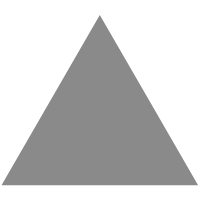
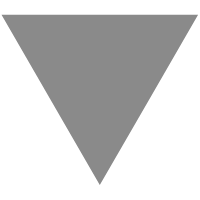
API with NestJS #115. Database migrations with Prisma
source link: https://wanago.io/2023/07/03/api-nestjs-prisma-migrations/
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
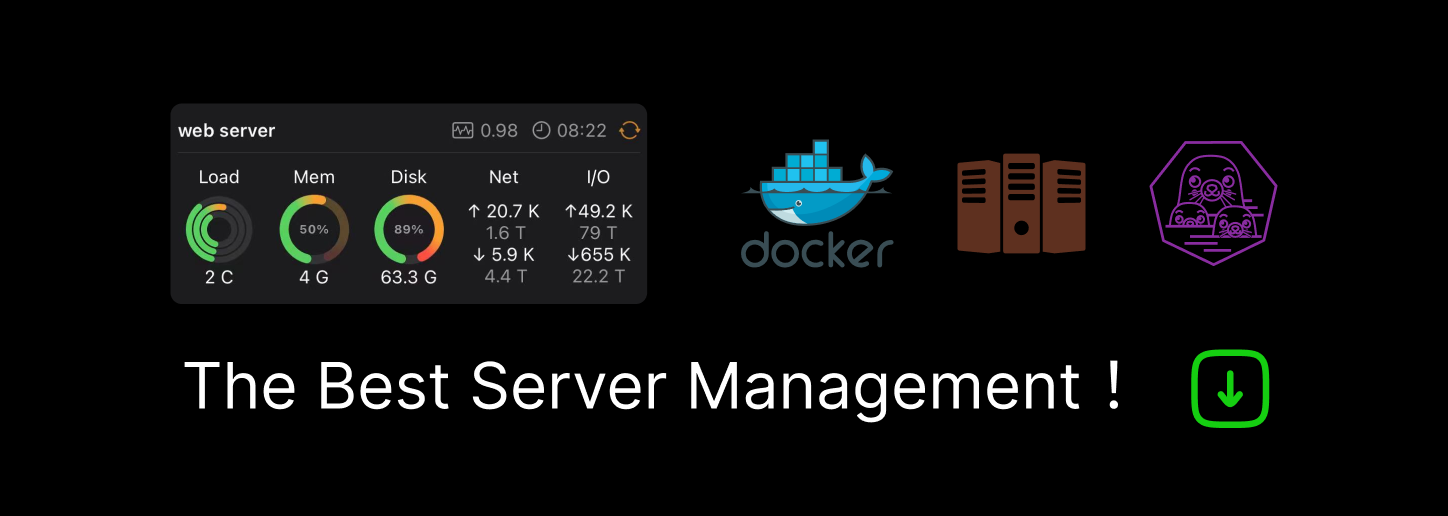
API with NestJS #115. Database migrations with Prisma
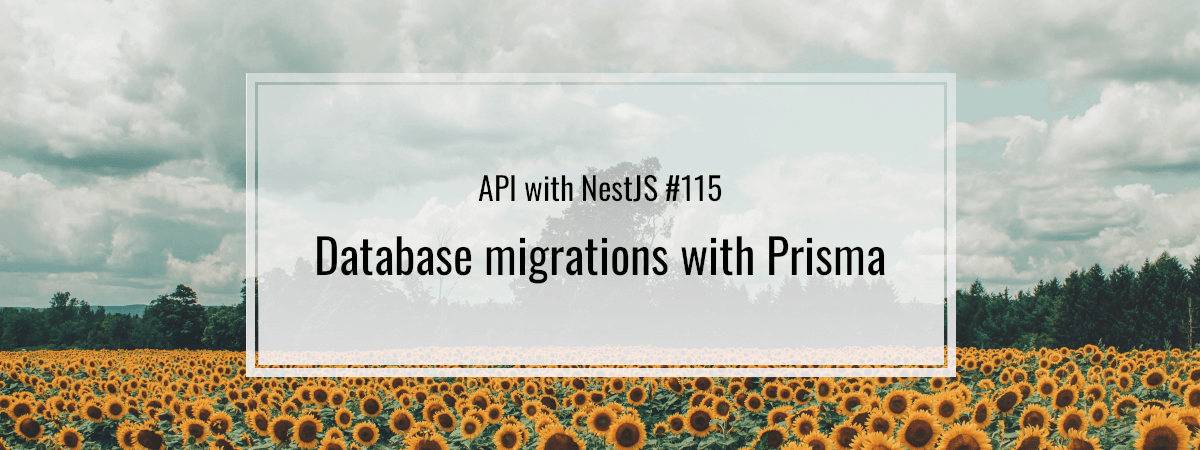
- 1. API with NestJS #1. Controllers, routing and the module structure
- 2. API with NestJS #2. Setting up a PostgreSQL database with TypeORM
- 3. API with NestJS #3. Authenticating users with bcrypt, Passport, JWT, and cookies
- 4. API with NestJS #4. Error handling and data validation
- 5. API with NestJS #5. Serializing the response with interceptors
- 6. API with NestJS #6. Looking into dependency injection and modules
- 7. API with NestJS #7. Creating relationships with Postgres and TypeORM
- 8. API with NestJS #8. Writing unit tests
- 9. API with NestJS #9. Testing services and controllers with integration tests
- 10. API with NestJS #10. Uploading public files to Amazon S3
- 11. API with NestJS #11. Managing private files with Amazon S3
- 12. API with NestJS #12. Introduction to Elasticsearch
- 13. API with NestJS #13. Implementing refresh tokens using JWT
- 14. API with NestJS #14. Improving performance of our Postgres database with indexes
- 15. API with NestJS #15. Defining transactions with PostgreSQL and TypeORM
- 16. API with NestJS #16. Using the array data type with PostgreSQL and TypeORM
- 17. API with NestJS #17. Offset and keyset pagination with PostgreSQL and TypeORM
- 18. API with NestJS #18. Exploring the idea of microservices
- 19. API with NestJS #19. Using RabbitMQ to communicate with microservices
- 20. API with NestJS #20. Communicating with microservices using the gRPC framework
- 21. API with NestJS #21. An introduction to CQRS
- 22. API with NestJS #22. Storing JSON with PostgreSQL and TypeORM
- 23. API with NestJS #23. Implementing in-memory cache to increase the performance
- 24. API with NestJS #24. Cache with Redis. Running the app in a Node.js cluster
- 25. API with NestJS #25. Sending scheduled emails with cron and Nodemailer
- 26. API with NestJS #26. Real-time chat with WebSockets
- 27. API with NestJS #27. Introduction to GraphQL. Queries, mutations, and authentication
- 28. API with NestJS #28. Dealing in the N + 1 problem in GraphQL
- 29. API with NestJS #29. Real-time updates with GraphQL subscriptions
- 30. API with NestJS #30. Scalar types in GraphQL
- 31. API with NestJS #31. Two-factor authentication
- 32. API with NestJS #32. Introduction to Prisma with PostgreSQL
- 33. API with NestJS #33. Managing PostgreSQL relationships with Prisma
- 34. API with NestJS #34. Handling CPU-intensive tasks with queues
- 35. API with NestJS #35. Using server-side sessions instead of JSON Web Tokens
- 36. API with NestJS #36. Introduction to Stripe with React
- 37. API with NestJS #37. Using Stripe to save credit cards for future use
- 38. API with NestJS #38. Setting up recurring payments via subscriptions with Stripe
- 39. API with NestJS #39. Reacting to Stripe events with webhooks
- 40. API with NestJS #40. Confirming the email address
- 41. API with NestJS #41. Verifying phone numbers and sending SMS messages with Twilio
- 42. API with NestJS #42. Authenticating users with Google
- 43. API with NestJS #43. Introduction to MongoDB
- 44. API with NestJS #44. Implementing relationships with MongoDB
- 45. API with NestJS #45. Virtual properties with MongoDB and Mongoose
- 46. API with NestJS #46. Managing transactions with MongoDB and Mongoose
- 47. API with NestJS #47. Implementing pagination with MongoDB and Mongoose
- 48. API with NestJS #48. Definining indexes with MongoDB and Mongoose
- 49. API with NestJS #49. Updating with PUT and PATCH with MongoDB and Mongoose
- 50. API with NestJS #50. Introduction to logging with the built-in logger and TypeORM
- 51. API with NestJS #51. Health checks with Terminus and Datadog
- 52. API with NestJS #52. Generating documentation with Compodoc and JSDoc
- 53. API with NestJS #53. Implementing soft deletes with PostgreSQL and TypeORM
- 54. API with NestJS #54. Storing files inside a PostgreSQL database
- 55. API with NestJS #55. Uploading files to the server
- 56. API with NestJS #56. Authorization with roles and claims
- 57. API with NestJS #57. Composing classes with the mixin pattern
- 58. API with NestJS #58. Using ETag to implement cache and save bandwidth
- 59. API with NestJS #59. Introduction to a monorepo with Lerna and Yarn workspaces
- 60. API with NestJS #60. The OpenAPI specification and Swagger
- 61. API with NestJS #61. Dealing with circular dependencies
- 62. API with NestJS #62. Introduction to MikroORM with PostgreSQL
- 63. API with NestJS #63. Relationships with PostgreSQL and MikroORM
- 64. API with NestJS #64. Transactions with PostgreSQL and MikroORM
- 65. API with NestJS #65. Implementing soft deletes using MikroORM and filters
- 66. API with NestJS #66. Improving PostgreSQL performance with indexes using MikroORM
- 67. API with NestJS #67. Migrating to TypeORM 0.3
- 68. API with NestJS #68. Interacting with the application through REPL
- 69. API with NestJS #69. Database migrations with TypeORM
- 70. API with NestJS #70. Defining dynamic modules
- 71. API with NestJS #71. Introduction to feature flags
- 72. API with NestJS #72. Working with PostgreSQL using raw SQL queries
- 73. API with NestJS #73. One-to-one relationships with raw SQL queries
- 74. API with NestJS #74. Designing many-to-one relationships using raw SQL queries
- 75. API with NestJS #75. Many-to-many relationships using raw SQL queries
- 76. API with NestJS #76. Working with transactions using raw SQL queries
- 77. API with NestJS #77. Offset and keyset pagination with raw SQL queries
- 78. API with NestJS #78. Generating statistics using aggregate functions in raw SQL
- 79. API with NestJS #79. Implementing searching with pattern matching and raw SQL
- 80. API with NestJS #80. Updating entities with PUT and PATCH using raw SQL queries
- 81. API with NestJS #81. Soft deletes with raw SQL queries
- 82. API with NestJS #82. Introduction to indexes with raw SQL queries
- 83. API with NestJS #83. Text search with tsvector and raw SQL
- 84. API with NestJS #84. Implementing filtering using subqueries with raw SQL
- 85. API with NestJS #85. Defining constraints with raw SQL
- 86. API with NestJS #86. Logging with the built-in logger when using raw SQL
- 87. API with NestJS #87. Writing unit tests in a project with raw SQL
- 88. API with NestJS #88. Testing a project with raw SQL using integration tests
- 89. API with NestJS #89. Replacing Express with Fastify
- 90. API with NestJS #90. Using various types of SQL joins
- 91. API with NestJS #91. Dockerizing a NestJS API with Docker Compose
- 92. API with NestJS #92. Increasing the developer experience with Docker Compose
- 93. API with NestJS #93. Deploying a NestJS app with Amazon ECS and RDS
- 94. API with NestJS #94. Deploying multiple instances on AWS with a load balancer
- 95. API with NestJS #95. CI/CD with Amazon ECS and GitHub Actions
- 96. API with NestJS #96. Running unit tests with CI/CD and GitHub Actions
- 97. API with NestJS #97. Introduction to managing logs with Amazon CloudWatch
- 98. API with NestJS #98. Health checks with Terminus and Amazon ECS
- 99. API with NestJS #99. Scaling the number of application instances with Amazon ECS
- 100. API with NestJS #100. The HTTPS protocol with Route 53 and AWS Certificate Manager
- 101. API with NestJS #101. Managing sensitive data using the AWS Secrets Manager
- 102. API with NestJS #102. Writing unit tests with Prisma
- 103. API with NestJS #103. Integration tests with Prisma
- 104. API with NestJS #104. Writing transactions with Prisma
- 105. API with NestJS #105. Implementing soft deletes with Prisma and middleware
- 106. API with NestJS #106. Improving performance through indexes with Prisma
- 107. API with NestJS #107. Offset and keyset pagination with Prisma
- 108. API with NestJS #108. Date and time with Prisma and PostgreSQL
- 109. API with NestJS #109. Arrays with PostgreSQL and Prisma
- 110. API with NestJS #110. Managing JSON data with PostgreSQL and Prisma
- 111. API with NestJS #111. Constraints with PostgreSQL and Prisma
- 112. API with NestJS #112. Serializing the response with Prisma
- 113. API with NestJS #113. Logging with Prisma
- 114. API with NestJS #114. Modifying data using PUT and PATCH methods with Prisma
- 115. API with NestJS #115. Database migrations with Prisma
One of the characteristics of relational databases is a strict data structure. We need to specify the shape of every table with its fields, indexes, and relationships. Even if we design our database carefully, the requirements that our application must meet are changing. Because of that, our database needs to evolve as well. When restructuring our database, we need to be careful not to lose any existing data.
While we could manually run SQL queries to make changes to our database, it would not be straightforward to repeat across different application environments. Instead, with database migrations, we can modify our database with a set of controlled changes, such as adding tables and changing columns. Altering the structure of the database is a delicate process that can damage the existing data. With database migrations, we commit the SQL queries to the repository, where they have a chance to undergo a rigorous review before merging them into the master branch. In this article, we learn about migrations with Prisma.
Introducing Prisma migrations
In one of the previous parts of this series, we defined a simple schema of a post.
postSchema.prisma
model Post { id Int @default(autoincrement()) @id title String content String |
Whenever we create new models or adjust the existing ones, we should create a migration using the Prisma CLI.
npx prisma migrate dev --name create-post |
Running the above command generates a new file in the migrations directory.
20230702195845_create_post/migration.sql
-- CreateTable CREATE TABLE "Post" ( "id" SERIAL NOT NULL, "title" TEXT NOT NULL, "content" TEXT NOT NULL, CONSTRAINT "Post_pkey" PRIMARY KEY ("id") |
Prisma also automatically runs the above SQL query. It results in adjusting two tables in our database.
First, it creates the Post table based on our model. Then, it adds a row to the _prisma_migrations table so that Prisma can track which migrations were applied.
Adjusting migrations manually
In a previous part of this series, we modified the post model by adding the paragraphs column and removing the content column.
model Post { id Int @id @default(autoincrement()) title String paragraphs String[] |
Unfortunately, relying on a migration generated by Prisma would destroy all data in the content column. Since we want to avoid that, let’s use the --create-only flag.
npx prisma migrate dev --create-only --name add-post-paragraphs |
Doing the above generates a migration but does not run it in our database yet.
20230702212422_add_post_paragraphs/migration.sql
Warnings: - You are about to drop the column `content` on the `Post` table. All the data in the column will be lost. -- AlterTable ALTER TABLE "Post" DROP COLUMN "content", ADD COLUMN "paragraphs" TEXT[]; |
We now have a chance to modify the above migration to avoid data loss.
20230702212422_add_post_paragraphs/migration.sql
ALTER TABLE "Post" ADD COLUMN "paragraphs" TEXT[]; UPDATE "Post" SET paragraphs = ARRAY[content]; ALTER TABLE "Post" DROP COLUMN content; |
Thanks to the above approach, we:
- add the paragraphs array column first,
- copy the text from the content column and set it as the first element of each paragraphs column,
- drop the content column.
Thanks to the above approach, we restructure our table while keeping the data.
Now, we need to tell Prisma to run the migration.
npx prisma migrate deploy |
Applying migration
20230702212422_post_paragraphs
The following migration have been applied:
migrations/
└─ 20230702212422_post_paragraphs/
└─ migration.sql
When we run the above command, Prisma compares the _prisma_migrations table with the migrations we have in our project. If there is a migration that hasn’t run yet, Prisma applies it to our database.
We should make the migrate deploy command a part of our automated CI/CD pipeline so that our changes can be populated to a production database.
Dealing with the schema drift
When we run the migrate dev command, Prisma creates a temporary shadow database. It runs all our migrations there and compares the state of the shadow database with our regular development database. If they don’t match, it means there is a schema drift.
The shadow database is deleted automatically afterwards.
A schema drift might happen when we adjust the database manually instead of doing it through migration. Let’s simulate this problem by adding a new column without using a migration.
ALTER TABLE "Post" ADD COLUMN description TEXT; |
Now, let’s tell Prisma to look for the schema drift.
npx prisma migrate dev |
Drift detected: Your database schema is not in sync with your migration history.
The following is a summary of the differences between the expected database schema given your migrations files, and the actual schema of the database.
It should be understood as the set of changes to get from the expected schema to the actual schema.
[*] Changed the
Post
table
[+] Added columndescription
We need to reset the “public” schema at “localhost:5432”
Do you want to continue? All data will be lost. › (y/N)
Accepting the above would remove the changes we manually made to our database. However, there is a different solution. We can ask Prisma to update our schema based on the current state of the database.
npx prisma db pull |
When we run the above command, Prisma compares our database with our schema and makes changes to our schema.prisma file.
model Post { id Int @id @default(autoincrement()) title String paragraphs String[] description String? |
We can now create a migration based on our modified schema.
npx prisma migrate dev --name add_description_to_post |
While the above command still resets our database, it preserves our manual changes and creates a new migration that includes them.
20230702221824_add_description_to_post/migration.sql
-- AlterTable ALTER TABLE "Post" ADD COLUMN "description" TEXT; |
Breaking changes with new Prisma versions
Sometimes Prisma changes the naming conventions that they use with Prisma Migrate. A good example was switching from Prisma 2 to Prisma 3 when the approach to constraint and index names changed. The most straightforward solution for dealing with this is letting Prisma generate a migration that changes the affected constraint and indexes.
npx prisma migrate dev --name constraints-rename |
Running the above constraint can create a migration that updates the naming convention used in our project.
20230702013827_constraints_rename/migration.sql
-- DropForeignKey ALTER TABLE "Post" DROP CONSTRAINT "Post_authorId_fkey"; -- AddForeignKey ALTER TABLE "Post" ADD CONSTRAINT "Post_authorId_fkey" FOREIGN KEY ("authorId") REFERENCES "User"("id") ON DELETE RESTRICT ON UPDATE CASCADE; -- RenameIndex ALTER INDEX "User.email_unique" RENAME TO "User_email_key"; -- RenameIndex ALTER INDEX "User_addressId_unique" RENAME TO "User_addressId_key"; |
An alternative is to run the npx prisma db pull command and let Prisma modify our schema so that we can keep the old naming convention in existing constraints and avoid creating a migration.
Summary
In this article, we’ve learned the concept of database migrations. With them, we can change our databases from various environments in a controlled way. Therefore, we should use them instead of modifying the database manually.
We used Prisma Migrate to perform our migrations. Besides the most basic situations, we’ve learned how to deal with some issues, such as the need to adjust migrations manually to avoid data loss. We’ve also seen what schema drift is and how to eliminate it. Besides the above, there might be some breaking changes in various versions of Prisma Migrate, such as changing the naming convention. Fortunately, we dealt with that in a straightforward way by creating a designated migration. Learning all of the above gave as a solid understanding of what migrations are and how to work with them in a project with NestJS and Prisma.
Series Navigation<< API with NestJS #114. Modifying data using PUT and PATCH methods with PrismaRecommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK