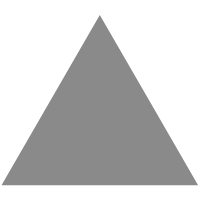
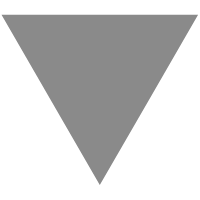
f64 - Rust
source link: https://doc.rust-lang.org/stable/std/primitive.f64.html
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
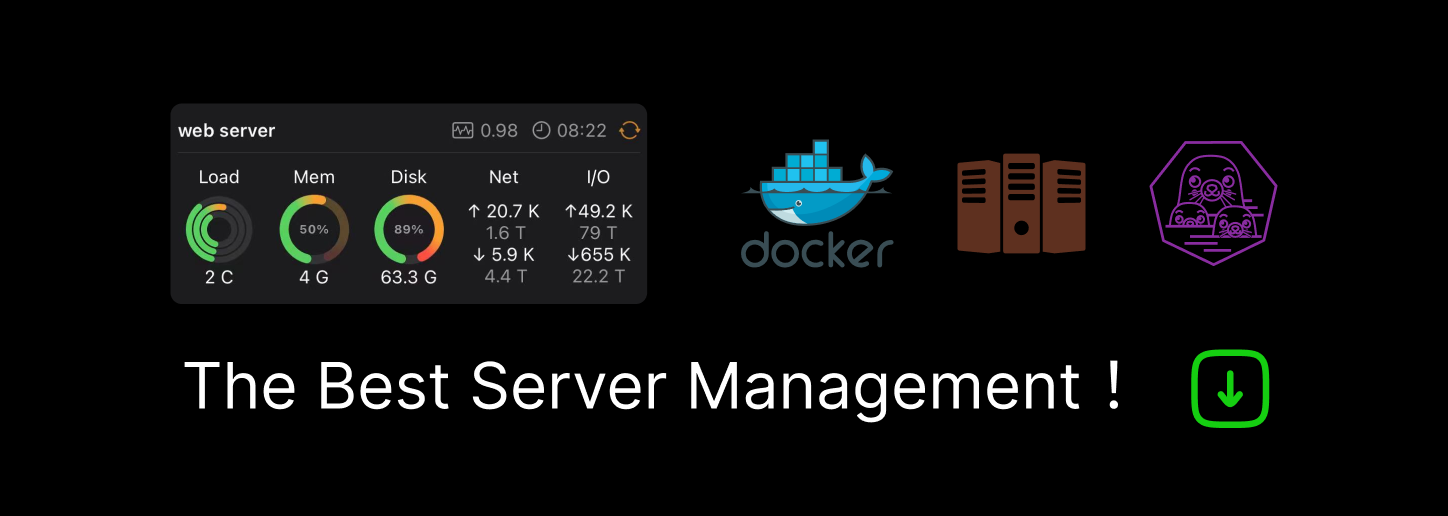
impl f64
[src]
#[must_use = "method returns a new number and does not mutate the original value"]
pub fn floor(self) -> f64
[src][−]Returns the largest integer less than or equal to a number.
Examples
let f = 3.7_f64; let g = 3.0_f64; let h = -3.7_f64; assert_eq!(f.floor(), 3.0); assert_eq!(g.floor(), 3.0); assert_eq!(h.floor(), -4.0);Run
#[must_use = "method returns a new number and does not mutate the original value"]
pub fn ceil(self) -> f64
[src][−]Returns the smallest integer greater than or equal to a number.
Examples
let f = 3.01_f64; let g = 4.0_f64; assert_eq!(f.ceil(), 4.0); assert_eq!(g.ceil(), 4.0);Run
#[must_use = "method returns a new number and does not mutate the original value"]
pub fn round(self) -> f64
[src][−]Returns the nearest integer to a number. Round half-way cases away from
0.0
.
Examples
let f = 3.3_f64; let g = -3.3_f64; assert_eq!(f.round(), 3.0); assert_eq!(g.round(), -3.0);Run
#[must_use = "method returns a new number and does not mutate the original value"]
pub fn trunc(self) -> f64
[src][−]Returns the integer part of a number.
Examples
let f = 3.7_f64; let g = 3.0_f64; let h = -3.7_f64; assert_eq!(f.trunc(), 3.0); assert_eq!(g.trunc(), 3.0); assert_eq!(h.trunc(), -3.0);Run
#[must_use = "method returns a new number and does not mutate the original value"]
pub fn fract(self) -> f64
[src][−]Returns the fractional part of a number.
Examples
let x = 3.6_f64; let y = -3.6_f64; let abs_difference_x = (x.fract() - 0.6).abs(); let abs_difference_y = (y.fract() - (-0.6)).abs(); assert!(abs_difference_x < 1e-10); assert!(abs_difference_y < 1e-10);Run
#[must_use = "method returns a new number and does not mutate the original value"]
pub fn abs(self) -> f64
[src][−]Computes the absolute value of self
. Returns NAN
if the
number is NAN
.
Examples
let x = 3.5_f64; let y = -3.5_f64; let abs_difference_x = (x.abs() - x).abs(); let abs_difference_y = (y.abs() - (-y)).abs(); assert!(abs_difference_x < 1e-10); assert!(abs_difference_y < 1e-10); assert!(f64::NAN.abs().is_nan());Run
#[must_use = "method returns a new number and does not mutate the original value"]
pub fn signum(self) -> f64
[src][−]Returns a number that represents the sign of self
.
1.0
if the number is positive,+0.0
orINFINITY
-1.0
if the number is negative,-0.0
orNEG_INFINITY
NAN
if the number isNAN
Examples
let f = 3.5_f64; assert_eq!(f.signum(), 1.0); assert_eq!(f64::NEG_INFINITY.signum(), -1.0); assert!(f64::NAN.signum().is_nan());Run
#[must_use = "method returns a new number and does not mutate the original value"]
pub fn copysign(self, sign: f64) -> f64
1.35.0[src][−]Returns a number composed of the magnitude of self
and the sign of
sign
.
Equal to self
if the sign of self
and sign
are the same, otherwise
equal to -self
. If self
is a NAN
, then a NAN
with the sign of
sign
is returned.
Examples
let f = 3.5_f64; assert_eq!(f.copysign(0.42), 3.5_f64); assert_eq!(f.copysign(-0.42), -3.5_f64); assert_eq!((-f).copysign(0.42), 3.5_f64); assert_eq!((-f).copysign(-0.42), -3.5_f64); assert!(f64::NAN.copysign(1.0).is_nan());Run
#[must_use = "method returns a new number and does not mutate the original value"]
pub fn mul_add(self, a: f64, b: f64) -> f64
[src][−]Fused multiply-add. Computes (self * a) + b
with only one rounding
error, yielding a more accurate result than an unfused multiply-add.
Using mul_add
may be more performant than an unfused multiply-add if
the target architecture has a dedicated fma
CPU instruction. However,
this is not always true, and will be heavily dependant on designing
algorithms with specific target hardware in mind.
Examples
let m = 10.0_f64; let x = 4.0_f64; let b = 60.0_f64; // 100.0 let abs_difference = (m.mul_add(x, b) - ((m * x) + b)).abs(); assert!(abs_difference < 1e-10);Run
#[must_use = "method returns a new number and does not mutate the original value"]
pub fn div_euclid(self, rhs: f64) -> f64
1.38.0[src][−]Calculates Euclidean division, the matching method for rem_euclid
.
This computes the integer n
such that
self = n * rhs + self.rem_euclid(rhs)
.
In other words, the result is self / rhs
rounded to the integer n
such that self >= n * rhs
.
Examples
let a: f64 = 7.0; let b = 4.0; assert_eq!(a.div_euclid(b), 1.0); // 7.0 > 4.0 * 1.0 assert_eq!((-a).div_euclid(b), -2.0); // -7.0 >= 4.0 * -2.0 assert_eq!(a.div_euclid(-b), -1.0); // 7.0 >= -4.0 * -1.0 assert_eq!((-a).div_euclid(-b), 2.0); // -7.0 >= -4.0 * 2.0Run
#[must_use = "method returns a new number and does not mutate the original value"]
pub fn rem_euclid(self, rhs: f64) -> f64
1.38.0[src][−]Calculates the least nonnegative remainder of self (mod rhs)
.
In particular, the return value r
satisfies 0.0 <= r < rhs.abs()
in
most cases. However, due to a floating point round-off error it can
result in r == rhs.abs()
, violating the mathematical definition, if
self
is much smaller than rhs.abs()
in magnitude and self < 0.0
.
This result is not an element of the function’s codomain, but it is the
closest floating point number in the real numbers and thus fulfills the
property self == self.div_euclid(rhs) * rhs + self.rem_euclid(rhs)
approximatively.
Examples
let a: f64 = 7.0; let b = 4.0; assert_eq!(a.rem_euclid(b), 3.0); assert_eq!((-a).rem_euclid(b), 1.0); assert_eq!(a.rem_euclid(-b), 3.0); assert_eq!((-a).rem_euclid(-b), 1.0); // limitation due to round-off error assert!((-f64::EPSILON).rem_euclid(3.0) != 0.0);Run
#[must_use = "method returns a new number and does not mutate the original value"]
pub fn powi(self, n: i32) -> f64
[src][−]Raises a number to an integer power.
Using this function is generally faster than using powf
Examples
let x = 2.0_f64; let abs_difference = (x.powi(2) - (x * x)).abs(); assert!(abs_difference < 1e-10);Run
#[must_use = "method returns a new number and does not mutate the original value"]
pub fn powf(self, n: f64) -> f64
[src][−]Raises a number to a floating point power.
Examples
let x = 2.0_f64; let abs_difference = (x.powf(2.0) - (x * x)).abs(); assert!(abs_difference < 1e-10);Run
#[must_use = "method returns a new number and does not mutate the original value"]
pub fn sqrt(self) -> f64
[src][−]Returns the square root of a number.
Returns NaN if self
is a negative number.
Examples
let positive = 4.0_f64; let negative = -4.0_f64; let abs_difference = (positive.sqrt() - 2.0).abs(); assert!(abs_difference < 1e-10); assert!(negative.sqrt().is_nan());Run
#[must_use = "method returns a new number and does not mutate the original value"]
pub fn exp(self) -> f64
[src][−]Returns e^(self)
, (the exponential function).
Examples
let one = 1.0_f64; // e^1 let e = one.exp(); // ln(e) - 1 == 0 let abs_difference = (e.ln() - 1.0).abs(); assert!(abs_difference < 1e-10);Run
#[must_use = "method returns a new number and does not mutate the original value"]
pub fn exp2(self) -> f64
[src][−]Returns 2^(self)
.
Examples
let f = 2.0_f64; // 2^2 - 4 == 0 let abs_difference = (f.exp2() - 4.0).abs(); assert!(abs_difference < 1e-10);Run
#[must_use = "method returns a new number and does not mutate the original value"]
pub fn ln(self) -> f64
[src][−]Returns the natural logarithm of the number.
Examples
let one = 1.0_f64; // e^1 let e = one.exp(); // ln(e) - 1 == 0 let abs_difference = (e.ln() - 1.0).abs(); assert!(abs_difference < 1e-10);Run
#[must_use = "method returns a new number and does not mutate the original value"]
pub fn log(self, base: f64) -> f64
[src][−]Returns the logarithm of the number with respect to an arbitrary base.
The result may not be correctly rounded owing to implementation details;
self.log2()
can produce more accurate results for base 2, and
self.log10()
can produce more accurate results for base 10.
Examples
let twenty_five = 25.0_f64; // log5(25) - 2 == 0 let abs_difference = (twenty_five.log(5.0) - 2.0).abs(); assert!(abs_difference < 1e-10);Run
#[must_use = "method returns a new number and does not mutate the original value"]
pub fn log2(self) -> f64
[src][−]Returns the base 2 logarithm of the number.
Examples
let four = 4.0_f64; // log2(4) - 2 == 0 let abs_difference = (four.log2() - 2.0).abs(); assert!(abs_difference < 1e-10);Run
#[must_use = "method returns a new number and does not mutate the original value"]
pub fn log10(self) -> f64
[src][−]Returns the base 10 logarithm of the number.
Examples
let hundred = 100.0_f64; // log10(100) - 2 == 0 let abs_difference = (hundred.log10() - 2.0).abs(); assert!(abs_difference < 1e-10);Run
#[must_use = "method returns a new number and does not mutate the original value"]
pub fn abs_sub(self, other: f64) -> f64
[src][−]you probably meant (self - other).abs()
: this operation is (self - other).max(0.0)
except that abs_sub
also propagates NaNs (also known as fdim
in C). If you truly need the positive difference, consider using that expression or the C function fdim
, depending on how you wish to handle NaN (please consider filing an issue describing your use-case too).
The positive difference of two numbers.
- If
self <= other
:0:0
- Else:
self - other
Examples
let x = 3.0_f64; let y = -3.0_f64; let abs_difference_x = (x.abs_sub(1.0) - 2.0).abs(); let abs_difference_y = (y.abs_sub(1.0) - 0.0).abs(); assert!(abs_difference_x < 1e-10); assert!(abs_difference_y < 1e-10);Run
#[must_use = "method returns a new number and does not mutate the original value"]
pub fn cbrt(self) -> f64
[src][−]Returns the cube root of a number.
Examples
let x = 8.0_f64; // x^(1/3) - 2 == 0 let abs_difference = (x.cbrt() - 2.0).abs(); assert!(abs_difference < 1e-10);Run
#[must_use = "method returns a new number and does not mutate the original value"]
pub fn hypot(self, other: f64) -> f64
[src][−]Calculates the length of the hypotenuse of a right-angle triangle given
legs of length x
and y
.
Examples
let x = 2.0_f64; let y = 3.0_f64; // sqrt(x^2 + y^2) let abs_difference = (x.hypot(y) - (x.powi(2) + y.powi(2)).sqrt()).abs(); assert!(abs_difference < 1e-10);Run
#[must_use = "method returns a new number and does not mutate the original value"]
pub fn sin(self) -> f64
[src][−]Computes the sine of a number (in radians).
Examples
let x = std::f64::consts::FRAC_PI_2; let abs_difference = (x.sin() - 1.0).abs(); assert!(abs_difference < 1e-10);Run
#[must_use = "method returns a new number and does not mutate the original value"]
pub fn cos(self) -> f64
[src][−]Computes the cosine of a number (in radians).
Examples
let x = 2.0 * std::f64::consts::PI; let abs_difference = (x.cos() - 1.0).abs(); assert!(abs_difference < 1e-10);Run
#[must_use = "method returns a new number and does not mutate the original value"]
pub fn tan(self) -> f64
[src][−]Computes the tangent of a number (in radians).
Examples
let x = std::f64::consts::FRAC_PI_4; let abs_difference = (x.tan() - 1.0).abs(); assert!(abs_difference < 1e-14);Run
#[must_use = "method returns a new number and does not mutate the original value"]
pub fn asin(self) -> f64
[src][−]Computes the arcsine of a number. Return value is in radians in the range [-pi/2, pi/2] or NaN if the number is outside the range [-1, 1].
Examples
let f = std::f64::consts::FRAC_PI_2; // asin(sin(pi/2)) let abs_difference = (f.sin().asin() - std::f64::consts::FRAC_PI_2).abs(); assert!(abs_difference < 1e-10);Run
#[must_use = "method returns a new number and does not mutate the original value"]
pub fn acos(self) -> f64
[src][−]Computes the arccosine of a number. Return value is in radians in the range [0, pi] or NaN if the number is outside the range [-1, 1].
Examples
let f = std::f64::consts::FRAC_PI_4; // acos(cos(pi/4)) let abs_difference = (f.cos().acos() - std::f64::consts::FRAC_PI_4).abs(); assert!(abs_difference < 1e-10);Run
#[must_use = "method returns a new number and does not mutate the original value"]
pub fn atan(self) -> f64
[src][−]Computes the arctangent of a number. Return value is in radians in the range [-pi/2, pi/2];
Examples
let f = 1.0_f64; // atan(tan(1)) let abs_difference = (f.tan().atan() - 1.0).abs(); assert!(abs_difference < 1e-10);Run
#[must_use = "method returns a new number and does not mutate the original value"]
pub fn atan2(self, other: f64) -> f64
[src][−]Computes the four quadrant arctangent of self
(y
) and other
(x
) in radians.
x = 0
,y = 0
:0
x >= 0
:arctan(y/x)
->[-pi/2, pi/2]
y >= 0
:arctan(y/x) + pi
->(pi/2, pi]
y < 0
:arctan(y/x) - pi
->(-pi, -pi/2)
Examples
// Positive angles measured counter-clockwise // from positive x axis // -pi/4 radians (45 deg clockwise) let x1 = 3.0_f64; let y1 = -3.0_f64; // 3pi/4 radians (135 deg counter-clockwise) let x2 = -3.0_f64; let y2 = 3.0_f64; let abs_difference_1 = (y1.atan2(x1) - (-std::f64::consts::FRAC_PI_4)).abs(); let abs_difference_2 = (y2.atan2(x2) - (3.0 * std::f64::consts::FRAC_PI_4)).abs(); assert!(abs_difference_1 < 1e-10); assert!(abs_difference_2 < 1e-10);Run
pub fn sin_cos(self) -> (f64, f64)
[src][−]
Simultaneously computes the sine and cosine of the number, x
. Returns
(sin(x), cos(x))
.
Examples
let x = std::f64::consts::FRAC_PI_4; let f = x.sin_cos(); let abs_difference_0 = (f.0 - x.sin()).abs(); let abs_difference_1 = (f.1 - x.cos()).abs(); assert!(abs_difference_0 < 1e-10); assert!(abs_difference_1 < 1e-10);Run
#[must_use = "method returns a new number and does not mutate the original value"]
pub fn exp_m1(self) -> f64
[src][−]Returns e^(self) - 1
in a way that is accurate even if the
number is close to zero.
Examples
let x = 1e-16_f64; // for very small x, e^x is approximately 1 + x + x^2 / 2 let approx = x + x * x / 2.0; let abs_difference = (x.exp_m1() - approx).abs(); assert!(abs_difference < 1e-20);Run
#[must_use = "method returns a new number and does not mutate the original value"]
pub fn ln_1p(self) -> f64
[src][−]Returns ln(1+n)
(natural logarithm) more accurately than if
the operations were performed separately.
Examples
let x = 1e-16_f64; // for very small x, ln(1 + x) is approximately x - x^2 / 2 let approx = x - x * x / 2.0; let abs_difference = (x.ln_1p() - approx).abs(); assert!(abs_difference < 1e-20);Run
#[must_use = "method returns a new number and does not mutate the original value"]
pub fn sinh(self) -> f64
[src][−]Hyperbolic sine function.
Examples
let e = std::f64::consts::E; let x = 1.0_f64; let f = x.sinh(); // Solving sinh() at 1 gives `(e^2-1)/(2e)` let g = ((e * e) - 1.0) / (2.0 * e); let abs_difference = (f - g).abs(); assert!(abs_difference < 1e-10);Run
#[must_use = "method returns a new number and does not mutate the original value"]
pub fn cosh(self) -> f64
[src][−]Hyperbolic cosine function.
Examples
let e = std::f64::consts::E; let x = 1.0_f64; let f = x.cosh(); // Solving cosh() at 1 gives this result let g = ((e * e) + 1.0) / (2.0 * e); let abs_difference = (f - g).abs(); // Same result assert!(abs_difference < 1.0e-10);Run
#[must_use = "method returns a new number and does not mutate the original value"]
pub fn tanh(self) -> f64
[src][−]Hyperbolic tangent function.
Examples
let e = std::f64::consts::E; let x = 1.0_f64; let f = x.tanh(); // Solving tanh() at 1 gives `(1 - e^(-2))/(1 + e^(-2))` let g = (1.0 - e.powi(-2)) / (1.0 + e.powi(-2)); let abs_difference = (f - g).abs(); assert!(abs_difference < 1.0e-10);Run
#[must_use = "method returns a new number and does not mutate the original value"]
pub fn asinh(self) -> f64
[src][−]Inverse hyperbolic sine function.
Examples
let x = 1.0_f64; let f = x.sinh().asinh(); let abs_difference = (f - x).abs(); assert!(abs_difference < 1.0e-10);Run
#[must_use = "method returns a new number and does not mutate the original value"]
pub fn acosh(self) -> f64
[src][−]Inverse hyperbolic cosine function.
Examples
let x = 1.0_f64; let f = x.cosh().acosh(); let abs_difference = (f - x).abs(); assert!(abs_difference < 1.0e-10);Run
#[must_use = "method returns a new number and does not mutate the original value"]
pub fn atanh(self) -> f64
[src][−]Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK