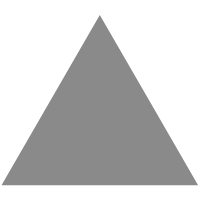
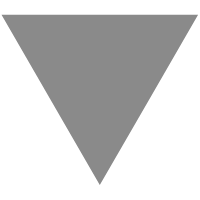
PHP Program to Find LCM of Two Numbers
source link: https://www.geeksforgeeks.org/php-program-to-find-lcm-of-two-numbers/
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
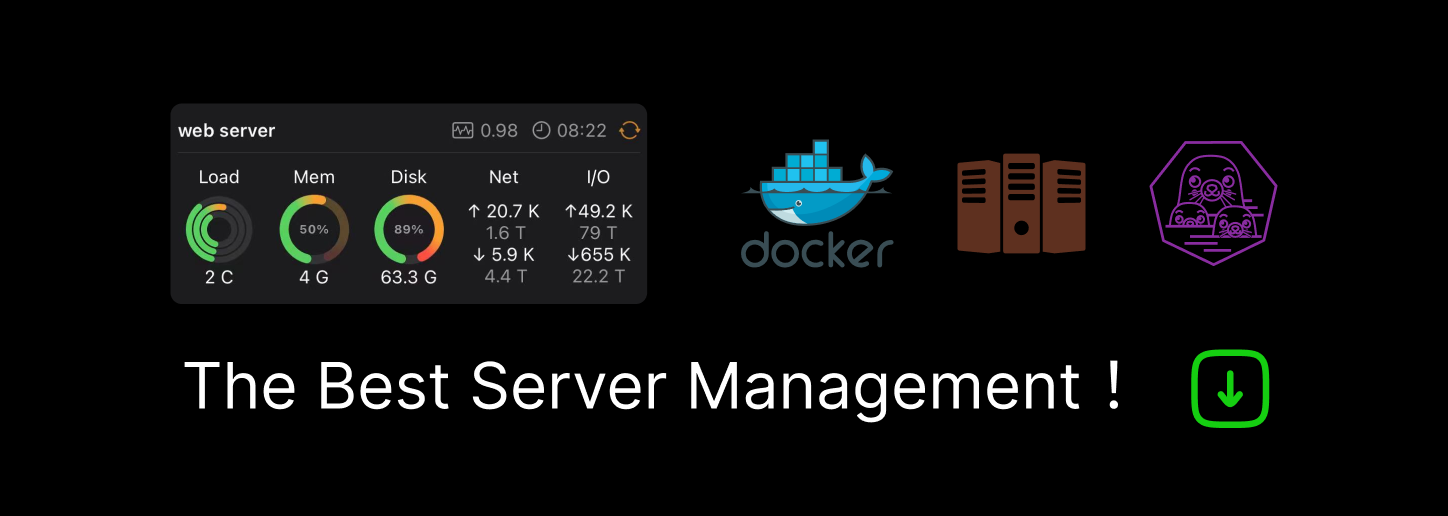
PHP Program to Find LCM of Two Numbers
Given two numbers, the task is to find the LCM of two numbers using PHP.
Examples:
Input: num1 = 25, num2 = 5
Output: LCM = 25
Input: num1 = 36, num2 = 72
Output: LCM = 72
There are two methods to find the LCM of two numbers, these are:
Using Mathematical Formula
In this section, we use mathematical formula to calculate the LCM of two numbers. The LCM of two numbers is equal to the product of the two numbers divided by their Greatest Common Divisor (GCD).
Example:
<?php function findGCD( $a , $b ) { while ( $b != 0) { $temp = $b ; $b = $a % $b ; $a = $temp ; } return $a ; } function findLCM( $a , $b ) { $gcd = findGCD( $a , $b ); $lcm = ( $a * $b ) / $gcd ; return $lcm ; } $num1 = 12; $num2 = 18; echo "LCM : " . findLCM( $num1 , $num2 ); ?> |
LCM : 36
Using Iteration
In this approach, iteratively checks multiples of the larger number until it finds the first multiple that is also divisible by the smaller number.
Example:
<?php function findLCM( $a , $b ) { $max = max( $a , $b ); $min = min( $a , $b ); $multiple = $max ; while ( $multiple % $min != 0) { $multiple += $max ; } return $multiple ; } $num1 = 12; $num2 = 18; echo "LCM : " . findLCM( $num1 , $num2 ); ?> |
LCM : 36
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK