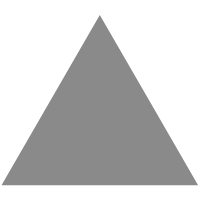
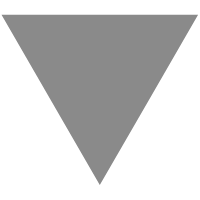
React Usecallback for Kids/Beginners
source link: https://dev.to/csituma/react-usecallback-for-kidsbeginners-18df
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
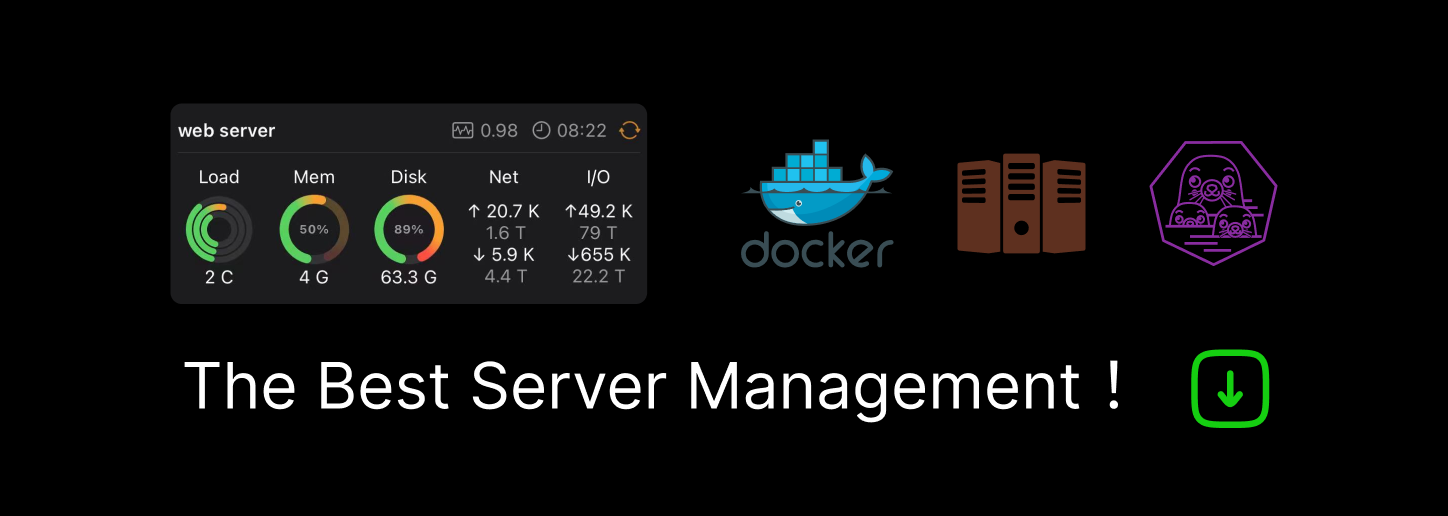
Let's say we're making a webpage where we want to draw a smiley face every time we click a button.
But drawing that smiley takes a lot of computer energy.
So, we tell React to remember how to draw a smiley face using useCallback.
Now, every time we click the button, React 'remembers' how to draw the smiley without using extra energy
import React, { useCallback } from 'react';
function SmileyComponent() {
// This is our "spell" for drawing a smiley
const drawSmiley = useCallback(() => {
console.log("😊");
}, []);
return (
<div>
{/* A button that, when clicked, will use the memorized drawSmiley function */}
<button onClick={drawSmiley}>Draw Smiley</button>
</div>
);
}
export default SmileyComponent;
Dependencies
Think of useCallback like a magic notebook for your robot friend that helps it remember how to do a task (like building a sandcastle) the best way possible, without wasting energy. But if something important changes (like the weather), the robot knows it needs to update its notebook with new instructions.
This way, your robot always builds the sandcastle perfectly, using the least amount of energy, and you both have more time to play and have fun at the beach!
import React from 'react';
const MyCustomButton = React.memo(({ onClick, children }) => {
console.log(`Rendering button: ${children}`);
return <button onClick={onClick}>{children}</button>;
});
import React, { useState, useCallback } from 'react';
function ColorBox() {
const [weather, setWeather] = useState('sunny'); // The weather condition
const [color, setColor] = useState('yellow'); // Color of the box
const updateColor = useCallback(() => {
switch (weather) {
case 'sunny':
setColor('yellow');
break;
case 'rainy':
setColor('gray');
break;
case 'windy':
setColor('blue');
break;
default:
setColor('green'); // Default color if weather is not recognized
}
}, [weather]); // The dependency array
return (
<div>
<h2>Weather: {weather}</h2>
<MyCustomButton onClick={() => setWeather('sunny')}>Sunny</MyCustomButton>
<MyCustomButton onClick={() => setWeather('rainy')}>Rainy</MyCustomButton>
<MyCustomButton onClick={() => setWeather('windy')}>Windy</MyCustomButton>
<MyCustomButton onClick={updateColor}>Update Box Color</MyCustomButton>
<div style={{ width: '100px', height: '100px', backgroundColor: color, marginTop: '20px' }}>
Box
</div>
</div>
);
}
export default ColorBox;
Conclusion
useCallback with dependencies is a smart way for things (like your robot or a computer program) to remember how to do something efficiently, only changing their plan when they really need to, based on what's different. !
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK