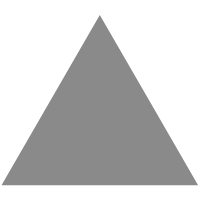
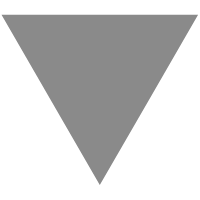
"VS Code doesn't support bits/stdc++.h" Solution
source link: https://codeforces.com/blog/entry/118427
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
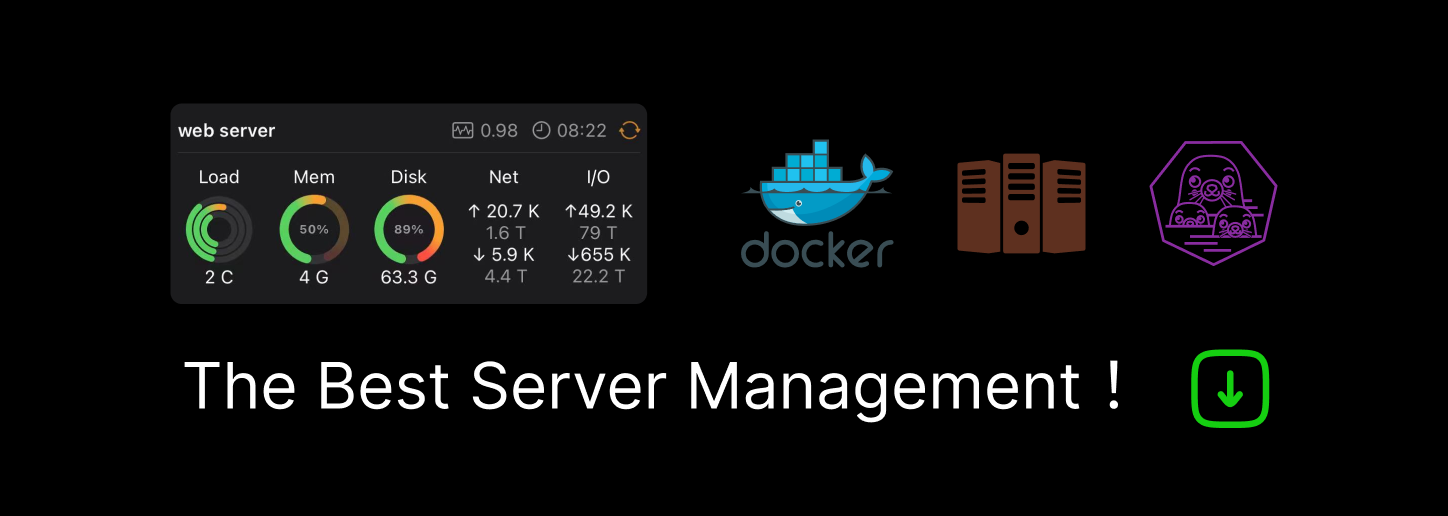
If you are using Visual Studio Code (VS Code) for solving C++ problems on platforms like Codeforces and you encounter issues with #include <bits/stdc++.h>
not being supported, here's a simple solution to get it working.
Create a
bits
folder: Start by creating a new folder named 'bits' in the same directory where your main C++ files for problem-solving are located.Create a header file: Inside the
bits
folder, create a new header file namedstdc++.h
. Make sure the extension of the file is.h
to create a valid header file.Add necessary headers: Open the
stdc++.h
file and copy-paste the following commonly used C++ standard library headers into it:
#include <iostream> // Input/output stream objects
#include <fstream> // File stream objects
#include <sstream> // String stream objects
#include <iomanip> // Input/output manipulators
#include <string> // String class and functions
#include <vector> // Dynamic array
#include <list> // Doubly linked list
#include <set> // Set container
#include <map> // Map container
#include <queue> // Queue container
#include <stack> // Stack container
#include <algorithm> // Algorithms on sequences (e.g., sort, find)
#include <cmath> // Mathematical functions
#include <ctime> // Date and time functions
#include <cstdlib> // General purpose functions (e.g., memory management)
#include <cstring> // C-style string functions
#include <cctype> // Character classification functions
#include <cassert> // Assert function for debugging
#include <exception> // Standard exceptions
#include <functional> // Function objects
#include <iterator> // Iterator classes
#include <limits> // Numeric limits
#include <locale> // Localization and internationalization
#include <numeric> // Numeric operations (e.g., accumulate)
#include <random> // Random number generators
#include <stdexcept> // Standard exception classes
#include <typeinfo> // Runtime type information
#include <utility> // Utility components (e.g., std::pair)
- Include the header: Now, you can include the 'bits/stdc++.h' header in your main C++ files as you normally would:
#include <bits/stdc++.h>
That's it! You have successfully set up #include <bits/stdc++.h>
in VS Code for seamless C++ problem-solving.
Note: Keep in mind that these libraries cover the most commonly used functionalities. If you encounter additional libraries while coding, feel free to add them to the stdc++.h
file to ensure you have access to all the necessary tools.
Happy coding and good luck in your coding journey! :)
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK