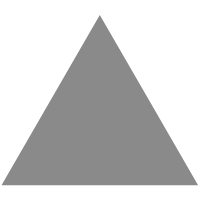
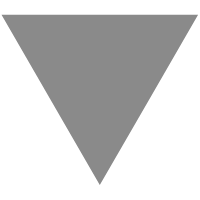
Validity Checks using Clojure
source link: https://blog.knoldus.com/validity-checks-using-clojure/
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
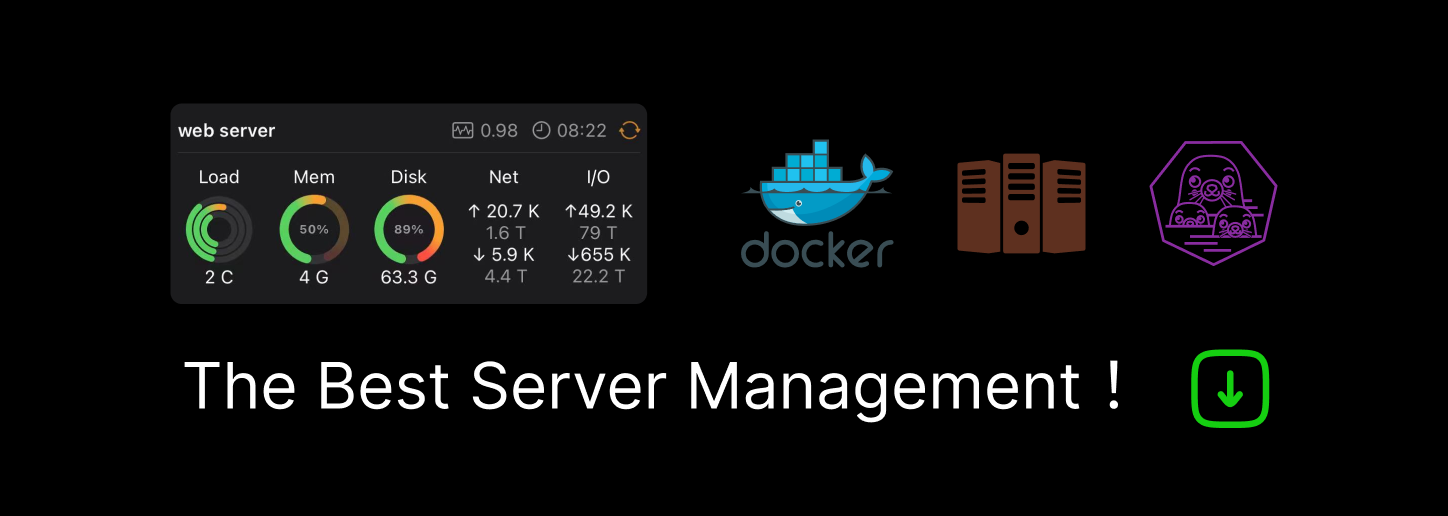
Validity Checks using Clojure
For putting Validation checks using Clojure code, clojure noir library checks are used.
One needs to first create a form page using basic html for placing the checks.As an Example here, I am using a registration page text boxes to put the validation checks over the required fields.
xxxxxxxxxx
<label for="id">
{% if id-error %}
<div>{{id-error}}</div>
{% endif %}
<input id="id" name="id" placeholder="User Name" type="text" value={{id}}></input>
</label>
<label for="email">
{% if id-error %}
<div>{{email-error}}</div>
{% endif %}
<input id="email" name="email" placeholder="Email" type="text" value={{email}}></input>
</label>
<label for="pass">
{% if pass-error %}
<div>{{pass-error}}</div>
{% endif %}
<input id="pass" name="pass" placeholder="Password" type="password" value={{pass}}></input>
</label>
<label for="confirmPass">
{% if confirmPass-error %}
<div>{{confirmPass-error}}</div>
{% endif %}
<input id="confirmPass" name="confirmPass" placeholder="Confirm Password" type="password" value={{confirmPass}}></input>
</label>
Then we move ahead by creating a register.clj file where all validations are defined under the namespace definition:
xxxxxxxxxx
(ns projectName.routes.register
(:use compojure.core)
(:require
[noir.session :as session]
[noir.response :as resp]
[noir.validation :as vali])
Next is to create the route that defines the register function which is called when the register page is rendered.
xxxxxxxxxx
(defroutes register-routes
(GET "/register" []
(register))
the above route calls the following function:
xxxxxxxxxx
(defn register [& [id]]
(layout/render
"register.html"
{:id id
:id-error (vali/on-error :id first)
:email-error (vali/on-error :email first)
:pass-error (vali/on-error :pass first)
:confirmPass-error (vali/on-error :confirmPass first)}))
where, [id] for any number of arguments passed, (layout/render) renders with that html page where validations are required to be placed, :id-error/email-error are variables placed in the html form that will define the error block, (vali/on-error) denotes noir validation structure.
Next step is to define the errors and put checks over the required fields i.e; defining the errors for above created check blocks.
xxxxxxxxxx
(defn valid? [id email pass confirmPass]
(vali/rule (vali/has-value? id)
[:id "Username is required"])
(vali/rule (vali/is-email? email)
[:email "Invalid Email Address"])
(vali/rule (vali/min-length? id 8)
[:id "Username must be at least 8 characters long"])
(vali/rule (vali/min-length? pass 8)
[:pass "Password must be at least 8 characters long"])
(vali/rule (= pass confirmPass)
[:confirmPass "Passwords does not match"])
(not (vali/errors? :id :email :pass :confirmPass)))
Joseph Ross is a Principal Consultant at Knoldus Inc. having more than 10 years of experience. Joseph has a passion for identifying challenges and give impactful solutions to the clients. He is a football fan and loves to watch TV series. Joseph has a cross-functional business operations and technology consulting experience. Joseph is familiar with programming languages such as Scala, C++, Java, CSS and HTML.
Written by Joseph Ross
Joseph Ross is a Principal Consultant at Knoldus Inc. having more than 10 years of experience. Joseph has a passion for identifying challenges and give impactful solutions to the clients. He is a football fan and loves to watch TV series. Joseph has a cross-functional business operations and technology consulting experience. Joseph is familiar with programming languages such as Scala, C++, Java, CSS and HTML.
Post navigation
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK