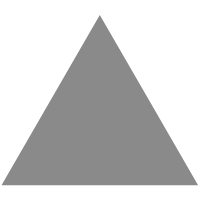
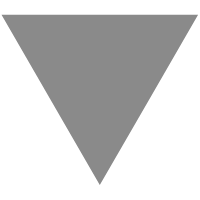
LeetCode: 2. Add Two Numbers
source link: https://mozillazg.com/2020/09/leetcode-2-add-two-numbers.html
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
题目¶
原题地址:https://leetcode.com/problems/add-two-numbers/
You are given two non-empty linked lists representing two non-negative integers. The digits are stored in reverse order and each of their nodes contain a single digit. Add the two numbers and return it as a linked list.
You may assume the two numbers do not contain any leading zero, except the number 0 itself.
Example:
Input: (2 -> 4 -> 3) + (5 -> 6 -> 4) Output: 7 -> 0 -> 8 Explanation: 342 + 465 = 807.
解法¶
按照加法运算的规则计算各个节点的值¶
因为是反序表示的正整数所以不会有进位导致的 head 变化的情况,只需要遍历两个链表然后使用小学学的进位加法运算方法将值进行相加即可:
- 将两个节点的值进行相加按进位加法得出新节点值,然后让进位的值参与下一个节点的计算即可。
2 -> 4 -> 3 + 5 -> 6 -> 4 = 7 -> 0(10进一位) -> 8(7+1)
这个方法的 Python 代码类似下面这样:
# Definition for singly-linked list. # class ListNode(object): # def __init__(self, val=0, next=None): # self.val = val # self.next = next class Solution(object): def addTwoNumbers(self, l1, l2): head = ListNode(None) pre = head carry = 0 while l1 is not None or l2 is not None or carry > 0: l1_val = 0 l2_val = 0 if l1 is not None: l1_val = l1.val l1 = l1.next if l2 is not None: l2_val = l2.val l2 = l2.next total = l1_val + l2_val + carry carry = total // 10 val = total % 10 if head.val is None: head.val = val else: node = ListNode(val) pre.next = node pre = node return head
之所以 while 循环哪里要加个 carry > 0 的条件是为了支持链表最后一个元素相加后出现进位的情况, 此时因为已经到了链表尾部如果不判断 carry > 0 的情况的话,就会漏了这个进位值。 如果不想在 while 循环里处理的话,也可以在 while 循环结束再加一个 if carry > 0: pre.next = ListNode(carry) 来支持这种情况。
input: (5) + (5) ouput: 0 -> 1
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK