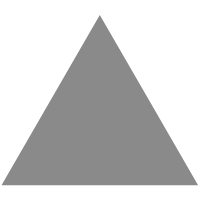
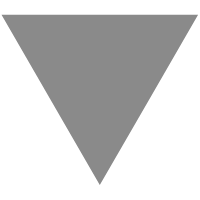
Introduction to Components in Angular
source link: https://blog.knoldus.com/introduction-to-components-in-angular/
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
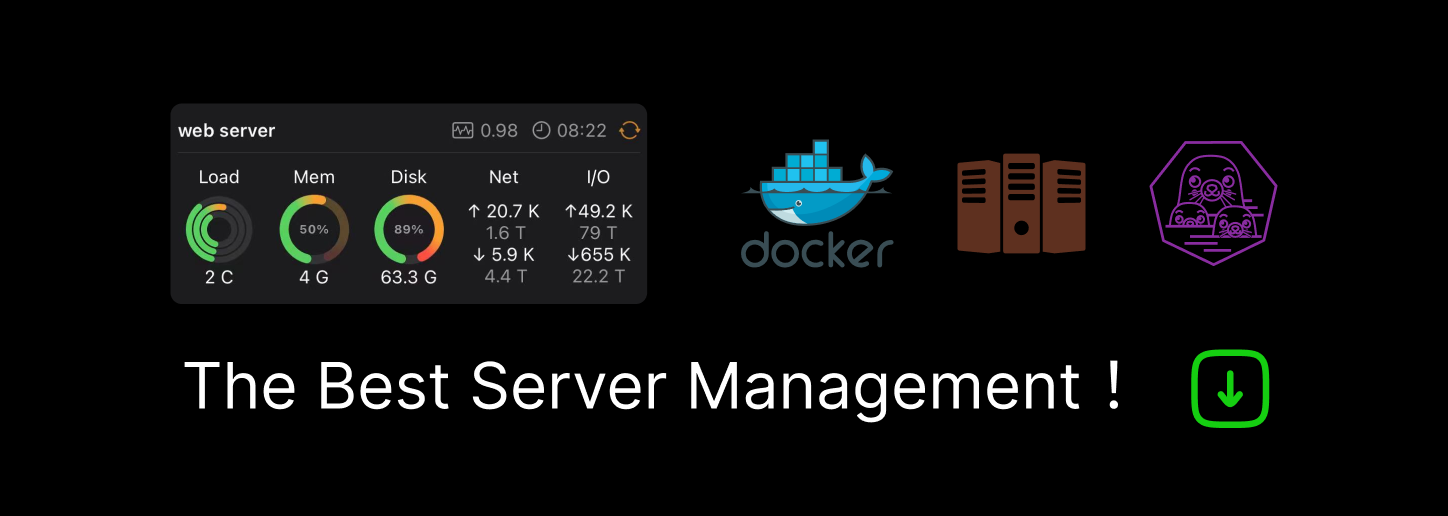
Introduction
In Angular, the whole view of the application is defined by using different components. Components are the basic building blocks of your application. By creating these components you are building an application that will have reusable parts. A component contains both your methods and properties bound together with the view(UI) defined. Let us now understand how we create these components and how to use them.
Components
Components are the main building blocks of your application. Each component in angular has the following files:
- An HTML template: This file defines how it will be rendered on the web page
- A CSS selector: It defines how the component is used in the template.
- CSS styles: It defines all the CSS styles which will be applied to your component.
- Typescript class: It defines the behavior.
The HTML template is basically the view of the component. All the html content is included in this file. Whereas all the css content is placed in the CSS styles. We also have a CSS selector, which is used when we want to use the component. It is used as a custom HTML tag. All the functions related to the functionalities of the component are included in the typescript class.
Creating a Component
There are two ways to create a component. Let us have a look:
1) Creating a component using angular CLI
Firstly, you need to navigate to the directory containing your application. After that, you just need to run this command:
ng generate component <component-name>
ng generate component firstComponent
After running this command, you will notice a folder with the name of the component and the folder will include four files(The ones which are mentioned above).
The file with the ‘ts’ extension is the typescript class. And the one with the ‘spec.ts’ is used for the unit testing purpose.
Every component must belong to the NgModule so that it is available for other components. For this, we need to declare our component in the declarations array of your app module. The component is automatically added to the declarations array if you create it with Angular CLI. But if you creating a component manually, you will need to declare it yourself.
2)Creating a component manually
To create a component manually, follow these steps:
- After navigating to the project directory, create a file named componentName.component.ts . This will be your typescript class.
- After that, add the import statement in this file:
import { Component } from ‘@angular/core’;
- Now add @component decorator:
@Component({ })
- Add your CSS selector, templateURL and styleUrls in the @component decorator.
- Add your class.
The @component decorator is basically a function that attaches to the class written below it. This component specifies that it is not a plain class, rather it is a component. The selector, templateURL and the styleUrl is the metadata.
Component’s Template
You can define the HTML template in two ways. You can either define an external file or you can write it directly there.
To define it externally, you write it as:
@component({
templateURL: ‘./componentName.component.html’;
})
To write directly:
@component({
template: ‘<h1>Hello</h1>’;
})
If you have multiple lines of HTML, use backticks:
@component({
template: `<h1>First Heading</h1>
<h2>Second Heading</h2>
<h3>Third Heading</h3>`;
})
Components CSS
Similarly, you can define the CSS styles in two ways. Either in an external file or directly in the component.
To define it externally, you write it as:
@component({
styleUrls: ['./componentName.component.css']
})
However, if you have few lines of code you may also write them directly as shown below.
@component({
styles: [' h1 { color: red; }']
})
How to use these components?
We have already created a component named ‘firstComponent’
Firstly lets change the first-component.component.html file.You may write any message in it.
<h3>This message is from the First Component</h3>
Let us create one more component named ‘secondComponent’. You can use the angular CLI method or you can create it manually. After that, change its html file.
<h3>This message is from the Second Component</h3>
Also change the content in app.component.html
<h2>This is my First app</h2>
Now let us use these components. Each of these components has a selector defined in the typescript class. We will use those selectors to use these components. Move to the app.component.html file. Add the selectors for the component as a custom HTML tag as shown below.
<h2>This is my First app</h2>
<app-first-component></app-first-component>
<app-second-component></app-second-component>
Now, execute the command: ng serve –open
You will notice these components rendered on the page.
Conclusion
In this blog, we understood how the view of our application is defined by the components in Angular and the two ways to create a component. Generally, we use the Angular CLI method to create components. However, it is also important to understand how we create them manually. In addition to this, we understood how to define our HTML and CSS for that component.
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK