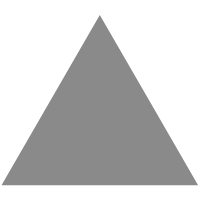
0
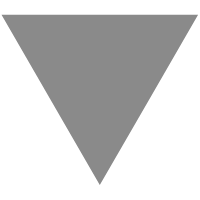
Go 语言程序员的进化
source link: https://www.techug.com/post/the-evolution-of-a-go-programmer/
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
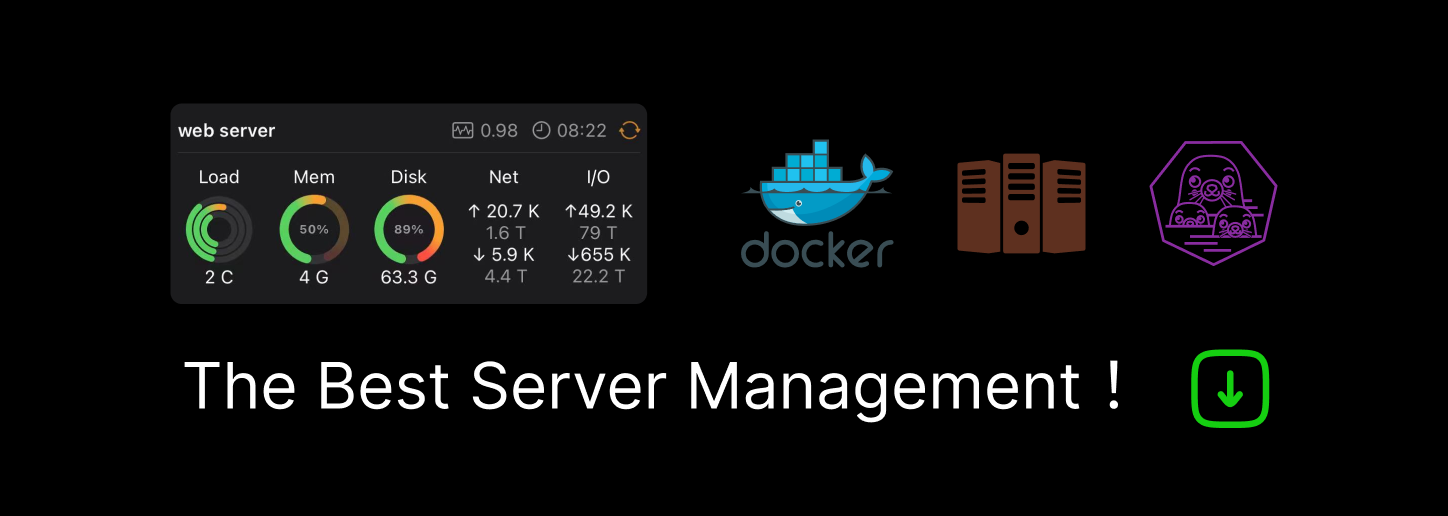
初级 Go 语言程序员
package fac
func Factorial(n int) int {
res := 1
for i := 1; i <= n; i++ {
res *= i
}
return res
}
函数式 Go 语言程序员
package fac
func Factorial(n int) int {
if n == 0 {
return 1
} else {
return Factorial(n - 1) * n
}
}
泛型式 Go 语言程序员
package fac
func Factorial(n interface{}) interface{} {
v, valid := n.(int)
if !valid {
return 0
}
res := 1
for i := 1; i <= v; i++ {
res *= i
}
return res
}
多线程优化式 Go 程序员
package fac
import "sync"
func Factorial(n int) int {
var (
left, right = 1, 1
wg sync.WaitGroup
)
wg.Add(2)
pivot := n / 2
go func() {
for i := 1; i < pivot; i++ {
left *= i
}
wg.Done()
}()
go func() {
for i := pivot; i <= n; i++ {
right *= i
}
wg.Done()
}()
wg.Wait()
return left * right
}
会使用 Go 模式
package fac
func Factorial(n int) <-chan int {
ch := make(chan int)
go func() {
prev := 1
for i := 1; i <= n; i++ {
v := prev * i
ch <- v
prev = v
}
close(ch)
}()
return ch
}
会用成熟的解决方案修复 Go 语言弱点
package fac
/**
* @see https://en.wikipedia.org/wiki/Factorial
*/
type IFactorial interface {
CalculateFactorial() int
}
// FactorialImpl implements IFactorial.
var _ IFactorial = (*FactorialImpl)(nil)
/**
* Used to find factorial of the n.
*/
type FactorialImpl struct {
/**
* The n.
*/
n int
}
/**
* Constructor of the FactorialImpl.
*
* @param n the n.
*/
func NewFactorial(n int) *FactorialImpl {
return &FactorialImpl{
n: n,
}
}
/**
* Gets the n to use in factorial function.
*
* @return int.
*/
func (this *FactorialImpl) GetN() int {
return this.n
}
/**
* Sets the n to use in factorial function.
*
* @param n the n.
* @return void.
*/
func (this *FactorialImpl) SetN(n int) {
this.n = n
}
/**
* Returns factorial of the n.
*
* @todo remove "if" statement. Maybe we should use a factory or somthing?
*
* @return int.
*/
func (this *FactorialImpl) CalculateFactorial() int {
if this.n == 0 {
return 1
}
n := this.n
this.n = this.n - 1
return this.CalculateFactorial() * n
}
高级 Go 程序员
package fac
// Factorial returns n!.
func Factorial(n int) int {
res := 1
for i := 1; i <= n; i++ {
res *= i
}
return res
}
Rob Pike (Go 语言之父)
package fac // Factorial returns n!. func Factorial(n int) int { res := 1 for i := 1; i <= n; i++ { res *= i } return res }
本文文字及图片出自 The Evolution of a Go Programmer
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK