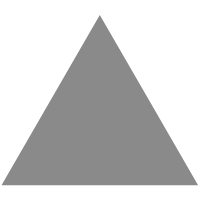
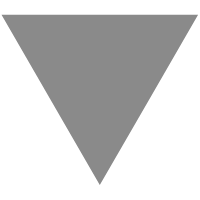
【前端方案】-表格排序列LRU缓存方案 - 辉是暖阳辉
source link: https://www.cnblogs.com/mrwh/p/17462996.html
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
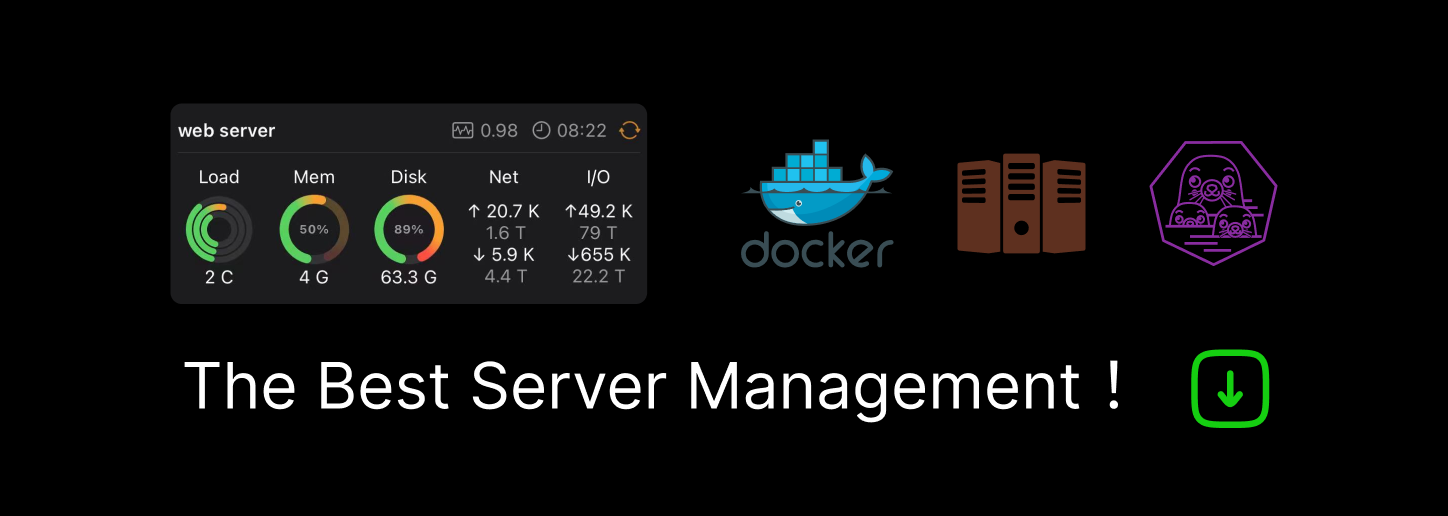
【前端方案】-表格排序列LRU缓存方案
目标:
排序后的表格列,页面刷新或者用户重新登录后,能够保持之前的操作排序
完成效果:
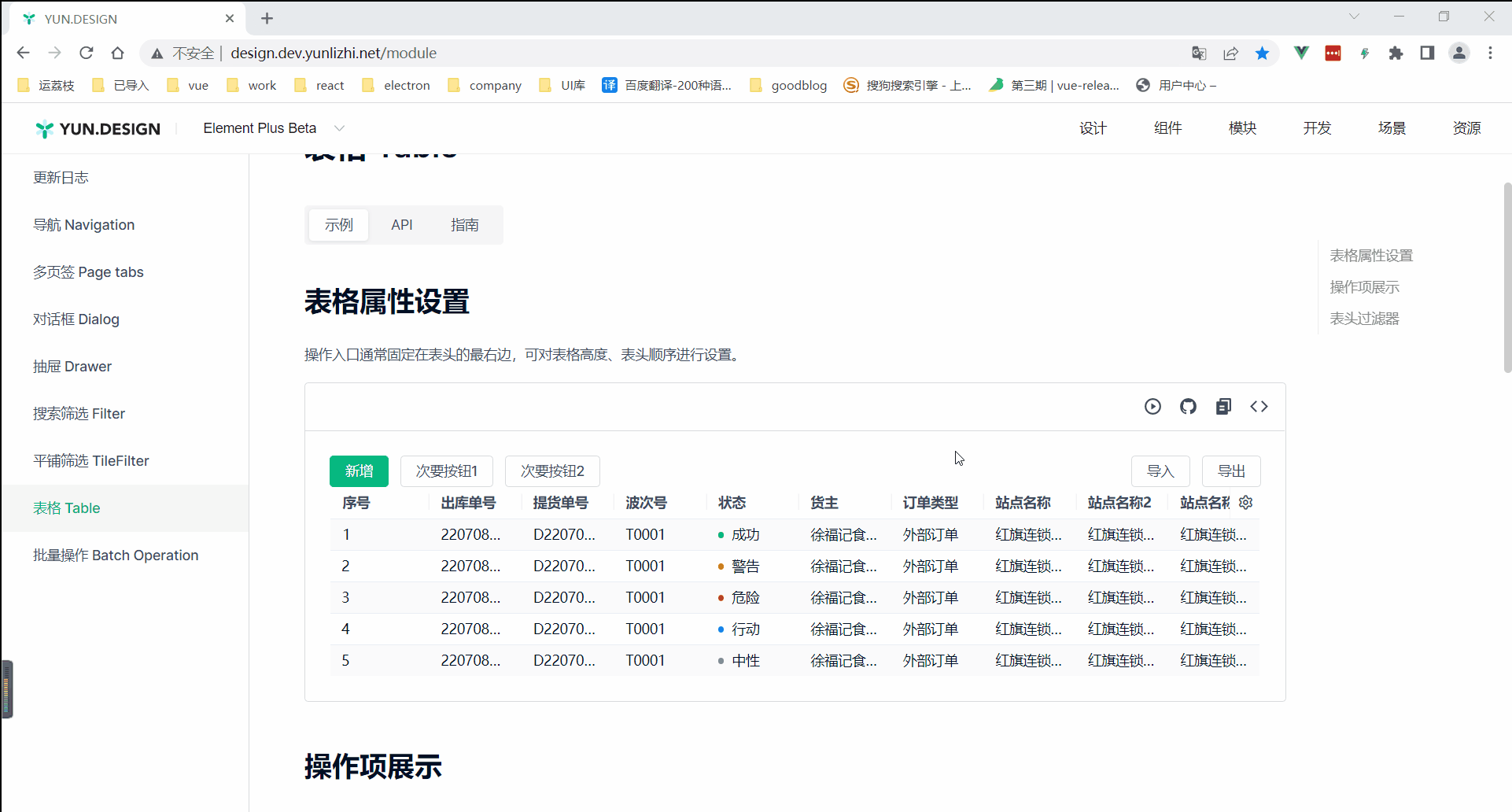
解决方案:
利用localstorage对排序后的表格列属性进行存储,页面刷新或者用户重新进入该页面时都先从localstorage中读取
1.存储方式:localstorage(key,value)
key - 表格增加配置属性tableHeaderKey,以当前路由的path+tableHeaderKey来做key
value - 排序后的属性列数组(defaultKeys-所有属性列key,selectedKeys要显示的key,tableSize表格高度)只存key,减少存储空间
2.存储时机:
配置有tableHeaderKey并且showTableSetting开启,进入页面就读取,没有则先存一份
3.移除或新增列的情况:
(1)在后续需求迭代中,以前表格的字段列可能会增加或者减少,需要将新的字段列和localstorage中的旧有字段列进行diff,如果存在删除的字段,则移除,存在新增字段则自动追加。
(2)或者强制更新,通过读取发版的版本号,如果发版的版本号大于旧的版本号,则清空localstorage。
升级方案:
针对localstorage缓存太多的情况,会采用LRU算法对缓存进行优化。
LRU全称为Least Recently Used,即最近使用的。针对的是在有限的内存空间内,只缓存最近使用的数据(即get和set的数据),超过有限内存空间的数据将会被删除。
大致思路:
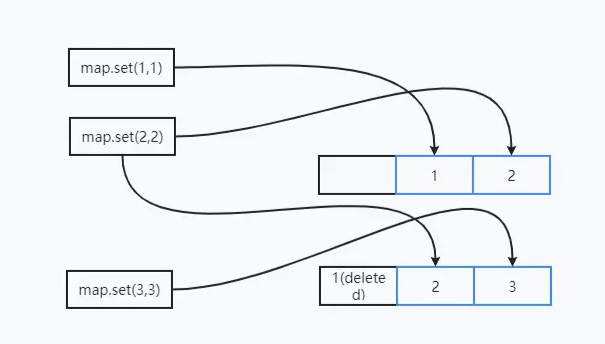
简单实现思路
class LRUCache {
data = new Map(); // 数据map
constructor(length) {
if (length < 1) throw new Error('长度不能小于1')
this.length = length
}
set(key, value) {
const data = this.data;
// 如果存在该对象,则直接删除
if (data.has(key)) {
data.delete(key);
}
// 将数据对象添加到map中
data.set(key, value);
if (data.size > this.length) {
// 如果map长度超过最大值,则取出map中的第一个元素,然后删除
const delKey = data.keys().next().value
data.delete(delKey);
}
}
get(key) {
const data = this.data;
// 数据map中没有key对应的数据,则返回null
if (!data.has(key)) return null;
const value = data.get(key);
// 返回数据前,先删除原数据,然后在添加,就可以保持在最新
data.delete(key);
data.set(key, value);
return value;
}
}
const lruCache = new LRUCache(2);
lruCache.set('1', 1); // Map(1) {1 => 1}
lruCache.set('2',2); // Map(2) {1 => 1, 2 => 2}
console.log(lruCache.get('1')); // Map(2) {2 => 2, 1 => 1}
lruCache.set('3',3); // Map(2) {1 => 1, 3 => 3}
console.log(lruCache.get('2')); // null
lruCache.set('4',4); // Map(2) {3 => 3, 4 => 4}
console.log(lruCache.get('1')); // null
console.log(lruCache.get('3')); // Map(2) {4 => 4, 3 => 3}
console.log(lruCache.get('4')); // Map(2) {3 => 3, 4 => 4}
map结合localstorage实现存储操作用例
let contacts = new Map()
contacts.set('Jessie', {phone: "213-555-1234", address: "123 N 1st Ave"})
contacts.set('Hilary22',333) // undefined
contacts.set('Hilary', {phone: "617-555-4321", address: "321 S 2nd St"})
//contacts.get('Jessie') // {phone: "213-555-1234", address: "123 N 1st Ave"}
console.log(contacts)
console.log(JSON.stringify(Array.from(contacts.entries())))
window.localStorage.setItem('test',JSON.stringify(Array.from(contacts.entries())))
//console.log(new Map(JSON.parse(window.localStorage.getItem('test'))))
contacts = new Map(JSON.parse(window.localStorage.getItem('test')))
onsole.log( contacts )
封装storage
export default {
setItem(key, item) {
let stringifyItem;
try {
stringifyItem = JSON.stringify(item);
} catch (error) {
stringifyItem = '';
} finally {
window.localStorage.setItem(key, stringifyItem);
}
},
getItem(key) {
let item;
try {
const stringifyItem = window.localStorage.getItem(key);
item = JSON.parse(stringifyItem);
} catch (erorr) {
item = null;
}
return item;
},
removeItem(key) {
window.localStorage.removeItem(key);
},
};
后续升级
达到一定量级,如果要做针对用户层面的表格列操作缓存,会考虑结合后端存储使用indexDb存储方式,支持G级别大小。
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK