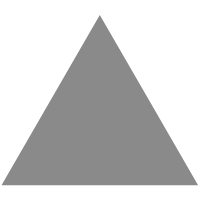
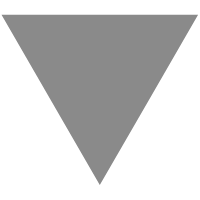
Spring MVC异常处理:这几种方式让你轻松应对,你都使用了那些方式?
source link: https://www.51cto.com/article/768482.html
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
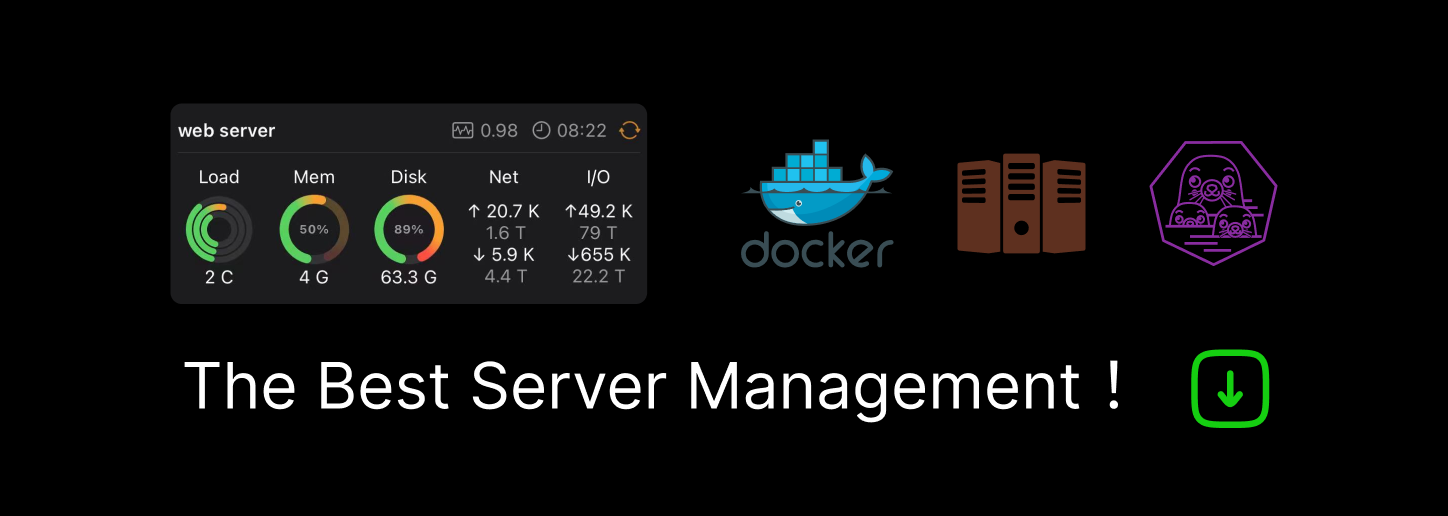
Spring MVC异常处理:这几种方式让你轻松应对,你都使用了那些方式?
环境:Spring5.3.23
Spring MVC提供了灵活的异常处理机制,可以让开发者方便地处理应用程序中发生的各种异常。Spring MVC的异常处理主要依赖于Spring的@ControllerAdvice和@ExceptionHandler注解。
@ControllerAdvice: 该注解用于定义一个全局的异常处理类,可以处理所有@RequestMapping方法中抛出的异常。例如,你可以创建一个全局的异常处理类,来处理所有的异常。
@ExceptionHandler: 该注解用于指定需要处理的异常类型。在全局异常处理类中,你可以使用@ExceptionHandler注解来指定需要处理的异常类型。例如,你可以创建一个全局的异常处理类,来处理所有的Exception异常。
现在基本上大部分项目都是前后端分离,API接口都是基于Restful。所以在项目中我们主要使用的是@RestControllerAdvice该注解与@ControllerAdvice主要区别其实就是Rest的注解中多了一个@ResponseBody 注解(将方法的返回值,以特定的格式写入到response的body,进而将数据返回给客户端,如果是字符串直接输出字符串信息,如果是对象将会把对象转为json进行输出)。
部分源码:
@Component
public @interface ControllerAdvice {
}
@ControllerAdvice
@ResponseBody
public @interface RestControllerAdvice {
}
2. 应用案例
Controller内部处理异常
@RestController
public class TestController {
@GetMapping("/test/{id}")
public Object test(@PathVariable Integer id) {
if (id < 5) {
throw new RuntimeException("运行时异常") ;
}
return "测试异常处理" ;
}
@ExceptionHandler
public Object handle(Exception e) {
return e.getMessage() ;
}
}
这样如果这个Controller中的接口发生了异常那么就会执行有@ExceptionHandler(当前还得根据异常进行匹配)标注的方法。
该种方式处理异常只是针对当前Controller,一个项目肯定会有很多的Controller,如果每一个类都这样处理明显是太麻烦,而且还不方便统一异常的处理。
全局异常处理
可以在一个类上添加 @RestControllerAdvice或@ControlerAdvice
@RestControllerAdvice
public class TestControllerAdvice {
@ExceptionHandler
public Object handle(Exception e) {
return "我是全局异常:" + e.getMessage() ;
}
}
到此全局异常的使用方式就结束了当你访问接口时你会发现全局异常没有起作用。
当我们把controller中的@ExceptionHandler注释了,这时全局异常才会生效。
结论:局部异常处理优先级高于全局异常处理。
以上是项目中如果使用异常处理句柄的方式;接下来我们来看看在全局异常处理句柄中如何进行局部控制(比如只处理有特定注解的或是只处理部分controller又或者是指定包下的controller)。
只处理特定注解
自定义Annotation:
@Target(ElementType.TYPE)
@Retention(RetentionPolicy.RUNTIME)
@Documented
public @interface AppAnnotation {
}
Controller类:
有@AppAnnotation注解的Controller
@AppAnnotation
@RestController
public class AnnotationController {
@GetMapping("/an/get/{id}")
public Object an(@PathVariable Integer id) {
if (id < 10) {
throw new RuntimeException("发生错误了") ;
}
return "自定义Annotation注解: " + id ;
}
}
没有@AppAnnotation注解的Controller
@RestController
public class AnnotationController2 {
@GetMapping("/an/get2/{id}")
public Object an(@PathVariable Integer id) {
if (id < 10) {
throw new RuntimeException("2发生错误了") ;
}
return "自定义Annotation注解2: " + id ;
}
}
ControllerAdvice异常处理类:
@RestControllerAdvice(annotations = {AppAnnotation.class})
public class AnnotationControllerAdvice {
@ExceptionHandler
public Object handle(Exception e) {
return "特定注解全局异常:" + e.getMessage() ;
}
}
分别访问/an/get/1 和/an/get2/1接口,只有有@AppAnnotation注解的Controller会被处理。
只处理指定的Controller
新建UserController
@RestController
public class UserController {
@GetMapping("/user/{id}")
public Object get(@PathVariable Integer id) {
if (id < 10) {
throw new RuntimeException("用户ID错误") ;
}
return "Users" ;
}
}
新建PersonController
@RestController
public class PersonController {
@GetMapping("/person/{id}")
public Object get(@PathVariable Integer id) {
if (id < 10) { throw new RuntimeException("Person ID错误") ;
}
return "Person" ;
}
}
全局异常处理类:
@RestControllerAdvice(assignableTypes = {UserController.class})
public class SpecificControllerAdvice {
@ExceptionHandler
public Object handle(Exception e) {
return "指定Controller全局异常:" + e.getMessage() ;
}
}
这里通过assignableTypes属性来限定了只有UserController类发生了异常才会做出响应。
PersonController发生异常不会被处理。
指定包下的Controller
@RestControllerAdvice(basePackages = {"com.pack.pkg1"})
public class PackageControllerAdvice {
@ExceptionHandler
public Object handle(Exception e) {
return "指定包下的全局异常:" + e.getMessage() ;
}
}
UserController类位于pkg1包下:
package com.pack.pkg1;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.PathVariable;
import org.springframework.web.bind.annotation.RestController;
@RestController("userPController")
public class UserController {
@GetMapping("/userp/{id}")
public Object get(@PathVariable Integer id) {
if (id < 10) {
throw new RuntimeException("用户ID错误") ;
}
return "Users" ;
}
}
PersonController类位于pkg2包下:
package com.pack.pkg2;
@RestController("personPController")
public class PersonController {
@GetMapping("/personp/{id}")
public Object get(@PathVariable Integer id) {
if (id < 10) {
throw new RuntimeException("Person ID错误") ;
}
return "Person" ;
}
}
当访问com.pack.pkg1包下的接口出现异常后就会被处理。
完毕!!!
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK