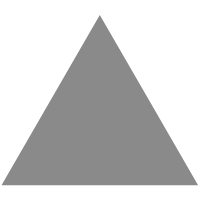
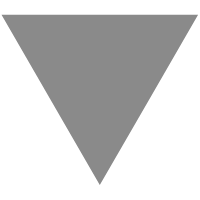
3 fundamental concepts in Javascript OOP
source link: https://dev.to/junlow/3-fundamental-concepts-in-javascript-oop-12fn
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
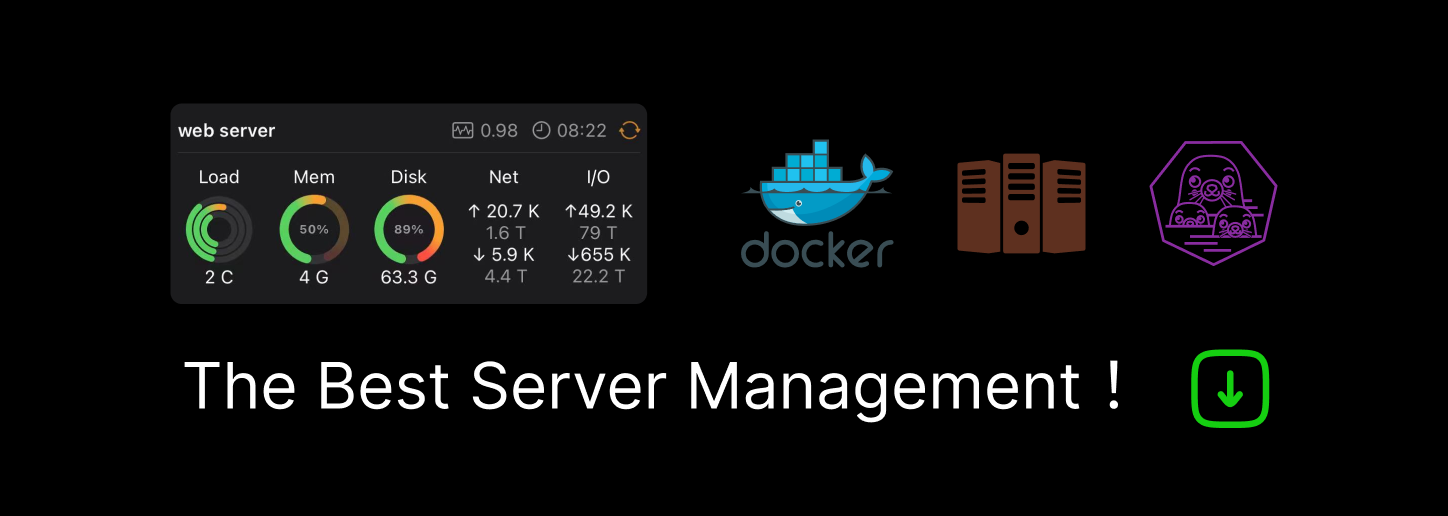
Hi beautiful people 👋 Ever feel like your codes are a jumbled mess of spaghetti functions?
Yeah, me too.
That's where object-oriented programming (OOP) comes in - one of its most powerful tools for crafting organized, reusable code in programming.
Think of it as building LEGOs for your apps. Each object is a brick, with its own data and superpowers (methods). Snap them together, and suddenly you've got a modular masterpiece that's easy to maintain and tweak ✨ OOP brings that same structure to our code, making it more modular, reusable, and easier to understand.
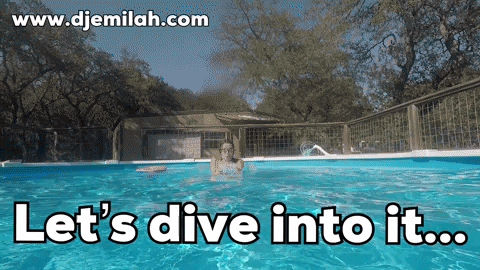
OOP concepts in action
Encapsulation
This is about bundling data (attributes) and methods (functions) that operate on the data into a single unit, known as a class. It helps in hiding the internal details of how an object works.
class Dog {
constructor(name) {
this.name = name;
}
bark() {
console.log(`${this.name} says Woof!`);
}
}
const myDog = new Dog('Nicky');
myDog.bark(); // Nicky says Woof!
Inheritance
This concept allows a class to inherit properties and methods from another class. It promotes code reuse and establishes a relationship between the parent (superclass) and child (subclass) classes.
class Animal {
constructor(name) {
this.name = name;
}
makeSound() {
console.log("Generic animal sound");
}
}
class Dog extends Animal {
bark() {
console.log(`${this.name} says Woof!`);
}
}
class Cat extends Animal {
meow() {
console.log(`${this.name} says Meow!`);
}
}
const myDog = new Dog("Nicky");
myDog.makeSound(); // Generic animal sound (inherited from Animal)
myDog.bark(); // Nicky says Woof!
const myCat = new Cat("Milo");
myCat.makeSound(); // Generic animal sound (inherited from Animal)
myCat.meow(); // Milo says Meow!
Polymorphism
This refers to the ability of objects to take on multiple forms. In JavaScript, this often involves using interfaces or method overloading to create flexible and interchangeable components.
interface AnimalSound {
makeSound(): void;
}
class Dog implements AnimalSound {
// ... (same as before)
}
class Cat implements AnimalSound {
// ... (same as before)
}
function makeAnimalSound(animal: AnimalSound) {
animal.makeSound();
}
const myDog = new Dog("Nicky");
const myCat = new Cat("Milo");
makeAnimalSound(myDog); // Nicky says Woof!
makeAnimalSound(myCat); // Milo says Meow!
Resources for OOP Enlightenment 🌐
Thanks for reading! I hope you find these resources as helpful as I did. Feel free to share your thoughts and keep that curiosity alive 😃 Happy coding! 🚀🌟
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK