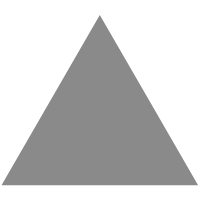
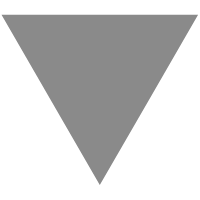
深入理解Spring Boot架构
source link: https://www.51cto.com/article/757069.html
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
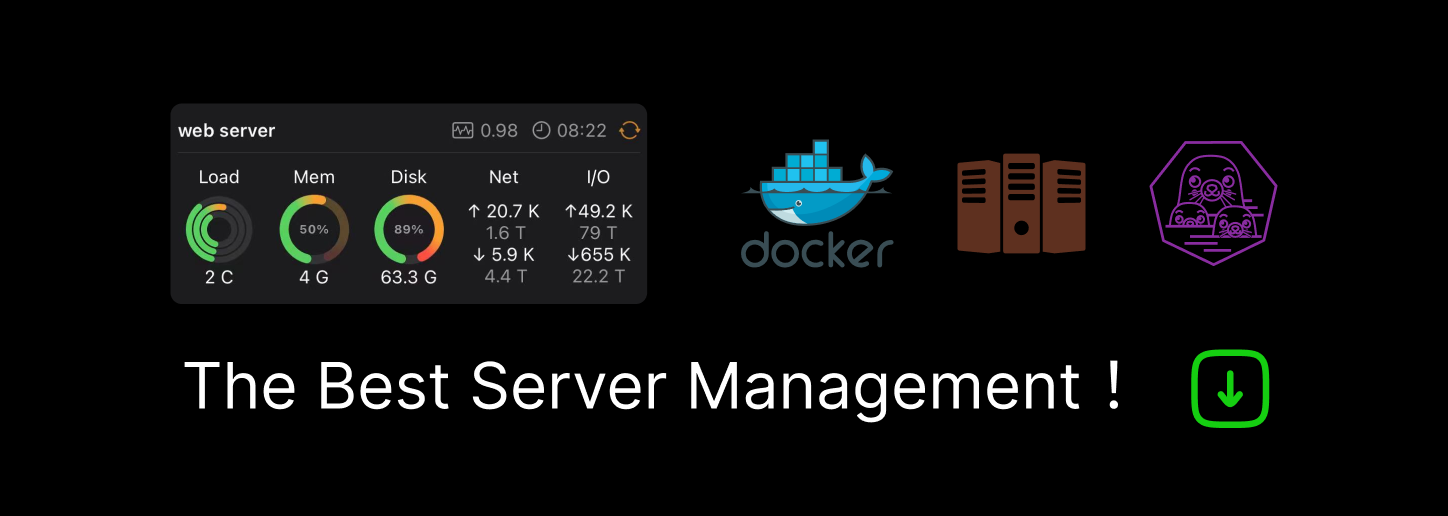
深入理解Spring Boot架构
本文的内容有助于理解Java Spring Boot框架的层次结构。
“我决定不让自己彻底崩溃,而是每个周二晚上都让自己小崩溃一下。” —— Graham Parke
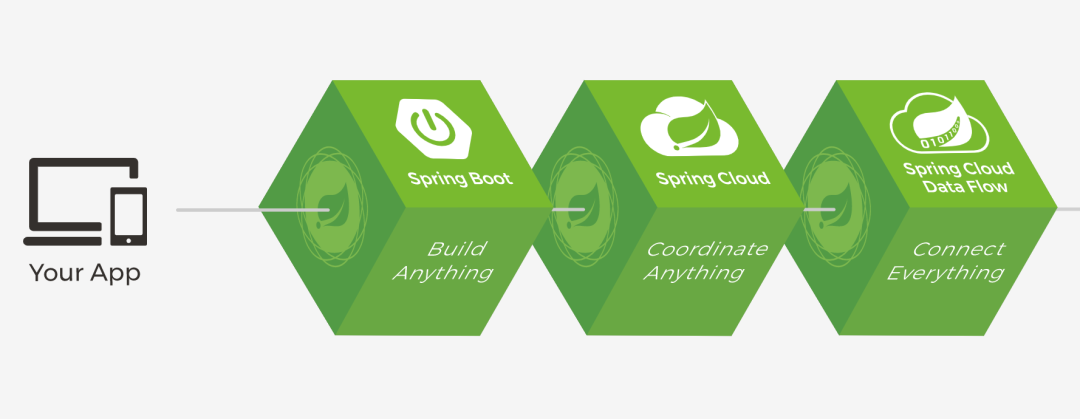
检查任何软件的最好方法是将其分成层,然后将这些层合并在一起。我们在这里遵循同样的方法。
在深入研究Java Spring Boot之前,让我们先来看一个众所周知的例子——计算机网络中的OSI模型。虽然网络整体上看起来很复杂,但我们通常将其分成层次以组织协议。我们还声明每个层都依赖于下面一层提供的服务。在Spring Boot中,同样的原则也适用。
1 Spring Boot的层次结构
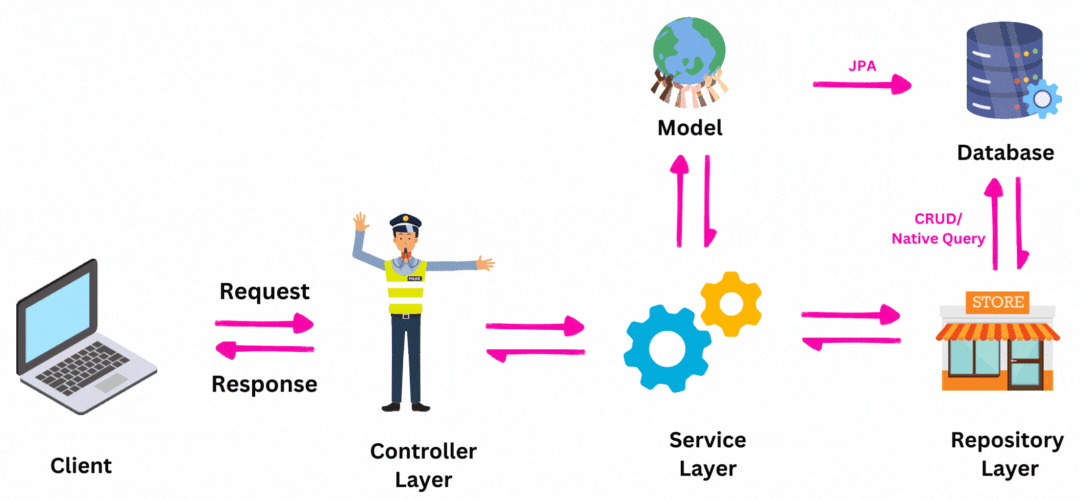
我们主要可以将Spring Boot分成四层:
1.1 控制器层
系统与客户端请求交互的第一部分是控制器。它们定义了API的端点,可以将端点想象为有效的路由和请求方法(GET、POST、PUT)。控制器的主要目标是向客户端提供服务,即提供响应、状态等。控制器利用服务层提供的服务来为客户端提供服务。
端点的示例:
- /login (POST)
- /register (POST)
- /products (GET)
1.2 服务层
服务层旨在实现业务逻辑。服务层的主要目的是向控制器层提供服务。所有对数据的计算都在这一层中执行,因此服务层需要数据。所以,它们依赖于DAO/Repository层提供的服务。
1.3 DAO/Repository层
DAO代表数据访问对象,这一层的主要目标是从数据库中高效地访问(查询)数据,并向服务层提供服务。
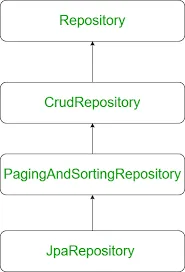
在Spring Boot中存在提供CRUD操作(创建、检索、更新、删除)的接口。因此,Repository层可以实现其中的一个。
1.4 模型层
模型表示现实世界中的对象,这些对象被称为模型。JPA(Java Persistence API)提供了关于ORM(对象关系映射)的参考或详细信息,这意味着Java类可以与数据库表相关联。在Spring Boot中存在许多JPA ORM的实现,其中之一是Hibernate。因此,您需要现实世界实体的Java类,然后将其映射到关系(表)中。
2 上述层次结构的实现模板
注意:对于实施,我们把项目管理作为一个主题。
2.1 控制器层:
ProjectController.java
package com.example.Controller;
//导入语句在此处
@RestController
public class UserController {
//列出所有可用项目
@GetMapping(path = "/projects", produces = MediaType.APPLICATION_JSON_VALUE)
public ResponseEntity<List<Project>> getProjects() {
// 执行验证检查
// 返回服务层提供的服务
}
//申请项目
@PostMapping(path = "/apply-project", consumes = MediaType.APPLICATION_JSON_VALUE)
public ResponseEntity<HttpStatus> applyProject(@RequestBody Map<String,String> json) {
// 执行验证检查
// 返回服务层提供的服务
}
//上传简历
@PostMapping(path = "/upload-resume/{usn}")
public ResponseEntity<List<Object>> uploadToDB(@RequestParam("file") MultipartFile[] file,@PathVariable String usn) {
// 执行验证检查
// 返回服务层提供的服务
}
//下载简历
@GetMapping("/files/download/{fileName:.+}")
public ResponseEntity downloadFromDB(@PathVariable String fileName) {
// 执行验证检查
// 返回服务层提供的服务
}
}
上述示例使用了@注释,这些注释用于告知spring是否是RestController,PostMapping等。
2.2 服务层:
ProjectService.java
package com.example.Service;
// 导入语句
public interface ProjectService {
ResponseEntity<List<Project>> getProjects();
HttpStatus applyProject(String USN,int project_id);
ResponseEntity<List<Object>> uploadProjectDocument(MultipartFile[] files,int project_id);
}
ProjectServiceImpl.Java
package com.example.Service;
//导入语句
@Service
public class ProjectServiceImpl implements ProjectService {
//将DAO进行依赖注入(Autowire)
@Override
public ResponseEntity<List<Project>> getProjects() {
try {
//利用DAO服务实现业务逻辑
} catch (Exception e) {
return new ResponseEntity<>(null,HttpStatus.INTERNAL_SERVER_ERROR) ;
}
}
@Override
public HttpStatus applyProject(String USN, int project_id) {
//利用DAO服务实现业务逻辑
}
//辅助函数
public ResponseEntity uploadToLocalFileSystem(MultipartFile file,int project_id) {
}
@Override
public ResponseEntity<List<Object>> uploadProjectDocument(MultipartFile[] files,int project_id) {
//利用DAO服务实现业务逻辑
}
}
2.3 Repository/DAO层:
ProjectDAO.java
package com.example.Dao;
//导入语句
public interface ProjectDao extends JpaRepository<Project,Integer> {
//你也可以在JPA提供的CRUD操作之上包含本地查询
//使用@Query注释和相应的函数在此处添加查询
@Query(value = "Your SQL query ",nativeQuery = true)
public List<Project> getProjects();
}
}
2.4 模型层:
Project.java
package com.example.Entity;
//导入语句
@Entity
@Table(name = "project")
public class Project {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private int project_id;
@Column(nullable = false, name = "company_name")
private String company_name;
@Column(nullable = false, name = "description")
private String description;
@Column(nullable = false, name = "requirements")
private String requirements;
@Column(nullable = false, name = "manager")
private String manager;
@Column(nullable = false, name = "start_date")
private Date start_date = new Date();
@Column( name = "end_date")
private Date end_date = new Date();
@Column(nullable = false,name = "opening")
private int opening;
@Column(name = "resources")
private String resources;
public Set<Staff> getStaff_incharge() {
return staff_incharge;
}
public void setStaff_incharge(Set<Staff> staff_incharge) {
this.staff_incharge = staff_incharge;
}
public Set<Student> getApplied_students() {
return applied_students;
}
public Set<Document> getDocuments() {
return documents;
}
public void setDocuments(Set<Document> documents) {
this.documents = documents;
}
@JsonIgnore
@ManyToMany(mappedBy="funded_projects")
private Set<Fund> funds;
public Set<Fund> getFunds() {
return funds;
}
public void setFunds(Set<Fund> funds) {
this.funds = funds;
}
public void setApplied_students(Set<Student> applied_students) {
this.applied_students = applied_students;
}
public Set<Student> getWorking_students() {
return working_students;
}
public void setWorking_students(Set<Student> working_students) {
this.working_students = working_students;
}
//构造函数
public Project() {
super();
}
public Project(int project_id, String company_name, String description, String requirements, String manager, Date start_date, Date end_date, int opening, String resources) {
super();
this.project_id = project_id;
this.company_name = company_name;
this.description = description;
this.requirements = requirements;
this.manager = manager;
this.start_date = start_date;
this.end_date = end_date;
this.opening = opening;
this.resources = resources;
}
public int getProject_id() {
return project_id;
}
public void setProject_id(int project_id) {
this.project_id = project_id;
}
public String getCompany_name() {
return company_name;
}
public void setCompany_name(String company_name) {
this.company_name = company_name;
}
public String getDescription() {
return description;
}
public void setDescription(String description) {
this.description = description;
}
public String getRequirements() {
return requirements;
}
public void setRequirements(String requirements) {
this.requirements = requirements;
}
public String getManager() {
return manager;
}
public void setManager(String manager) {
this.manager = manager;
}
public Date getStart_date() {
return start_date;
}
public void setStart_date(Date start_date) {
this.start_date = start_date;
}
public Date getEnd_date() {
return end_date;
}
public void setEnd_date(Date end_date) {
this.end_date = end_date;
}
public int getOpening() {
return opening;
}
public void setOpening(int opening) {
this.opening = opening;
}
public String getResources() {
return resources;
}
public void setResources(String resources) {
this.resources = resources;
}
@Override
public String toString() {
return "Project{" +
"project_id=" + project_id +
", company_name='" + company_name + '\'' +
", descriptinotallow='" + description + '\'' +
", requirements='" + requirements + '\'' +
", manager='" + manager + '\'' +
", start_date=" + start_date +
", end_date=" + end_date +
", opening=" + opening +
", resources='" + resources + '\'' +
'}';
}
}
在上面的示例中,该类表示一个表,其数据成员表示表的属性。我们还可以使用OneToOne、ManyToOne、ManyToMany注释表示表之间的关系。
上述实现是不完整的,因为本文的目的是了解工作流程和层次结构。Spring Boot非常庞大,本文只涵盖了其中的一小部分。如果本文有任何错误,在此深表歉意,希望对您有所帮助,谢谢!
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK