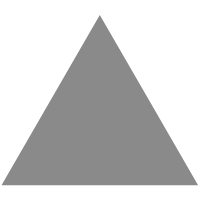
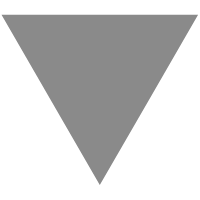
How to drop rows in Pandas DataFrame by index labels? - GeeksforGeeks
source link: https://www.geeksforgeeks.org/how-to-drop-rows-in-pandas-dataframe-by-index-labels/?ref=leftbar-rightbar
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
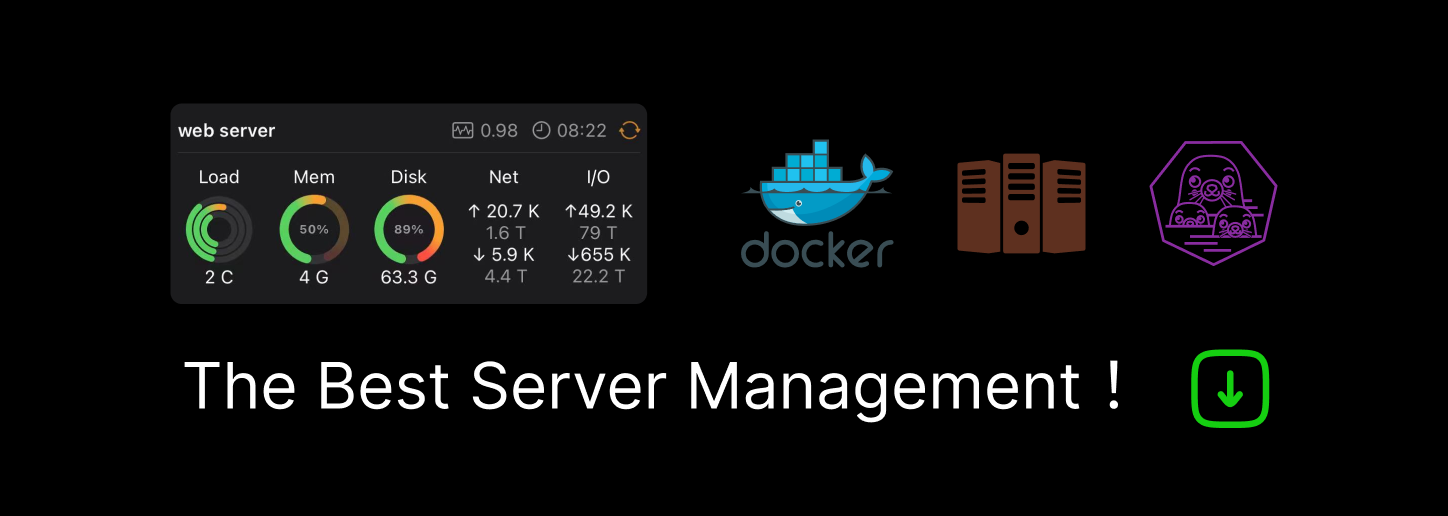
How to drop rows in Pandas DataFrame by index labels?
Last Updated: 02-07-2020Pandas provide data analysts a way to delete and filter data frame using .drop()
method. Rows can be removed using index label or column name using this method.
Syntax:
DataFrame.drop(labels=None, axis=0, index=None, columns=None, level=None, inplace=False, errors=’raise’)Parameters:
labels: String or list of strings referring row or column name.
axis: int or string value, 0 ‘index’ for Rows and 1 ‘columns’ for Columns.
index or columns: Single label or list. index or columns are an alternative to axis and cannot be used together.
level: Used to specify level in case data frame is having multiple level index.
inplace: Makes changes in original Data Frame if True.
errors: Ignores error if any value from the list doesn’t exists and drops rest of the values when errors = ‘ignore’Return type: Dataframe with dropped values
Now, Let’s create a sample dataframe
filter_none
edit
close
play_arrow
link
brightness_4
code
# import pandas library
import
pandas as pd
# dictionary with list object in values
details
=
{
'Name'
: [
'Ankit'
,
'Aishwarya'
,
'Shaurya'
,
'Shivangi'
],
'Age'
: [
23
,
21
,
22
,
21
],
'University'
: [
'BHU'
,
'JNU'
,
'DU'
,
'BHU'
],
}
# creating a Dataframe object
df
=
pd.DataFrame(details,columns
=
[
'Name'
,
'Age'
,
'University'
],
index
=
[
'a'
,
'b'
,
'c'
,
'd'
])
df
Output:
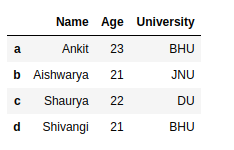
Example #1: Delete a single Row in DataFrame by Row Index Label
filter_none
edit
close
play_arrow
link
brightness_4
code
# import pandas library
import
pandas as pd
# dictionary with list object in values
details
=
{
'Name'
: [
'Ankit'
,
'Aishwarya'
,
'Shaurya'
,
'Shivangi'
],
'Age'
: [
23
,
21
,
22
,
21
],
'University'
: [
'BHU'
,
'JNU'
,
'DU'
,
'BHU'
],
}
# creating a Dataframe object
df
=
pd.DataFrame(details, columns
=
[
'Name'
,
'Age'
,
'University'
],
index
=
[
'a'
,
'b'
,
'c'
,
'd'
])
# return a new dataframe by dropping a
# row 'c' from dataframe
update_df
=
df.drop(
'c'
)
update_df
Output :
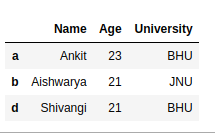
Example #2: Delete Multiple Rows in DataFrame by Index Labels
filter_none
edit
close
play_arrow
link
brightness_4
code
# import pandas library
import
pandas as pd
# dictionary with list object in values
details
=
{
'Name'
: [
'Ankit'
,
'Aishwarya'
,
'Shaurya'
,
'Shivangi'
],
'Age'
: [
23
,
21
,
22
,
21
],
'University'
: [
'BHU'
,
'JNU'
,
'DU'
,
'BHU'
],
}
# creating a Dataframe object
df
=
pd.DataFrame(details, columns
=
[
'Name'
,
'Age'
,
'University'
],
index
=
[
'a'
,
'b'
,
'c'
,
'd'
])
# return a new dataframe by dropping a row
# 'b' & 'c' from dataframe
update_df
=
df.drop([
'b'
,
'c'
])
update_df
Output :
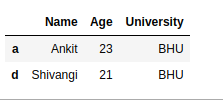
Example #3: Delete a Multiple Rows by Index Position in DataFrame
filter_none
edit
close
play_arrow
link
brightness_4
code
# import pandas library
import
pandas as pd
# dictionary with list object in values
details
=
{
'Name'
: [
'Ankit'
,
'Aishwarya'
,
'Shaurya'
,
'Shivangi'
],
'Age'
: [
23
,
21
,
22
,
21
],
'University'
: [
'BHU'
,
'JNU'
,
'DU'
,
'BHU'
],
}
# creating a Dataframe object
df
=
pd.DataFrame(details, columns
=
[
'Name'
,
'Age'
,
'University'
],
index
=
[
'a'
,
'b'
,
'c'
,
'd'
])
# return a new dataframe by dropping a row
# 'b' & 'c' from dataframe using their
# respective index position
update_df
=
df.drop([df.index[
1
], df.index[
2
]])
update_df
Output :
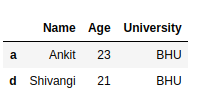
Example #4: Delete rows from dataFrame in Place
filter_none
edit
close
play_arrow
link
brightness_4
code
# import pandas library
import
pandas as pd
# dictionary with list object in values
details
=
{
'Name'
: [
'Ankit'
,
'Aishwarya'
,
'Shaurya'
,
'Shivangi'
],
'Age'
: [
23
,
21
,
22
,
21
],
'University'
: [
'BHU'
,
'JNU'
,
'DU'
,
'BHU'
],
}
# creating a Dataframe object
df
=
pd.DataFrame(details, columns
=
[
'Name'
,
'Age'
,
'University'
],
index
=
[
'a'
,
'b'
,
'c'
,
'd'
])
# droppping a row 'c' & 'd' from actual dataframe
df.drop([
'c'
,
'd'
], inplace
=
True
)
df
Output :
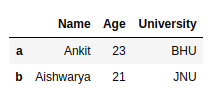
Attention geek! Strengthen your foundations with the Python Programming Foundation Course and learn the basics.
To begin with, your interview preparations Enhance your Data Structures concepts with the Python DS Course.
If you like GeeksforGeeks and would like to contribute, you can also write an article using contribute.geeksforgeeks.org or mail your article to [email protected]. See your article appearing on the GeeksforGeeks main page and help other Geeks.
Please Improve this article if you find anything incorrect by clicking on the "Improve Article" button below.
Be the First to upvote.
No votes yet.
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK