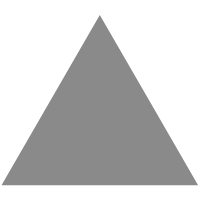
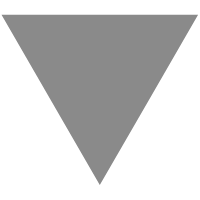
Creating a large language model from scratch: A beginner's guide
source link: https://www.pluralsight.com/resources/blog/data/how-build-large-language-model
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
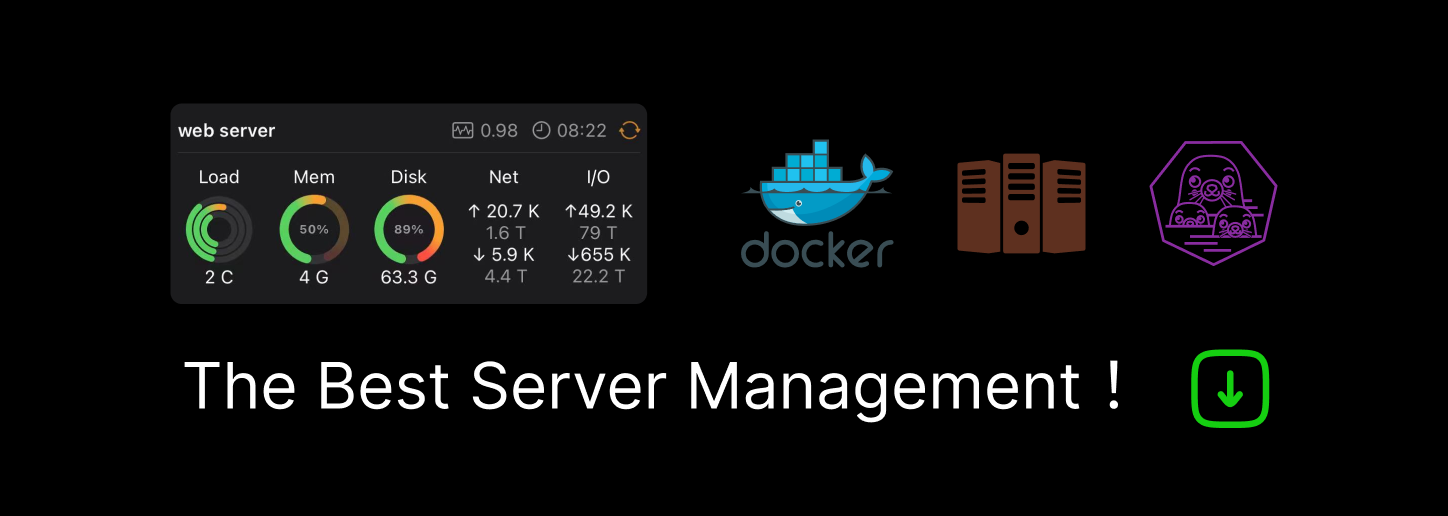
Creating a large language model from scratch
It is a type of artificial intelligence model specifically designed to understand, interpret, generate, and sometimes translate human language. These models are a subset of machine learning models and are part of the broader field of natural language processing (NLP). Let's break down the concept to understand it better:
Key Characteristics of Large Language Models:
Large Scale: As the name suggests, these models are 'large' not just in their physical size in terms of the number of parameters they contain, but also in the vast amount of data they are trained on. Models like GPT-3, BERT, and T5 consist of billions of parameters and are trained on diverse datasets comprising texts from books, websites, and other sources.
Understanding Context: One of the primary strengths of LLMs is their ability to understand the context. Unlike earlier models that focused on individual words or phrases in isolation, LLMs consider the entire sentence or paragraph, allowing them to comprehend nuances, ambiguities, and the flow of language.
Generating Human-Like Text: LLMs are known for their ability to generate text that closely resembles human writing. This includes completing sentences, writing essays, creating poetry, or even generating code. The advanced models can maintain a theme or style over long passages.
Adaptability: These models can be fine-tuned or adapted for specific tasks, like answering questions, translating languages, summarizing texts, or even creating content for specific domains like legal, medical, or technical fields.
The Transformer: The Engine Behind LLMs
At the heart of most LLMs is the Transformer architecture, introduced in the paper "Attention Is All You Need" by Vaswani et al. (2017). Imagine the Transformer as an advanced orchestra, where different instruments (layers and attention mechanisms) work in harmony to understand and generate language.
import tensorflow as tf
from tensorflow.keras.layers import MultiHeadAttention, LayerNormalization, Dense
class TransformerEncoderLayer(tf.keras.layers.Layer):
def __init__(self, d_model, num_heads, dff, rate=0.1):
super(TransformerEncoderLayer, self).__init__()
self.mha = MultiHeadAttention(num_heads, d_model)
self.ffn = tf.keras.Sequential([
Dense(dff, activation='relu'),
Dense(d_model)
])
self.layernorm1 = LayerNormalization(epsilon=1e-6)
self.layernorm2 = LayerNormalization(epsilon=1e-6)
self.dropout1 = tf.keras.layers.Dropout(rate)
self.dropout2 = tf.keras.layers.Dropout(rate)
def call(self, x, training):
attn_output = self.mha(x, x, x)
attn_output = self.dropout1(attn_output, training=training)
out1 = self.layernorm1(x + attn_output)
ffn_output = self.ffn(out1)
ffn_output = self.dropout2(ffn_output, training=training)
out2 = self.layernorm2(out1 + ffn_output)
return out2
class TransformerDecoderLayer(tf.keras.layers.Layer):
def __init__(self, d_model, num_heads, dff, rate=0.1):
super(TransformerDecoderLayer, self).__init__()
self.mha1 = MultiHeadAttention(num_heads, d_model)
self.mha2 = MultiHeadAttention(num_heads, d_model)
self.ffn = tf.keras.Sequential([
Dense(dff, activation='relu'),
Dense(d_model)
])
self.layernorm1 = LayerNormalization(epsilon=1e-6)
self.layernorm2 = LayerNormalization(epsilon=1e-6)
self.layernorm3 = LayerNormalization(epsilon=1e-6)
self.dropout1 = tf.keras.layers.Dropout(rate)
self.dropout2 = tf.keras.layers.Dropout(rate)
self.dropout3 = tf.keras.layers.Dropout(rate)
def call(self, x, enc_output, training, look_ahead_mask, padding_mask):
attn1, attn_weights_block1 = self.mha1(x, x, x, look_ahead_mask)
attn1 = self.dropout1(attn1, training=training)
out1 = self.layernorm1(attn1 + x)
attn2, attn_weights_block2 = self.mha2(enc_output, enc_output, out1, padding_mask)
attn2 = self.dropout2(attn2, training=training)
out2 = self.layernorm2(attn2 + out1)
ffn_output = self.ffn(out2)
ffn_output = self.dropout3(ffn_output, training=training)
out3 = self.layernorm3(ffn_output + out2)
return out3, attn_weights_block1, attn_weights_block2
The Transformer Decoder is an essential part of the Transformer model, often used in tasks like machine translation, text generation, and more. Let’s break down the parts of the code that are new:
Attention layers
Two multi-head attention layers (mha1 and mha2) are defined. mha1 is used for self-attention within the decoder, and mha2 is used for attention over the encoder's output. The feed-forward network (ffn) follows a similar structure to the encoder.
The call Method:
The decoder processes its input through two multi-head attention layers. The first one (attn1) is self-attention with a look-ahead mask, and the second one (attn2) focuses on the encoder's output. This is followed by the feed-forward network. Each step involves dropout and normalization.
Step 3: Assembling the Transformer
Think of this step as assembling your orchestra. Each encoder and decoder layer is an instrument, and you're arranging them to create harmony.
Full Transformer Model:
class Transformer(tf.keras.Model):
def __init__(self, num_layers, d_model, num_heads, dff, input_vocab_size,
target_vocab_size, pe_input, pe_target, rate=0.1):
super(Transformer, self).__init__()
self.encoder = Encoder(num_layers, d_model, num_heads, dff,
input_vocab_size, pe_input, rate)
self.decoder = Decoder(num_layers, d_model, num_heads, dff,
target_vocab_size, pe_target, rate)
self.final_layer = tf.keras.layers.Dense(target_vocab_size)
def call(self, inp, tar, training, enc_padding_mask,
look_ahead_mask, dec_padding_mask):
enc_output = self.encoder(inp, training, enc_padding_mask)
dec_output, attention_weights = self.decoder(
tar, enc_output, training, look_ahead_mask, dec_padding_mask)
final_output = self.final_layer(dec_output)
from tensorflow.keras.layers import Input, Dense
from tensorflow.keras.models import Model
# Define input layers
input_ids = Input(shape=(None,), dtype='int32', name="input_ids")
attention_mask = Input(shape=(None,), dtype='int32', name="attention_mask")
# Load the pre-trained BERT model
bert = model(input_ids, attention_mask=attention_mask)
# Add a classification layer on top
x = bert.last_hidden_state[:, 0, :]
x = Dense(128, activation='relu')(x)
output = Dense(1, activation='sigmoid')(x)
# Construct the final model
fine_tuned_model = Model(inputs=[input_ids, attention_mask], outputs=[output])
# Compile the model
fine_tuned_model.compile(optimizer='adam', loss='binary_crossentropy', metrics=['accuracy'])
# Example labels for the sentences
labels = [1, 0] # 1 for positive, 0 for negative sentiment
# Train the model
fine_tuned_model.fit(inputs, labels, epochs=3, batch_size=32)
Conclusion
Creating an LLM from scratch is an intricate yet immensely rewarding process. By understanding and building upon the Transformer architecture with TensorFlow and Keras, and leveraging transfer learning through Hugging Face, you can create a model that's not just a powerful NLP tool but a reflection of your unique approach to understanding language.
As you continue on this journey, remember that the field of NLP is ever-evolving, and there's always more to learn and explore. Happy modeling!
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK