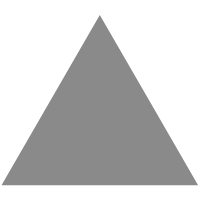
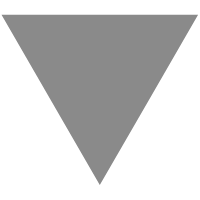
Check if all values in a Map are Equal in C++
source link: https://thispointer.com/check-if-all-values-in-a-map-are-equal-in-c/
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
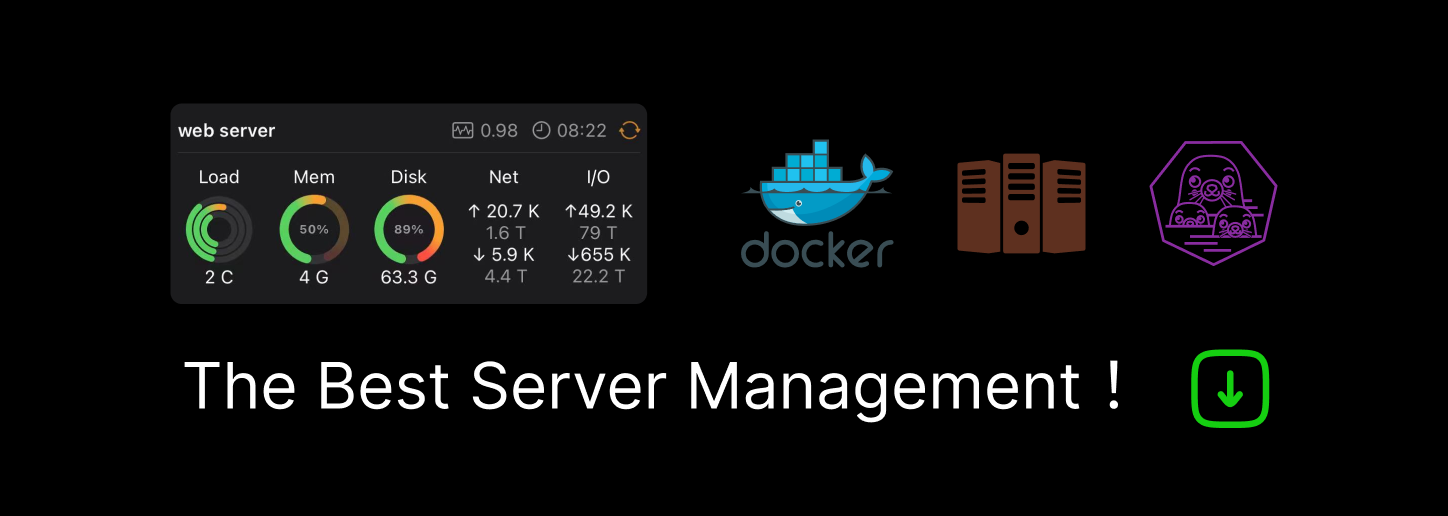
Check if all values in a Map are Equal in C++
This tutorial will discuss how to check if all values in a map are equal in C++.
To check if all the values in a map are equal or not, we can first fetch the value of the first key-value pair in the map, and then we can compare this value with all the remaining key-value pairs in the map. If this value matches with all the key-value pairs’ value field, then it means all the values in the map are equal.
We have created a generic function for this.
template <typename Key, typename Value> bool allValuesEqual( const std::map<Key, Value> &mapObj) { bool result = !mapObj.empty(); if (result) { const Value &firstValue = mapObj.begin()->second; for (const auto &pair : mapObj) { if (pair.second != firstValue) { result = false; } } } return result; }
It accepts a map of any type as an argument and returns true if all the values in the map are equal.
Let’s see the complete example,
#include <iostream> #include <map> template <typename Key, typename Value> bool allValuesEqual( const std::map<Key, Value> &mapObj) { bool result = !mapObj.empty(); if (result) { const Value &firstValue = mapObj.begin()->second; for (const auto &pair : mapObj) { if (pair.second != firstValue) { result = false; } } } return result; } int main() { std::map<std::string, int> wordFrequency{ {"this", 22}, {"why", 22}, {"what", 22}, {"how", 22}}; if (allValuesEqual(wordFrequency)) { std::cout << "All values in Map are equal." << std::endl; } else { std::cout << "Values in Map are not all equal." << std::endl; } return 0; }
Output
All values in Map are equal.
Summary
Today, we learned how to check if all values in a map are equal in C++.
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK