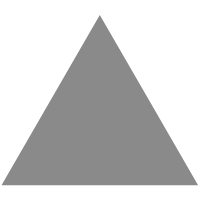
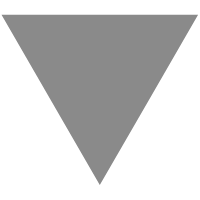
You’re better off using Exceptions – Eirik Tsarpalis' blog
source link: https://eiriktsarpalis.wordpress.com/2017/02/19/youre-better-off-using-exceptions/
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
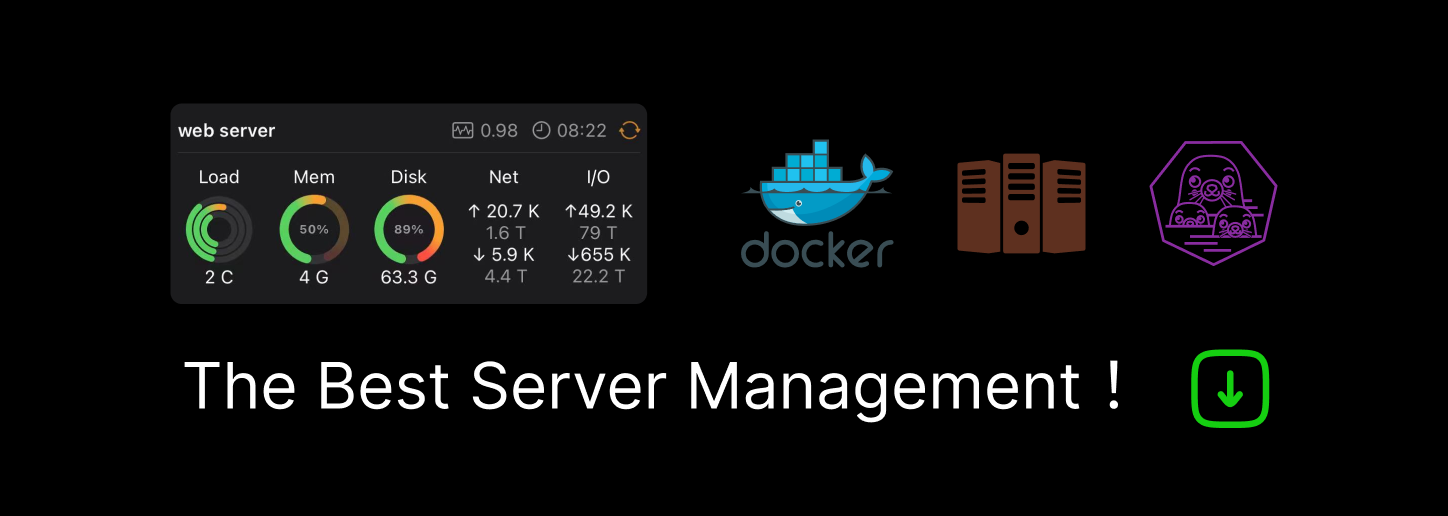
You’re better off using Exceptions
Exception handling is an error management paradigm that has often been met with criticism. Such criticisms typically revolve around scoping considerations, exceptions-as-control-flow abuse or even the assertion that exceptions are really just a type safe version of goto. To an extent, these seem like valid concerns but it is not within the scope of this article to address those per se.
Such concerns resonate particularly well within FP communities, often taken to the extreme: we should reject exceptions altogether, since code that throws is necessarily impure. In the F# community, this opinion is in part realized by advocating alternatives like result types and railway-oriented programming. In essence, these approaches follow the Either monad found in Haskell, but often intentionally avoiding the use of do notation/computation expressions (since that’s just interpreted exception semantics).
The TL;DR version of the approach is that we define a union type for results that looks like this:
type Result<'TSuccess, 'TError> = | Ok of 'TSuccess | Error of 'TError |
then require that any function which admits the possibility of error should return a Result
value. This way we are forcing the type system acknowledge error cases and makes our error handling logic more explicit and predictable. We can also use combinators like bind
to encode common error handling flows.
I would like to illustrate in this article why I believe that this often causes a lot of problems — while simultaneously not achieving the advertised benefits, particularly in the context of maintaining large codebases. It also gives rise to a multitude of anti-patterns, particularly when used by people new to F#.
An issue of Runtime
Most programming languages out there carry the concept of a runtime error. Such errors sometimes stem from unexpected locations, in code otherwise considered pure. In Haskell for instance, consider what the pure expressions “2 `div` 0
” or “head []
” evaluate to. In the case of the CLR, runtime errors are manifested in the form of thrown exceptions, which arguably happens more often than Haskell.
Because runtime errors are difficult to anticipate, I claim that using result types as a holistic replacement for error handling in an application is a leaky abstraction. Consider the following example:
type Customer = { Id : string; Credit : decimal option } let average (customers : Customer list) = match customers with | [] -> Error "list was empty" | _ -> customers |> List.averageBy ( fun c -> c.Credit.Value) |> Ok |
Notice that the above snippet now admits two possible classes of errors, string
and the potential NullReferenceException
admitted by careless access of the optional field in the customer record. This eventually delivers an unpleasant surprise to consumers of the function, since the type signature communicates an exception-free implementation.
An Awkward Reconciliation
It’s by such misadventure that the working F# programmer soon realizes that any function could still potentially throw. This is an awkward realization, which has to be addressed by catching as soon as possible. This of course is the purely functional equivalent of the Pokemon anti-pattern:
let avg : Result<decimal, string> = try average customers with e -> Error e.Message // gotta catch em all! |
Boilerplate
In the majority of codebases using result types that I’ve been reviewing, people typically just end up re-implementing exception semantics on an ad-hoc basis. This can result in extremely noisy code, as illustrated in the following example:
let combine x y z = match x, y, z with | Ok x, Ok y, Ok z -> Ok (x,y,z) | Error e, _, _ | _, Error e, _ | _, _, Error e -> Error e |
or in using pre-baked combinators
let combine x y z = x |> bind ( fun x -> y |> bind ( fun y -> z |> bind ( fun z -> Ok(x,y,z)))) |
We really shouldn’t be doing this. It is essentially the type equivalent of using a linter that demands inserting catch-alls and rethrows in every method body.
Given sufficient implementation complexity, things can get really ugly: binding on results of differing error types and other incidental complexities require substantial refactoring to get things right. Often, this will prompt developers to cut corners by doing unexpected things, like inserting a throw just to get rid of an annoying error branch or returning nested result types like
Result<Result<string, string>, string list> |
Where’s my Stacktrace?
An important property of exceptions -which cannot be stressed enough- is that they are entities managed and understood by the underlying runtime, endowed with metadata critical to diagnosing bugs in complex systems. They can also be tracked and highlighted by tooling such as debuggers and profilers, providing invaluable insight when probing a large system.
By lifting all of our error handling to passing result values, we are essentially discarding all that functionality. There is nothing worse than having to deal with a production issue which comes up in the logs simply as "list was empty"
.
The Problem with IO
The points discussed thus far have typically focused on applications that might as well have been purely functional. As might be expected, these become even more pronounced when bringing in IO or when interfacing with third-party .NET libraries. Consider the following example:
let tryReadAllText (path : string) : string option = try System.IO.File.ReadAllText path |> Some with _ -> None |
I have spotted this approach and variations in many production-grade F# codebases, and have been responsible for some of these myself back when I was younger and more naive. This adapter is motivated by the desire to have an exception-free file reader, however it is deeply flawed precisely because of that.
While it is fairly unambiguous what a Some
result means, the None
bit hardly conveys any information other than the obvious. Here is an official list of possible errors that can be raised by that particular call. By discarding the exception, it becomes impossible to diagnose what could have gone wrong.
Stringly-typed error handling
One could quickly point out that the snippet above can be amended so that data loss is minimized.
let readAllText (path : string) : Result<string, string> = try System.IO.File.ReadAllText path |> Ok with e -> Error e.Message |
This alteration still makes error handling awkward and unsafe
match readAllText "foo.txt" with | Error e when e.Contains "Could not find file" -> // ... |
particularly when compared to how the idiomatic approach works
try File.ReadAllText path with :? FileNotFoundException -> // ... |
Interop issues
It is often easy to forget that Result
values are plain objects, with no particular bearing when used in the context of other frameworks/libraries. The following example illustrates an innocent mistake when working with TPL:
let task = Task.StartNew( fun () -> if Random.Next() % 2 = 0 then Ok () else Error ()) task.Wait() if task.IsFaulted then printfn "Task failed" else printfn "Task succeeded" |
Or perhaps it could be mistakenly believed that F# Async somehow recognizes result types
let job1 = async { return Ok () } let job2 = async { return Error () } let ! result = [job1 ; job2] |> Async.Parallel |> Async.map Ok match result with | Ok _ -> printfn "All jobs completed successfully" | Error _ -> printfn "Some of the jobs failed" |
Conclusions
It is not in the intentions of this article to argue that result types shouldn’t be used at all. I have written DSLs and interpreters using result types. MBrace uses result types internally. In most cases though, the benefit is only maximized by writing custom result types that model a particular domain. Rather than having a general-purpose error branch that encodes infinite classes of failures, assign each class of errors to an individual branch in that result type:
type MoneyWithdrawalResult = | Success of amount:decimal | InsufficientFunds of balance:decimal | CardExpired of DateTime | UndisclosedFailure |
This approach constrains error classes in a finite domain, which also allows for more effective testing of our code.
That said, I strongly believe that using result types as a general-purpose error handling mechanism for F# applications should be considered harmful. Exceptions should remain the dominant mechanism for error propagation when programming in the large. The F# language has been designed with exceptions in mind, and has achieved that goal very effectively.
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK