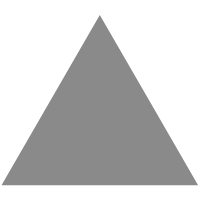
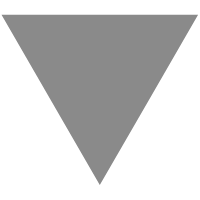
在 Create React App 中使用 TypeScript,你学会了吗?
source link: https://www.51cto.com/article/780652.html
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
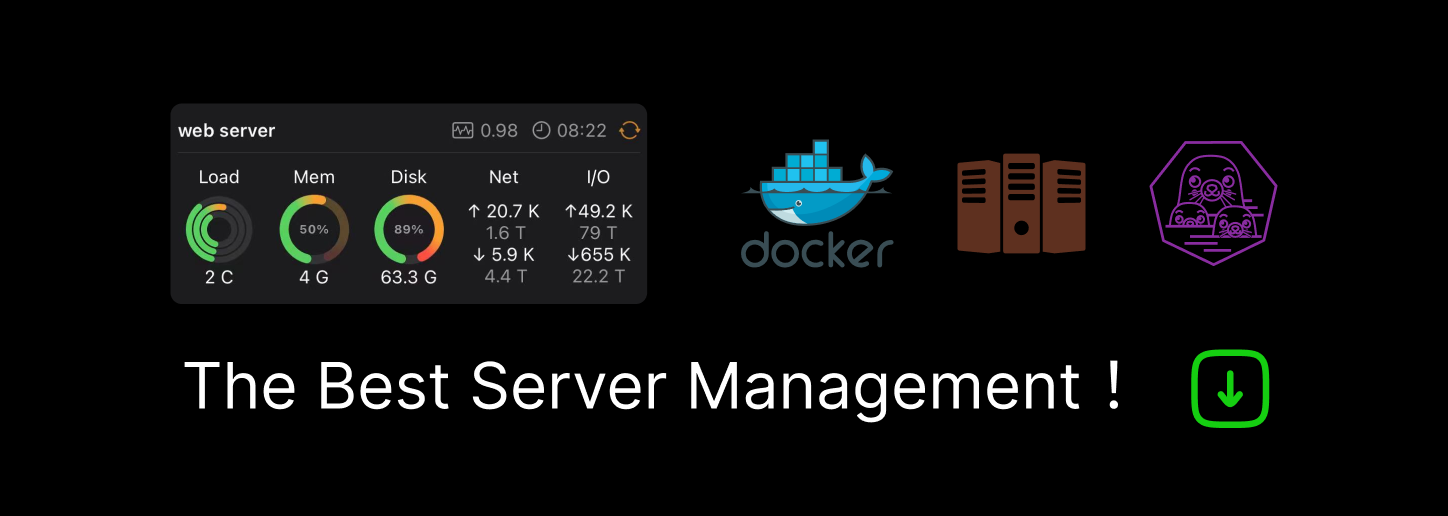
在React项目中引入TypeScript(TS)涉及到一系列配置步骤。以下是一个完整的配置过程,从创建React项目到配置TypeScript:
1. 需要创建一个使用 TypeScript 的新项目
首先,你可以使用create-react-app工具来创建一个React结合TypeScript的项目。Create React App 内置了对 TypeScript 的支持。在命令行中运行以下命令:
npx create-react-app my-app --template typescript
这将创建一个名为my-react-app的React项目,并安装默认的配置。
2. 进入项目目录:
cd my-react-app
3. 已有的项目添加TyoeScript:
npm install --save typescript @types/node @types/react @types/react-dom @types/jest
4. 重命名文件并修改后缀:
将项目目录下的src/App.js文件重命名为src/App.tsx,这样React就能识别它是一个TypeScript文件。
5. 修改 src/index.tsx 文件:
将src/index.js文件中的内容改为:
import React from 'react';
import ReactDOM from 'react-dom';
import './index.css';
import App from './App';
ReactDOM.render(
<React.StrictMode>
<App />
</React.StrictMode>,
document.getElementById('root')
);
6. 配置 tsconfig.json 文件:
没有配置项,编译器提供不了任何帮助。在 TypeScript 里,这些配置项都在一个名为 tsconfig.json 的特殊文件中定义。可以通过执行以下命令生成该文件:使用 Yarn,执行:
yarn run tsc --init
使用 npm,执行:
npx tsc --init
在 tsconfig.json 文件里面添加以下基本配置:
{
"compilerOptions": {
"target": "es5",
"lib": ["dom", "dom.iterable", "esnext"],
"allowJs": true,
"skipLibCheck": true,
"esModuleInterop": true,
"allowSyntheticDefaultImports": true,
"strict": true,
"forceConsistentCasingInFileNames": true,
"module": "esnext",
"moduleResolution": "node",
"resolveJsonModule": true,
"isolatedModules": true,
"noEmit": true,
"jsx": "react-jsx"
},
"include": ["src/**/*.ts", "src/**/*.tsx"],
"exclude": ["node_modules"]
}
这个 tsconfig.json 文件包含了基本的TypeScript配置。你可以根据项目的需求进行调整。更多配置请参考文档
7. 修改 src/App.tsx 文件:
在 src/App.tsx 文件中,可以使用 TypeScript 的语法,例如声明组件的 props 类型和状态类型:
import React, { useState } from 'react';
interface AppProps {
message: string;
}
const App: React.FC<AppProps> = ({ message }) => {
const [count, setCount] = useState<number>(0);
return (
<div>
<h1>{message}</h1>
<p>Count: {count}</p>
<button onClick={() => setCount(count + 1)}>Increment</button>
</div>
);
}
export default App;
这里使用了 TypeScript 的 interface 来定义 App 组件的 props 类型,以及使用 useState 声明了 count 的状态类型。
8.文件扩展名在 React 中,组件文件大多数使用 .js 作为扩展名。在 TypeScript 中,提供两种文件扩展名:
.ts 是默认的文件扩展名,而 .tsx 是一个用于包含 JSX 代码的特殊扩展名。
9.类型定义如果你想要显示来自其他包(libraries)的错误和提示,通常你需要安装相应库的 TypeScript 类型声明。TypeScript 类型声明文件的后缀为 .d.ts,它包含了有关库的类型信息,使得 TypeScript 编译器能够理解和验证你对库的使用。
以下是一些常见情况下可能需要安装的 TypeScript 类型声明的例子:
React 类型声明:
npm install --save @types/react @types/react-dom
如果你使用了 React,这个命令将安装 React 和 ReactDOM 的类型声明文件。
其他 npm 包的类型声明:
对于其他可能使用的库,你可以查看它们的 npm 包是否有相应的 @types 包。例如,如果你使用了 axios,可以运行:
npm install --save @types/axios
声明文件不可用的情况:
有时,某些包可能没有官方的 TypeScript 类型声明文件,或者它们可能不是最新的。在这种情况下,你可能需要使用类型声明文件生成工具,例如 tsc(TypeScript 编译器)的 --allowJs 和 --declaration 选项,以从 JavaScript 代码生成类型声明文件。但请注意,这可能不如官方的类型声明文件准确和全面。
确保你的项目中包含了所需的类型声明文件后,TypeScript 编译器就能够正确地检查和验证你对这些库的使用,以及在开发过程中显示相关的错误和提示信息。
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK