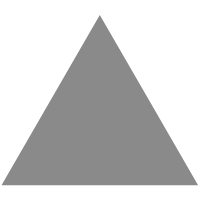
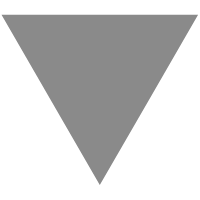
5 Essential Practices for Front-End Developers (React Edition)
source link: https://dev.to/sufian/5-essential-practices-for-front-end-developers-react-edition-3h96
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
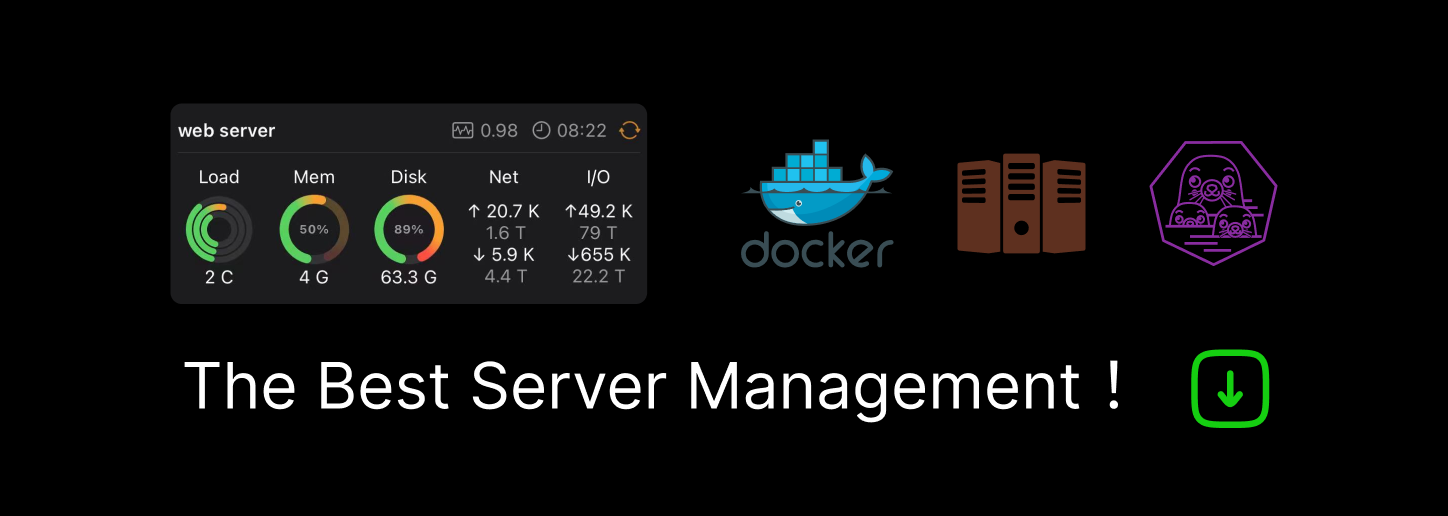
Introduction
Embarking on a new professional journey is often accompanied by excitement and high expectations. However, the reality can be starkly different when faced with a codebase resembling a chaotic puzzle. To mitigate this common scenario, especially for developers in senior roles, adopting specific best practices becomes imperative. This ensures not only code quality but also positions you as a meticulous professional, garnering recognition and potential promotions within the company.
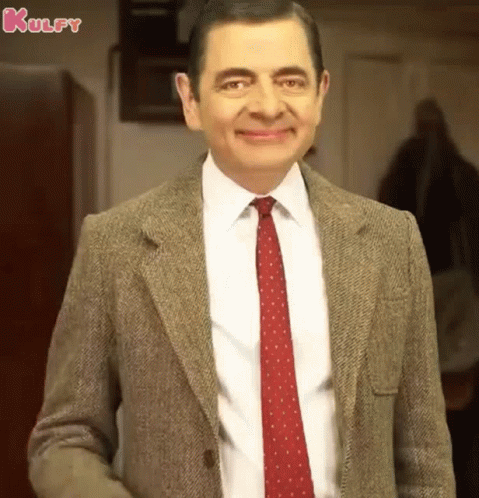
1. Optimal Path Handling: Absolute Paths Over Relative Paths
Imagine navigating a maze with nothing but cryptic clues like "go back four steps and turn left twice." That's what relative paths in your code can feel like. Instead, embrace the power of absolute paths! These provide the full address of a file, making imports crystal clear and saving you from endless guessing games. Setting them up might involve some configuration magic with tools like Webpack or TypeScript, but trust us, it's worth the effort.
Bonus Tip: For projects using create-react-app, a simple jsconfig.json file can be your hero. With a few lines of code, you can define a base URL for imports, transforming that monster path ../../../../../components/Button into a sleek @/components/Button.
If you’re using TypeScript, add the following configurations to your “tsconfig.json” file:
{
"compilerOptions": {
"baseUrl": "src",
"paths": {
"@/*": ["src/*"]
}
},
"include": ["src"]
}
By doing so, you can transform a code snippet that looks like this:
//from this
import { Button } from '../../../../components/Button'
import { Icon } from '../../../../components/Icon'
import { Input } from '../../../../components/Input'
Into something cleaner and easier to read, like:
//to this
import { Button } from '@/components/Button'
import { Icon } from '@/components/Icon'
import { Input } from '@/components/Input'
2. Streamlining Module Organization: The Power of "Export Barrel"
Code readability and maintenance receive a substantial boost with the "export barrel" technique, also known as "re-export." Creating an "index.js" (or "index.ts" for TypeScript) file within a folder and exporting all modules simplifies imports and enhances code organization.
Implementation Example:
Imagine a "components" folder with "Button.tsx," "Icon.tsx," and "Input.tsx." Utilizing the "export barrel," you can create an "index.ts" file to streamline imports:
export * from './Button'
export * from './Icon'
export * from './Input'
This practice not only reduces the need for individual imports but also contributes to a cleaner and more comprehensible codebase—essential for medium to large-scale projects.
3. Choosing Between “Export Default” and “Named Export”
As we delve into the topic of the “export barrel,” it’s essential to note that it can conflict with the use of “export default.” If this is not clear, I’ll illustrate the situation with examples:
Let’s go back to our components:
export const Button = () => {
return <button>Button</button>
}
export default Button
export const Icon = () => {
return <svg>Icon</svg>
}
export default Icon
export const Input = () => {
return <input />
}
export default Input
Imagine each of these components is in a separate file, and you want to import all of them at once. If you are accustomed to default imports, you might attempt something like:
import Button from '@/components'
import Icon from '@/components'
import Input from '@/components'
However, this won’t work because JavaScript can’t determine which “export default” to use, resulting in errors. You would be forced to do something like this:
import Button from '@/components/Button'
import Icon from '@/components/Icon'
import Input from '@/components/Input'
This, however, negates the advantage of the “export barrel.” How can you resolve this dilemma? The solution is simple: use “Named Export,” which is exporting without “default”:
import { Button, Icon, Input } from '@/components'
Another critical issue associated with “export default” is the ability to rename what you’re importing. I’ll share a real-life example from early in my career. I inherited a React Native project where the previous developer used “export default” for absolutely everything. There were screens named “Login,” “Register,” and “ForgotPassword.” However, all three screens were copies of each other with minor modifications. The problem was that, at the end of each screen file, there was an “export default Login.” This resulted in confusion, as the route file imported correctly:
import Login from '../../screens/Login'
import Register from '../../screens/Register'
import ForgotPassword from '../../screens/ForgotPassword'
// Further down, the usage in routes:
{
ResetPassword: { screen: ResetPassword },
Login: { screen: LoginScreen },
Register: { screen: RegisterScreen },
}
But when opening the screen files, they all exported the same name:
const login() {
return <>tela de login</>
}
export default Login
const login() {
return <>tela de registro</>
}
export default Login
const login() {
return <>tela de esqueci minha senha</>
}
export default Login
This created a maintenance nightmare with constant confusion and the need for extreme vigilance to avoid errors.
In summary, it’s highly recommended to use “Named Export” in most cases in your project and resort to “export default” only when strictly necessary. There are situations, such as Next.js routes and React.lazy, that may require the use of “export default.” However, it’s crucial to strike a balance between code clarity and compliance with specific requirements.
4. Proper File Naming Conventions
Let’s imagine you have a components folder with the following files:
-components:
----Button.tsx
----Icon.tsx
----Input.tsx
Now, suppose you want to separate styles, logic, or types of these components into separate files. How would you name these files? An obvious approach might be the following:
--components:
----Button.tsx
----Button.styles.css
----Icon.tsx
----Icon.styles.css
----Input.tsx
----Input.styles.css
Certainly, this approach can seem somewhat disorganized and challenging to understand, especially when you intend to further divide the component into distinct files, such as logic or types. But how can you keep the structure organized? Here’s the solution:
--components:
----Button
------index.ts (exports everything necessary)
------types.ts
------styles.css
------utils.ts
------component.tsx
----Icon
------index.ts (exports everything necessary)
------types.ts
------styles.css
------utils.ts
------component.tsx
----Input
------index.ts (exports everything necessary)
------types.ts
------styles.css
------utils.ts
------component.tsx
This approach makes it easy to identify the purpose of each file and simplifies the search for what you need. Additionally, if you’re using Next.js or a similar framework, you can adapt the file naming to indicate whether the component is intended for the client or server side. For example:
--components:
----RandomComponent
------index.ts (exports everything necessary)
------types.ts
------styles.css
------utils.ts
------component.tsx
----RandomComponent2
------index.ts (exports everything necessary)
------types.ts
------styles.css
------utils.ts
------component.server.tsx
This way, it becomes extremely simple to distinguish whether a component is meant for the client or server side without the need to open the code for verification. Organizing and standardizing file naming is essential to maintain clarity and efficiency in development projects.
5. Proper Use of ESLint and Prettier for Code Standardization
Imagine yourself working on a project with over 10 colleagues, each bringing their own coding style from past experiences. Here’s where ESLint and Prettier come into play. They play a crucial role in maintaining code consistency throughout the team.
Prettier acts as a kind of “guardian” of code formatting, ensuring that everyone adheres to the style guidelines set for the project. If the project’s standard dictates the use of double quotes, for example, you can’t simply opt for single quotes because Prettier will automatically replace them. Furthermore, Prettier can perform various other fixes and formatting, like code alignment, adding semicolons at the end of statements, and more. You can check the specific Prettier rules in the official documentation: Prettier Options.
ESLint, on the other hand, enforces specific rules on the code, helping to maintain a cohesive and coherent codebase. For instance, it can enforce the use of arrow functions over regular functions, ensure that the React dependencies array is properly filled, prohibit the use of “var” declarations in favor of “let” and “const,” and apply naming conventions like camelCase. You can find the specific ESLint rules in the official documentation: ESLint Rules.
The combined use of ESLint and Prettier helps to maintain consistency in source code. Without them, each developer can follow their own style, which can lead to conflicts and maintenance difficulties in the future. Having these tools set up is essential for the longevity of a project, as it helps keep the code organized and easy to understand. If you’re not already using ESLint and Prettier, seriously consider incorporating them into your workflow, as they will greatly benefit your team and your project as a whole.
Conclusion:
By incorporating these best practices into your React development workflow, you contribute to a more organized, readable, and maintainable codebase. Stay committed to improving coding standards, and don't forget to Like 🦄 if you found these practices useful! Happy coding! 🚀
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK