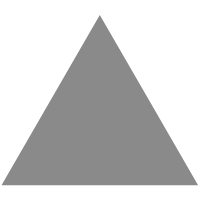
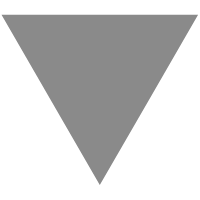
Web Tools #546 - MediaCapture, CSS/HTML Tools, SVG, Uncats
source link: https://mailchi.mp/webtoolsweekly/web-tools-546
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
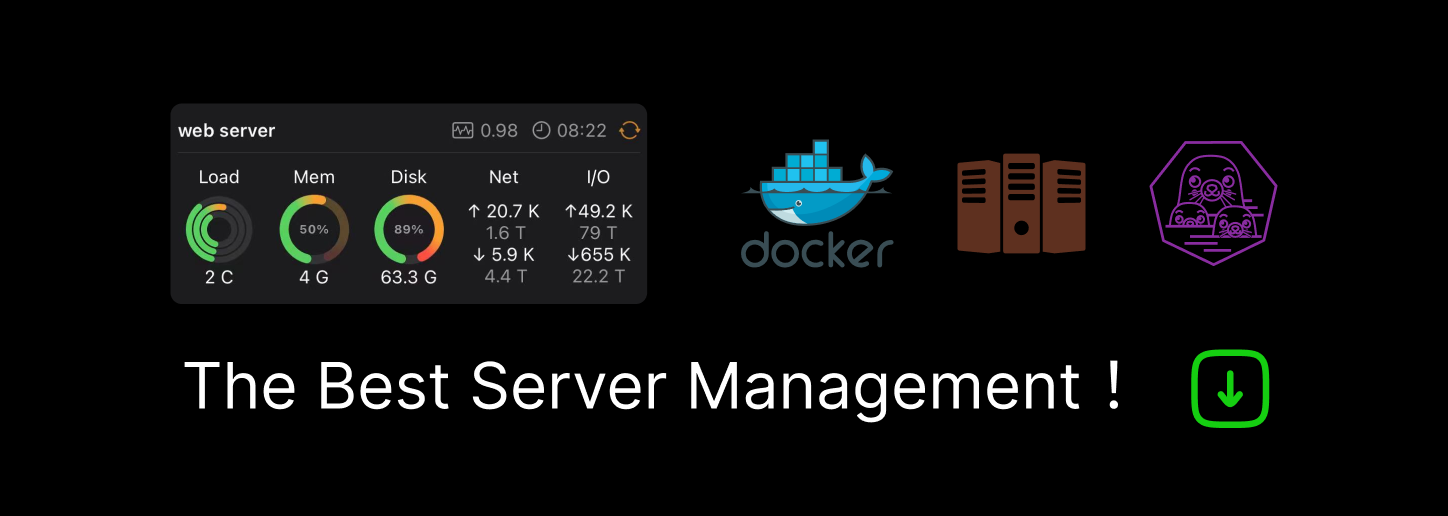
MediaCapture, CSS/HTML Tools, SVG, Uncats
|
Issue #546 • January 4, 2024
Advertisement
|
Build with the Power of Code — Without Writing Any Take control of HTML, CSS, and JavaScript in a visual canvas. Webflow generates clean, semantic code that’s ready to publish or hand to developers. |
It's amazing how far the Web APIs have come and how much we can now do with just a little bit of JavaScript. One example of this is the Media Capture API, which allows you to capture part or all of a screen for things like streaming, recording, or sharing during a WebRTC conference.
The above example uses the await operator in conjunction with the .getDisplayMedia() method of the mediaDevices interface. A MediaRecorder() constructor is also used, after which the recording session is initiated using the .start() method.
During the streaming process, the code listens for the "ended" event to trigger a stop to the recording process.
This creates an anchor (link) element that doesn't exist on the page but technically can still be manipulated. The link.click() method triggers a virtual "visit" to the link that's created, but since it has the "download" attribute present instead of a standard href, the file gets downloaded. |
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK