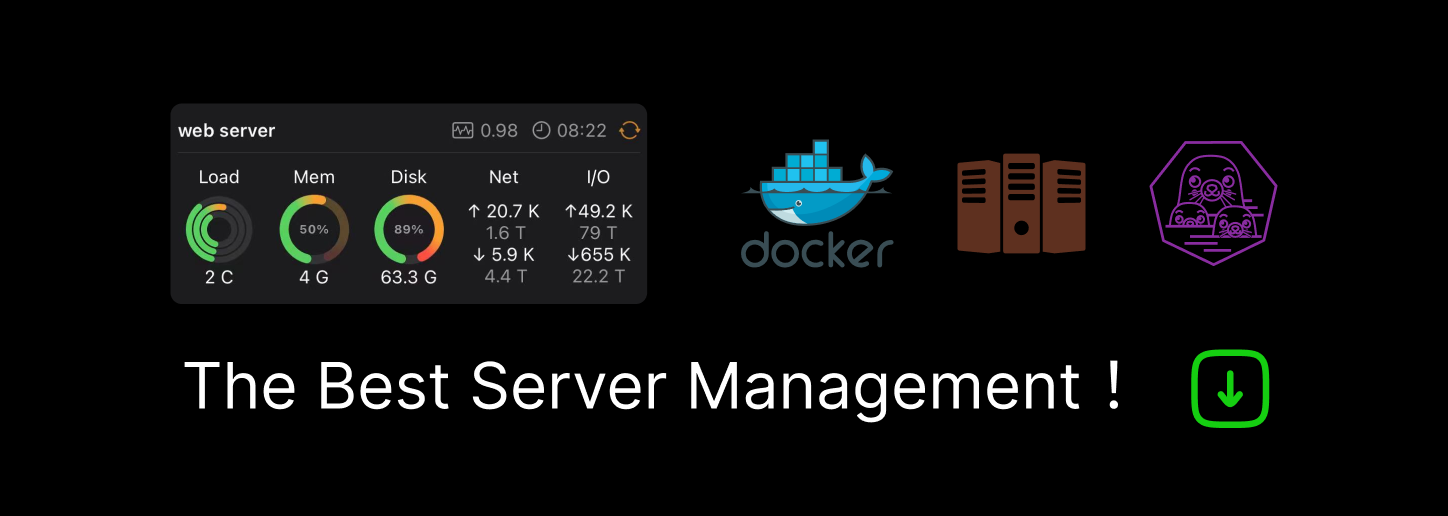
C++ Program for Matrix manipulation (menu based) by using Multi Dimensional Array and SWITCH CASE – Q6
Q6. Program Matrix manipulation (menu based):
for following Matrix manipulations:
i) Add two matrices
ii) Multiply two matrices
iii) Find the difference
iv) Find the sum of Rows and Sum of Cols.
… from College notes (BCA/MCA assignments):
#include <iostream.h>
#include <conio.h>
#include <stdlib.h>
void
Init_Matrix (
int
[][3],
int
[][3]);
void
Disp_Matrix (
int
[][3],
int
[][3]);
void
Add_Matrix (
int
[][3],
int
[][3]);
void
Sub_Matrix (
int
[][3],
int
[][3]);
void
Mult_Matrix (
int
[][3],
int
[][3]);
void
Sum_R_C (
int
[][3],
int
[][3]);
void
main(){
int
mat1[3][3], mat2[3][3];
int
ch;
clrscr();
cout<<
"\n Matrix Operation of Order (3*3):- \n"
;
Init_Matrix(mat1, mat2);
while
(1){
clrscr();
Disp_Matrix(mat1, mat2);
cout<<
"\n Matrix Operation of Order (3*3):- \n"
;
cout<<
"\n 1 -> Change Elements of Matrices."
;
cout<<
"\n 2 -> Add Matrices."
;
cout<<
"\n 3 -> Subtract Matrices."
;
cout<<
"\n 4 -> Multiply Matrices."
;
cout<<
"\n 5 -> Sum of ROWs n COLs."
;
cout<<
"\n 6 -> Exit."
;
cout<<
"\n Enter your choice: "
;
cin>>ch;
switch
(ch){
case
1:
Init_Matrix(mat1, mat2);
break
;
case
2:
Add_Matrix(mat1, mat2);
break
;
case
3:
Sub_Matrix(mat1, mat2);
break
;
case
4:
Mult_Matrix(mat1, mat2);
break
;
case
5:
Sum_R_C(mat1, mat2);
break
;
case
6:
exit
(1);
default
:
cout<<
"\n Enter Correct Choice."
;
}
// end of switch.
getch();
}
// end of while.
}
// end of main.
void
Init_Matrix(
int
mat1[][3],
int
mat2[][3]){
cout<<
"\n Enter the Elments of First Matrix: "
;
for
(
int
i=0; i<3; i++)
for
(
int
j=0; j<3; j++)
cin>>mat1[i][j];
cout<<
"\n Enter the Elments of First Matrix: "
;
for
(i=0; i<3; i++)
for
(j=0; j<3; j++)
cin>>mat2[i][j];
}
void
Disp_Matrix(
int
mat1[][3],
int
mat2[][3]){
cout<<
"\n First matrix is:- \n"
;
for
(
int
i=0; i<3; i++){
for
(
int
j=0; j<3; j++)
cout<<
" "
<<mat1[i][j];
cout<<endl;
}
cout<<
"\n Second matrix is:- \n"
;
for
(i=0; i<3; i++){
for
(
int
j=0; j<3; j++)
cout<<
" "
<<mat2[i][j];
cout<<endl;
}
}
void
Add_Matrix(
int
mat1[][3],
int
mat2[][3]){
int
tot[3][3];
for
(
int
i=0; i<3; i++)
for
(
int
j=0; j<3; j++)
tot[i][j] = mat1[i][j] + mat2[i][j];
cout<<
"\n Addition of 2 Matrices:- \n"
;
for
(i=0; i<3; i++){
for
(
int
j=0; j<3; j++)
cout<<
" "
<<tot[i][j];
cout<<endl;
}
}
void
Sub_Matrix(
int
mat1[][3],
int
mat2[][3]){
int
tot[3][3];
for
(
int
i=0; i<3; i++)
for
(
int
j=0; j<3; j++)
tot[i][j] = mat1[i][j] - mat2[i][j];
cout<<
"\n Subtraction of 2 Matrices:- \n"
;
for
(i=0; i<3; i++){
for
(
int
j=0; j<3; j++)
cout<<
" "
<<tot[i][j];
cout<<endl;
}
}
void
Mult_Matrix(
int
mat1[][3],
int
mat2[][3]){
int
tot[3][3];
for
(
int
i=0; i<3; i++)
for
(
int
j=0; j<3; j++){
tot[i][j]=0;
for
(
int
k=0; k<3; k++)
tot[i][j] = tot[i][j] +
(mat1[i][k]*mat2[k][j]);
}
cout<<
"\n Multiplication of 2 Matrices:- \n"
;
for
(i=0; i<3; i++){
for
(
int
j=0; j<3; j++)
cout<<
" "
<<tot[i][j];
cout<<endl;
}
}
void
Sum_R_C(
int
mat1[][3],
int
mat2[][3]){
int
row=0, col=0;
cout<<
"\n Sum of ROWs and COLs :- \n"
;
cout<<
"\n First Matrix :- \n"
;
// Sum of row of mat1.
for
(
int
i=0; i<3; i++){
for
(
int
j=0; j<3; j++){
cout<<
" "
<<mat1[i][j];
row = row + mat1[i][j];
}
cout<<
" - "
<<row;
row = 0;
cout<<endl;
}
cout<<
"\n | | | \n"
;
// Sum of col of mat1.
for
(i=0; i<3; i++){
for
(
int
j=0; j<3; j++)
col = col + mat1[j][i];
cout<<
" "
<<col;
col = 0;
}
cout<<
"\n\n Second Matrix :- \n"
;
// Sum of row of mat2.
for
(i=0; i<3; i++){
for
(
int
j=0; j<3; j++){
cout<<
" "
<<mat2[i][j];
row = row + mat2[i][j];
}
cout<<
" - "
<<row;
cout<<endl;
row = 0;
}
cout<<
"\n | | | \n"
;
// Sum of col of mat2.
for
(i=0; i<3; i++){
for
(
int
j=0; j<3; j++)
col = col + mat2[j][i];
cout<<
" "
<<col;
col = 0;
}
}
Output:
Matrix Operation of Order (3*3):-
Enter the Elments of First Matrix: 2 1 3 5 4 9 8 6 7
Enter the Elments of First Matrix: 3 1 4 2 5 6 9 8 0
First matrix is:-
2 1 3
5 4 9
8 6 7
Second matrix is:-
3 1 4
2 5 6
9 8 0
Matrix Operation of Order (3*3):-
1 -> Change Elements of Matrices.
2 -> Add Matrices.
3 -> Subtract Matrices.
4 -> Multiply Matrices.
5 -> Sum of ROWs n COLs.
6 -> Exit.
Enter your choice: 2
Addition of 2 Matrices:-
5 2 7
7 9 15
17 14 7
First matrix is:-
2 1 3
5 4 9
8 6 7
Second matrix is:-
3 1 4
2 5 6
9 8 0
Matrix Operation of Order (3*3):-
1 -> Change Elements of Matrices.
2 -> Add Matrices.
3 -> Subtract Matrices.
4 -> Multiply Matrices.
5 -> Sum of ROWs n COLs.
6 -> Exit.
Enter your choice: 3
Subtraction of 2 Matrices:-
-1 0 -1
3 -1 3
-1 -2 7
First matrix is:-
2 1 3
5 4 9
8 6 7
Second matrix is:-
3 1 4
2 5 6
9 8 0
Matrix Operation of Order (3*3):-
1 -> Change Elements of Matrices.
2 -> Add Matrices.
3 -> Subtract Matrices.
4 -> Multiply Matrices.
5 -> Sum of ROWs n COLs.
6 -> Exit.
Enter your choice: 4
Multiplication of 2 Matrices:-
35 31 14
104 97 44
99 94 68
1 -> Change Elements of Matrices.
2 -> Add Matrices.
3 -> Subtract Matrices.
4 -> Multiply Matrices.
5 -> Sum of ROWs n COLs.
6 -> Exit.
Enter your choice: 5
Sum of ROWs and COLs :-
First Matrix :-
2 1 3 – 6
5 4 9 – 18
8 6 7 – 21
| | |
15 11 19
Second Matrix :-
3 1 4 – 8
2 5 6 – 13
9 8 0 – 17
| | |
14 14 10
Related
Leave a Reply Cancel reply
This site uses Akismet to reduce spam. Learn how your comment data is processed.
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK