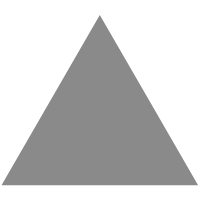
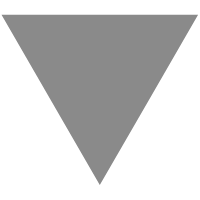
Learn With Me: Coding a Discord Chatbot in Python - Part 1
source link: https://hackernoon.com/learn-with-me-coding-a-discord-chatbot-in-python-part-1
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
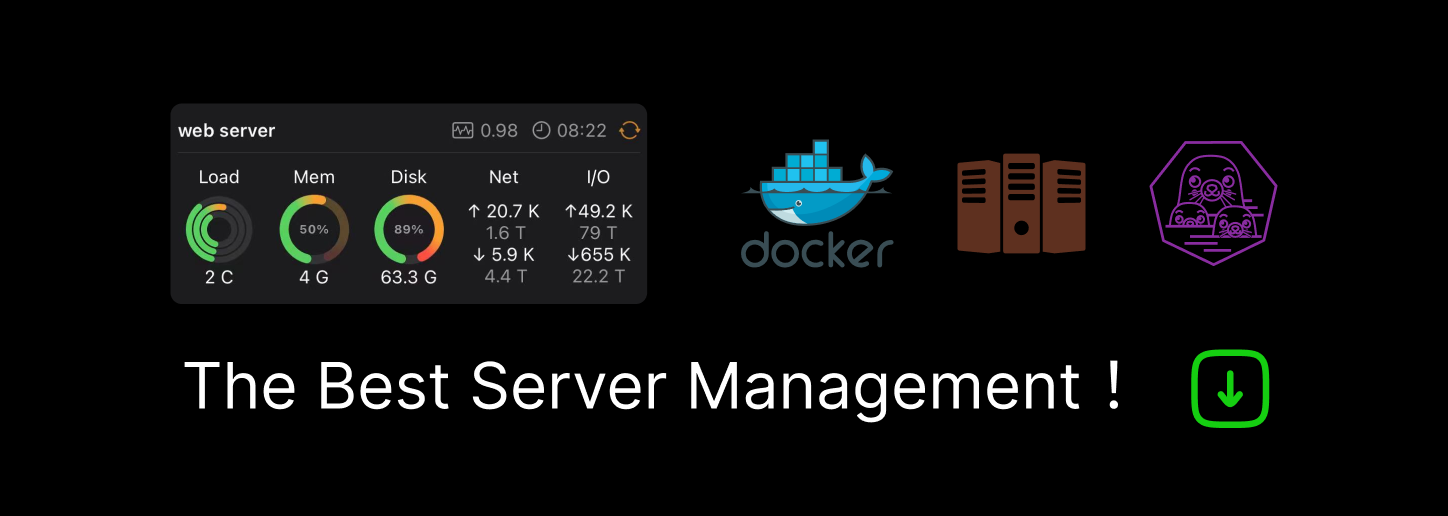
Learn With Me: Coding a Discord Chatbot in Python - Part 1
by @battlenub
Joey Charpentier
@battlenub
QA Tech Worker, previously at AMD and HP, turned blogger...
Too Long; Didn't Read
The bot’s name is currently “Norgannon”, a reference to the [Titan of Celestial Magics and Lore] in Warcraft. The bot was created using Python and pip. It is currently being tested on the Discord server of the [/r/WarcraftLore] community.
@battlenub
Joey CharpentierQA Tech Worker, previously at AMD and HP, turned blogger ...
Receive Stories from @battlenub
Credibility
In the past, I’ve struggled with not consistently practicing skills that I learn in big, sporadic bursts of energy and inspiration. So, of course, the skill levels drop back down to nearly 0 after a while, and I need to practically start again. This time, I’m trying to more consistently, yet organically, find the time and energy to create cool projects with some of the things I’ve learned, such as programming.
This is my first step in documenting my journey. Hopefully, I finish a cool project and come up with new ideas to make even more projects.
My goal is to develop a chatbot for the Discord server of the /r/WarcraftLore community. I’m a moderator of that sub and just an overall huge Warcraft lore nerd. We already have a bot to help with moderating, so this bot is going to replace some of the entertainment we enjoyed from the discontinued quiz bot.
I intend for the quiz bot to:
-
Start on command, with controls Mods of a server can be set to avoid abuse and spam.
-
Set optional limits on how often the quiz can be started hourly, daily, or weekly.
-
Set optional limits on what user roles can start a quiz.
-
Set optional limits on what channels the quiz can be started in, designated by the mod team. So for example mods could create their own quiz channel, in order to concentrate the quiz spam to just one space.
-
The bot’s name is currently “Norgannon”, a reference to the Titan of Celestial Magics and Lore in Warcraft’s lore. You can also check my progress on the github page for it: https://github.com/Battlenub89/Norgannon
I’m leveraging the capabilities of ChatGPT to assist in coding, debugging, and project planning. As an individual with ADHD, I often grapple with certain aspects that render creative projects challenging.
-
Firstly, initiating a new task is not my forte. AI tools, such as ChatGPT, are aiding me in overcoming this hurdle by providing a starting point in the form of a code block, which I can then tailor to my requirements rather than starting from scratch.
-
Secondly, planning – a seemingly insurmountable task for many with ADHD and admittedly a personal nemesis of mine. While not impossible, planning can be incredibly mentally taxing for me. Nevertheless, it’s an essential aspect if I want to employ an effective productivity and problem-solving strategy: breaking down larger tasks into smaller, more manageable ones. This approach significantly eases the perceived enormity of each step, but at times, the effort required to identify these tasks and sub-tasks proves to be too overwhelming for me.
Setting up my environment
Before starting this project, I needed to have Python and pip (Python’s package installer) ready on my machine. I also needed a few libraries: discord.py
for connecting with Discord, python-dotenv
for securely managing my bot’s token, and random
for shuffling my quiz questions.
Setting up my development environment was crucial. I created a Python virtual environment to isolate my project dependencies, ensuring that installing or updating a package for this project wouldn’t affect my other Python projects.
Discord.py ‘s example: https://discordpy.readthedocs.io/en/stable/intro.html?highlight=env#virtual-environments
Making the Bot Respond
Once my environment was set up, I began by creating the bot’s response to simple commands. This was achieved using the @bot.command()
decorator provided by [discord.py](https://discordpy.readthedocs.io/en/stable/)
.
Initially, my bot wasn’t responding to commands, but after consulting with ChatGPT, OpenAI’s language model, I realized I was missing the ‘intents’ argument while initializing my bot. Once fixed, my bot started acknowledging the ‘!hello’ command, which was a small but gratifying win.
Securing the Bot Token
Discord bots require the inclusion of a bot token within the program for identification. This token, however, could potentially be exploited by malicious actors to hijack your bot and misuse it. Despite this, I wanted to share the source code on GitHub throughout the development process. To tackle this dilemma, I discovered a solution in the form of python-dotenv.
This allows me to store the token in a .env file, which doesn’t get committed to GitHub. For added safety, I also included .env in my .gitignore file, ensuring I never inadvertently commit it. Here’s an explainer article by Monica Powell
Building the Quiz
I created a dictionary of questions and answers and used the random.choice()
function to select a random question each time. To prevent the same question from being asked twice, I converted my dictionary into a list and popped (removed) a random question off each time.
At this point, I encountered an interesting challenge: some questions could have multiple correct answers or different spellings.
To resolve this, I modified my check()
function to accept any answer from a list of correct answers. Thanks to Python’s built-in lower()
function, the bot could accept answers regardless of their capitalization. Here’s the example of this method given to me by ChatGPT:
import discord
from discord.ext import commands
import random
# Initialize bot with command prefix '!'
bot = commands.Bot(command_prefix='!')
# Quiz dictionary with questions as keys and answers as values
quiz = {
'What is the capital of France?': ['Paris'],
'Who wrote the book "1984"?': ['George Orwell', 'Orwell'],
'In what year did the first man land on the moon?': ['1969']
}
# Start quiz command
@bot.command()
async def start_quiz(ctx):
# Copy the quiz dict to a local dict
local_quiz = quiz.copy()
for _ in range(5):
if local_quiz: # Check if there are still questions left in the quiz
question, answers = random.choice(list(local_quiz.items()))
await ctx.send(question)
def check(m):
return m.content.lower() in [answer.lower() for answer in answers] and m.channel == ctx.channel
msg = await bot.wait_for('message', check=check)
await ctx.send(f'{msg.author} got it right!')
# remove the question from the local quiz dictionary
local_quiz.pop(question)
else:
await ctx.send("No more questions left!")
break
bot.run('your-token-here')
Testing the Bot
Running the bot in a Discord server, I found a bug: when I answered a quiz question correctly, the bot didn’t respond, and my terminal showed an AttributeError. ChatGPT explained that the issue was due to my attempt to call lower()
on a list object. The error was resolved by changing my check()
function to compare the user’s answer with each answer in the list, after converting all of them to lowercase.
With all these features in place, the bot was ready. It’s been a fascinating project, with plenty of learning points, and I’m grateful to ChatGPT for being my coding companion throughout. I hope sharing my experience inspires you to try creating your own Discord bot. Happy coding!
Share Your Thoughts
Do you have any experiences that you’d like to share? Your insights can be a great help to others and myself!
Also published here.
Additionally, I’m always looking for new topics to cover in my blog. If you have a question about anything mentioned here, or if there’s a specific topic you’d like me to delve into, please let me know in the comments section below via my contact form. Your question could be the inspiration for our next blog post!
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK